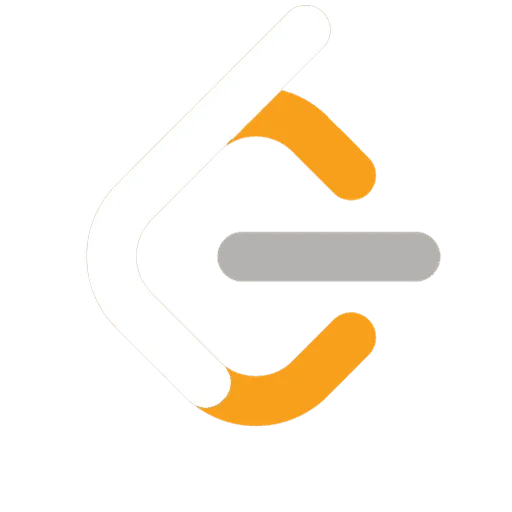
😎풀이
- 직렬화는
bfs
를 활용하여 root
로 전달받은 트리를 하나의 배열로 변경한다.
- 역직렬화는 전달받은 문자열을 배열로 변환하여
bfs
를 활용해 다시 이진 트리로 변환한다.
function serialize(root: TreeNode | null): string {
if(!root) return ''
let result = []
const queue = [[root]]
while(queue.length) {
const curDepth = queue.shift()
const nextDepth = []
for(let i = 0; i < curDepth.length; i++) {
const curNode = curDepth[i]
if(curNode === null) {
result.push(null)
continue
}
result.push(curNode.val)
nextDepth.push(curNode.left)
nextDepth.push(curNode.right)
}
if(nextDepth.length) queue.push(nextDepth)
}
return JSON.stringify(result)
};
function deserialize(data: string): TreeNode | null {
if(!data) return null
const nodeArr = JSON.parse(data);
const root = new TreeNode(nodeArr[0])
const queue = [root]
let idx = 1
while(queue.length) {
const parent = queue.shift()
if(idx < nodeArr.length && nodeArr[idx] !== null) {
parent.left = new TreeNode(nodeArr[idx])
queue.push(parent.left)
}
idx++
if(idx < nodeArr.length && nodeArr[idx] !== null) {
parent.right = new TreeNode(nodeArr[idx])
queue.push(parent.right)
}
idx++
}
return root
}