이쁘니까 ,,,,ㅎㅎ
일상과 공부 내용을 정리를 위한 플랫폼으로서, 벨로그가 가장 보기에 편하고
코드블럭을 생성할 수 있기 때문에 선택하게 되었다.
#include<iostream>
#include<string>
using namespace std;
class arrStack {
public:
int t;
int* array;
int capacity;
arrStack(int capacity) {
this->t = -1;
this->array = new int[capacity];
this->capacity = capacity;
}
int empty() {
if (t == -1)// if stack is empty
return 1;
else
return 0;
}
void push(int elem) {
if (t == capacity - 1)//if stack is full
{
cout << -1 << endl;
}
else
{
t = t + 1;//t 하나 증가
array[t] = elem;
}
}
int top() {
if (t == -1)//스택이 비었으면
return -1;
else
return array[t];
}
void pop() {
if (empty())//스택이 비었으면
cout << -1 << endl;
else
{
cout << array[t] << endl;//삭제할 element 출력
t = t - 1;//top 하나 줄이기
}
}
int size() {
return t + 1;
}
};
int main() {
int N;
cin >> N;
arrStack stack(10000);
for (int i = 0; i < N; i++) {
string menu;
cin >> menu;
if (menu == "top") {
cout << stack.top() << endl;
}
else if (menu == "empty") {
cout << stack.empty() << endl;
}
else if (menu == "push") {
int elem;
cin >> elem;
stack.push(elem);
}
else if (menu == "pop") {
stack.pop();
}
else if (menu == "size") {
cout << stack.size() << endl;
}
}
}
#include<iostream>
#include<string>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int e) {
this->data = e;
this->next = NULL;
}
};
class Stack {
public:
Node* top;
int size;
Stack() {
top = NULL;
size = 0;
}
int empty() {
if (size == 0)
return 1;
else
return 0;
}
int Top() {
if (size == 0)
{
return -1;
}
else
{
return top->data;
}
}
void push(int X) {
Node* v = new Node(X);
if (size == 0)
{
top = v;
size++;
}
else
{
v->next = top;
top = v;
size++;
}
}
int pop() {
if (size == 0)
return -1;
else
{
Node* temp = top;
top = temp->next;
size--;
return temp->data;
delete temp;
}
}
int Size()
{
return size;
}
};
int main() {
int N;
cin >> N;
Stack s;
for (int i = 0; i < N; i++) {
string menu;
cin >> menu;
if (menu == "empty")
cout << s.empty() << endl;
else if (menu == "top")
cout << s.Top() << endl;
else if (menu == "push")
{
int x;
cin >> x;
s.push(x);
}
else if (menu == "pop")
cout << s.pop() << endl;
else if (menu == "size")
cout << s.Size() << endl;
}
}
후위표기식 연산 예시
* 378+-2*
->3(7+8)-2*
->(3-15)*2
->-12*2=-24
#include<iostream>
#include<string>
using namespace std;
class Node {
public:
int data;
Node* next;
Node(int e) {
this->data = e;
this->next = NULL;
}
};
class Stack {
public:
Node* top;
int size;
Stack() {
top = NULL;
size = 0;
}
int empty() {
if (size == 0) {
return 1;
}
else
return 0;
}
void push(int X) {
Node* v = new Node(X);
if (size == 0) {
top = v;
size++;
}
else {
v->next = top;
top = v;
size++;
}
}
int pop() {
if (size == 0)
{
return -1;
}
else {
Node* temp = top;
top = temp->next;
size--;
return temp->data;
delete temp;
}
}
int getTop() {
if (size == 0) {
return -1;
}
else {
return top->data;
}
}
int calculate(string inputString) {
int n = inputString.size();
Stack S;
char charOne;
int result = 0;
int num1;
int num2;
for (int i = 0; i < n; i++) {
charOne = inputString[i];
if (charOne == '+') {
num1 = S.pop();
num2 = S.pop();
result = num1 + num2;
S.push(result);
}
else if (charOne == '-') {
num1 = S.pop();
num2 = S.pop();
result = num2 - num1;
S.push(result);
}
else if (charOne == '*') {
num1 = S.pop();
num2 = S.pop();
result = num1 * num2;
S.push(result);
}
else {
int num = charOne - '0';
S.push(num);
}
}
return S.getTop();
}
};
int main() {
Stack myStack;
int N;
cin >> N;
for (int i = 0; i < N; i++) {
string inp;
cin >> inp;
cout << myStack.calculate(inp) << '\n';
}
}
오늘은 html을 복습해 보았다.
creating web page
<strong>creating web page</strong>
<u>web</u>
<h1>HTML</h1>
new
<br>line
p and br tag do the same thing.
But br tag is just for make a new line and p tag refers to make a paragraph.
so if you want to make a paragraph, p tag is better than bt tag.
paragraph tag
<p>paragraph1</p><p>paragraph2</p>
<li>1. HTML</li>
<li>2. CSS</li>
<li>3. JavsScript</li>
<ul>
<li>1. HTML</li>
<li>2. CSS</li>
<li>3. JavsScript</li>
</ul>
<ul>
<li>name1</li>
<li>name2</li>
<li>name3</li>
<h1>HTML</h1>
</ul>
- web
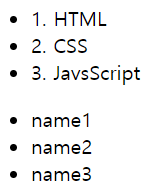
#### ordered list
- code
```c
<ol>
<li>HTML</li>
<li>CSS</li>
<li>/JavsScript</li>
</ol>
<title>WEB1 - html</title>
- web

#### utf-8 로 문서를 읽기
: utf-8로 작성된 코드(문서)는 utf 8 로 읽어야 한다.
- code
```c
<meta charset="utf-8">
:본문은 body tag로 묶어야 한다.
:본문(body) 를 설명하는 태그들은 head 태그로 묶어야 한다.
: head, body tag을 감싸는 태그.
: html 태그 위에, 아래의 문서는 html으로 작성된 것임을 나타내기 위해 쓰는 태그.
<a href="https://www.w3.org/TR/2011/WD-html5-20110405/">Hypertext Markup Language (HTML)</a>
- web

#### 링크 클릭햇을 때 새 탭으로 열기
- code
```c
<a href="https://www.w3.org/TR/2011/WD-html5-20110405/" target="_blank">Hypertext Markup Language (HTML)</a>
<a href="https://www.w3.org/TR/2011/WD-html5-20110405/" target="_blank" title="html5 specification">Hypertext Markup Language (HTML)</a>
https://opentutorials.org/course/3084/18891
: 유튜브 퍼가기에서 코드 복사 후 삽입
code
web
analytics.google.com
header: 웹 문서 맨 윗부분에 있으며 웹 사이트 이름, 글로벌 링크(로그인, 회원가입, 사이트맵, 언어 선택등 웹사이트 어느 곳에서든지 이용할 수 있는 링크)등으로 구성된 영역이다.
사이트 이름(로고), 내비게이션, 헤드라인, 검색 등으로 구성된다.
nav: 목적지로 이동할 수 있도록 링크를 별도로 모아둔 영역이다.
section: 제목, 본문 등 섹션을 표시한다.
article: 코멘트
footer: 저작권, 연락처 등