스택(Stack)
- 한 쪽 끝에서만 자료를 넣고 뺄 수 있는 LIFO(Last In First Out) 형식의 자료구조
- 한 줄로 눕혀진 탑을 생각하면 이해가 편하다.
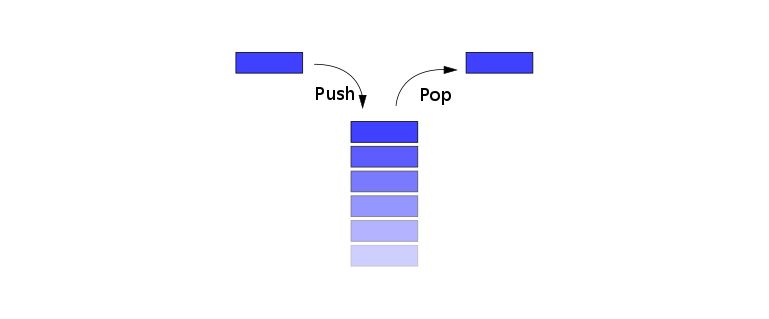
스택(Stack)의 연산
- pop : 스택의 마지막 요소를 제거한다.
- push : 스택의 가장 윗부분에 요소를 추가한다.
- top : 스택의 가장 위에 있는 항목을 반환한다. 스택이 비어있다면 -1을 반환한다.
- isEmpty : 스택이 비어 있다면 true를 반환한다.
스택(Stack)의 구현
class Stack {
constructor() {
this.arr = [];
}
size() {
return this.arr.length;
}
push(item) {
this.arr.push(item);
}
pop() {
if (this.arr.length <= 0) return null;
const result = this.arr.pop();
return result;
}
top() {
if (this.arr.length <= 0) return -1;
return this.arr[this.arr.length - 1];
}
isEmpty() {
if (!this.arr.length) {
return true;
}
return false;
}
}
const myStack = new Stack();
myStack.push(1);
myStack.push(2);
myStack.push(3);
console.log(myStack);
myStack.pop();
console.log(myStack);
console.log(myStack.size());
myStack.pop();
console.log(myStack.top());
배열 기본 메서드를 사용하지 않은 구현
class Stack {
constructor() {
this.arr = [];
this.index = 0;
}
size() {
return this.index;
}
push(item) {
this.arr[this.index++] = item;
}
pop() {
if (this.index <= 0) return null;
const result = this.arr[--this.index];
return result;
}
top() {
if (this.arr.length <= 0) return -1;
return this.arr[this.arr.length - 1];
}
isEmpty() {
if (!this.arr.length) {
return true;
}
return false;
}
}