큐(Queue)
- 스택과 달리 먼저 들어간데이터가먼저 나오는 선입선출 자료구조 FIFO(First In First Out)
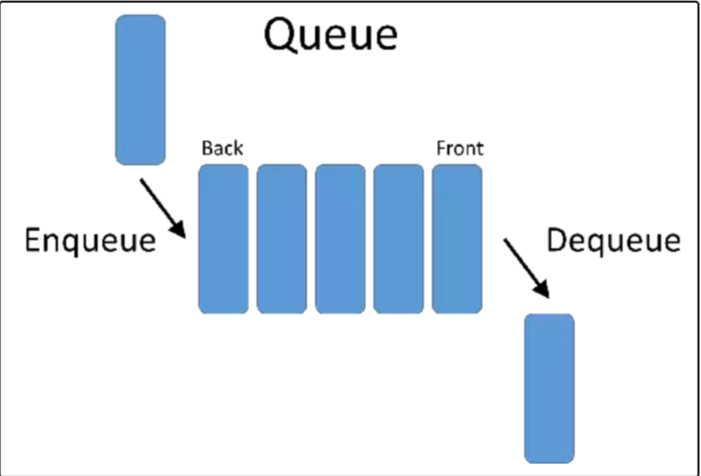
큐(Queue)의 연산
- enqueue (add) : 큐의 끝 부분에 요소를 추가한다.
- dequeue : 큐의 첫번째 요소를 제거한다.
- front : 큐의 가장 앞 요소를 출력한다.
- back : 큐의 가장 뒤 요소를 출력한다.
큐(Queue)의 구현
class Queue {
constructor() {
this.arr = [];
}
size() {
return this.arr.length;
}
enqueue(item) {
this.arr.push(item);
}
dequeue() {
if (!this.arr.length) {
return -1;
} else {
const result = this.arr.shift();
return result;
}
}
front() {
if (!this.arr.length) {
return -1;
} else {
return this.arr[0];
}
}
back() {
if (!this.arr.length) {
return -1;
} else {
return this.arr[this.arr.length - 1];
}
}
isEmpty() {
if (!this.arr.length) {
return 1;
} else {
return 0;
}
}
}
배열 메서드 없이 구현
class Queue {
constructor() {
this.arr = [];
this.head = 0;
this.tail = 0;
}
enqueue(item) {
this.arr[this.tail++] = item;
}
dequeue() {
if (this.head >= this.tail) {
return null;
} else {
const result = this.arr[this.head++];
return result;
}
}
}
class Node {
constructor(x, y, wall, time) {
this.x = x;
this.y = y;
this.wall = wall;
this.time = time;
this.next = null;
}
}
class Queue {
constructor() {
this.head = null;
this.tail = null;
this.size = 0;
}
push(x, y, wall, time) {
let node = new Node(x, y, wall, time);
if (this.size === 0) {
this.head = node;
} else {
this.tail.next = node;
}
this.tail = node;
this.size++;
}
shift() {
let temp = this.head;
if (this.size === 0) {
this.head = null;
this.tail = null;
} else {
this.head = this.head.next;
}
this.size--;
return temp;
}
length() {
return this.size;
}
}
``