1. AJAX
1-1. JSON
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script>
var person = {
"employees": [
{
"name": "Surim",
"lastName": "Son"
},
{
"name": "Someone",
"lastName": "Huh"
},
{
"name": "Someone else",
"lastName": "Kim"
}
]
}
</script>
- key와 value가 있으며, key는 쌍따옴표 안에 넣어준다. ("key")
console.log(person.employees[0].name);
console.log(person.employees[1].lastName);
1-2. AJAX 기초
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.3/jquery.min.js"></script>
<script>
$(function () {
$.ajax({
type: "get",
url: "https://mdn.github.io/learning-area/javascript/oojs/json/superheroes.json",
success: function (data) {
console.log(data);
}
});
});
</script>
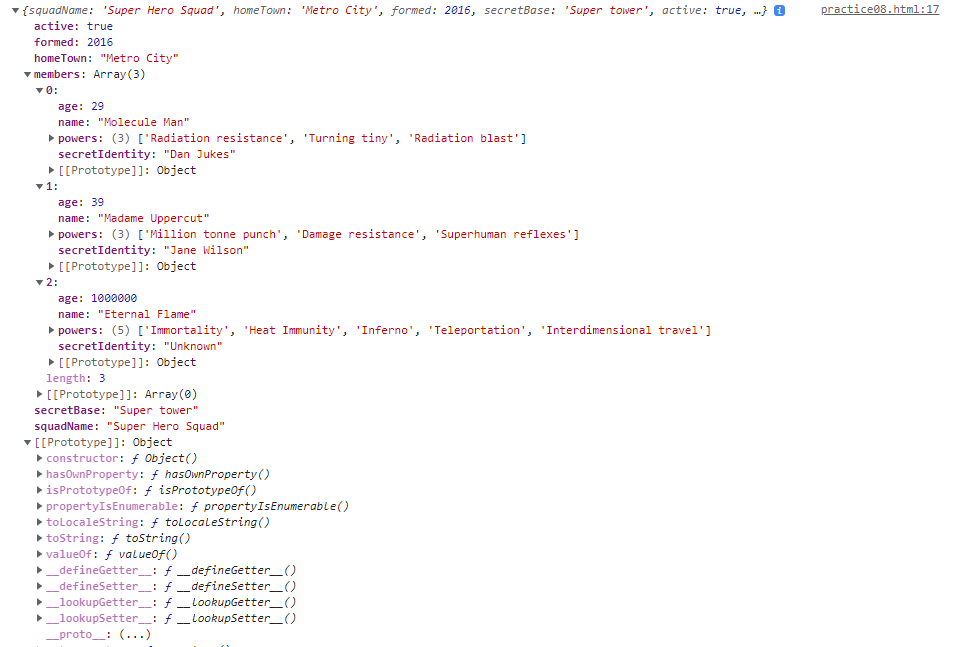
console.log(data);
console.log(data.squadName);
$(data.members).each(function (index, member) {
console.log(member);
});
2. 예제
2-1. 슈퍼 히어로
<!DOCTYPE html>
<html>
<head>
<title>Superheroes Table</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function () {
$.ajax({
url: "https://mdn.github.io/learning-area/javascript/oojs/json/superheroes.json",
dataType: "json",
success: function (data) {
console.log(data);
$.each(data.members, function (index, superhero) {
var row = "<tr>" +
"<td>" + superhero.name + "</td>" +
"<td>" + superhero.age + "</td>" +
"<td>" + superhero.secretIdentity + "</td>" +
"<td>" + superhero.powers + "</td>" +
"</tr>";
$("#superheroesTable tbody").append(row);
});
}
});
});
</script>
<style>
table,
th,
td {
border: 1px solid black;
border-collapse: collapse;
padding: 5px;
}
</style>
</head>
<body>
<table id="superheroesTable">
<thead>
<tr>
<th>Name</th>
<th>Age</th>
<th>Secret Identity</th>
<th>Powers</th>
</tr>
</thead>
<tbody></tbody>
</table>
</body>
</html>

2-2. 인적사항 테이블
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: "GET",
url: "http://sample.bmaster.kro.kr/contacts?pageno=3&pagesize=10",
success: function (result) {
console.log(result);
var htmls = "";
$("#list-table").html("");
$("<tr>", {
html: "<td>" + "번호" + "</td>" +
"<td>" + "이름" + "</td>" +
"<td>" + "전화번호" + "</td>" +
"<td>" + "주소" + "</td>" +
"<td>" + "사진" + "</td>" +
"<td>" + "삭제" + "</td>"
}).appendTo("#list-table")
$(result.contacts).each(function () {
htmls += '<tr>';
htmls += '<td>' + this.no + '</td>';
htmls += '<td>' + this.name + '</td>';
htmls += '<td>' + this.tel + '</td>';
htmls += '<td>' + this.address + '</td>';
htmls += '<td>' + '<img src=' + this.photo + ' />' + '</td>';
htmls += '<td>' + "<input type='button' class='del-button' id=" + this.no + ' value="삭제" />' + '</td>';
htmls += '</tr>';
});
$("#list-table").append(htmls);
},
error: function (e) {
console.log(e);
}
});
});
</script>
<script>
$(document).on("click", ".del-button", function () {
console.log(this);
$(this).parent().parent().remove();
});
</script>
</head>
<body>
<table id="list-table" width="500" border="1">
</table>
</body>
</html>