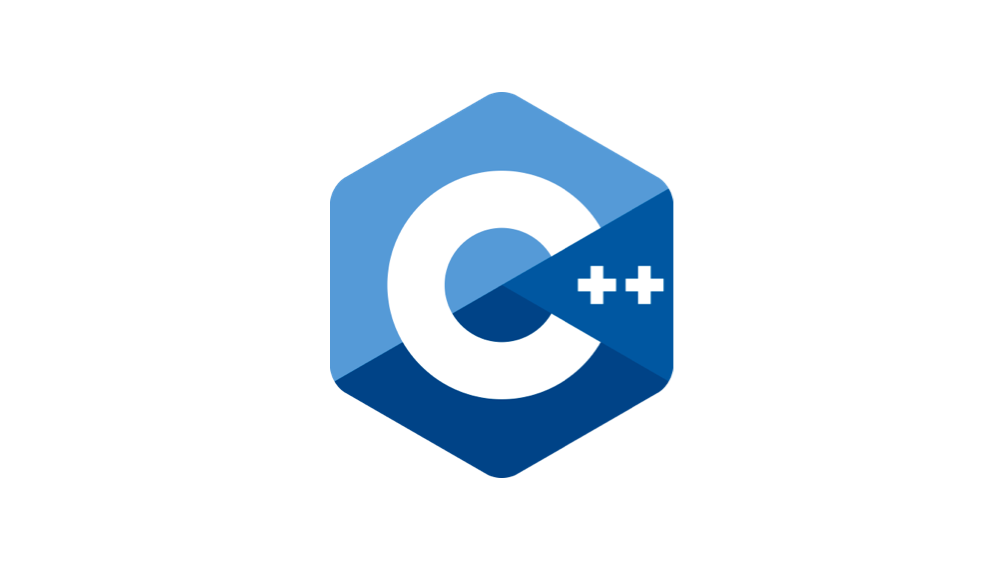
cpp란
- C++ is a general-purpose programming language created by Bjarne Stroustrup as an extension of the C programming language, or "C with Classes" (source: Wikipedia).
CPP Module 주의사항
- -std=c++98 플래그 사용. c++98 문법으로 작성해야함.
- class이름은 UpperCamelCase format으로 작성. 클래스를 담고 있는 파일은 항상 클래스명과 동일해야 함.
- C++11 또는 Boost library 사용 불가.
- using namespace 금지.
- 08 제외 STL 사용 금지. Containers나 Algorithms 사용 금지.
ex00
<iostream>
- <stdio.h> 와 비슷하게 생각하면 됨. cpp의 입출력 관련 헤더.
namespace
ex01
- Contact 클래스와 Phonebook 클래스를 만들어 전화번호부 프로그램을 만든다.
- Contact는 기본적으로 first name, last name, nickname, phone number, darkest secret에 대한 정보를 저장할 수 있어야 한다.
- 그리고 Phonebook 클래스에서는 contact클래스 배열을 선언하여 가질 수 있게 한다. (최대 8개)
ex02
- 로그 파일과 똑같이 출력되도록 Account.hpp와 test.cpp를 보고 Account.cpp에 필요한 함수를 정의하면 된다.
- 로그 파일이라고 해서 뭐 별다른 함수를 사용해야 하는 게 아니라 그냥 test.cpp에 보면 display관련 함수들에서 로그파일에 관련된 내용을 출력해야 하는 것을 유추할 수 있다. 그 양식에 맞게 함수를 짜주면 된다.
_displayTimestamp(void)
[19920104_091532]
생성자 Account( int initial_deposit )
[19920104_091532] index:0;amount:42;created
[19920104_091532] index:1;amount:54;created
[19920104_091532] index:2;amount:957;created
[19920104_091532] index:3;amount:432;created
[19920104_091532] index:4;amount:1234;created
[19920104_091532] index:5;amount:0;created
[19920104_091532] index:6;amount:754;created
[19920104_091532] index:7;amount:16576;created
- _displayTimestamp 사용해서 타임스탬프 찍어주고 각 값 출력해주면 됨.
displayAccountsInfos(void)
[19920104_091532] accounts:8;total:20049;deposits:0;withdrawals:0
- private static 변수 출력하기 위해 getter를 사용해(getNbAccounts, getTotalAmount, getNbDeposits, getNbWithdrawals) 각 값 출력
displayStatus( void ) const
[19920104_091532] index:0;amount:42;deposits:0;withdrawals:0
[19920104_091532] index:1;amount:54;deposits:0;withdrawals:0
[19920104_091532] index:2;amount:957;deposits:0;withdrawals:0
[19920104_091532] index:3;amount:432;deposits:0;withdrawals:0
[19920104_091532] index:4;amount:1234;deposits:0;withdrawals:0
[19920104_091532] index:5;amount:0;deposits:0;withdrawals:0
[19920104_091532] index:6;amount:754;deposits:0;withdrawals:0
[19920104_091532] index:7;amount:16576;deposits:0;withdrawals:0
- 양식에 맞게 _accountIndex, _amount, _nbDeposits, _nbWithdrawals 출력 해주면 됨
makeDeposit( int deposit )
[19920104_091532] index:0;p_amount:42;deposit:5;amount:47;nb_deposits:1
[19920104_091532] index:1;p_amount:54;deposit:765;amount:819;nb_deposits:1
[19920104_091532] index:2;p_amount:957;deposit:564;amount:1521;nb_deposits:1
[19920104_091532] index:3;p_amount:432;deposit:2;amount:434;nb_deposits:1
[19920104_091532] index:4;p_amount:1234;deposit:87;amount:1321;nb_deposits:1
[19920104_091532] index:5;p_amount:0;deposit:23;amount:23;nb_deposits:1
[19920104_091532] index:6;p_amount:754;deposit:9;amount:763;nb_deposits:1
[19920104_091532] index:7;p_amount:16576;deposit:20;amount:16596;nb_deposits:1
- 인자로 받은 입금액을 멤버변수(_amount)와 static 변수(_totalAmount)에 더해주면 됨.
- 양식에 맞게 입금하기 전 p_amount(_amount), 입금액 deposit, 입금 후 금액 amount(_amount + deposit), 입금 횟수 nb_deposits(_nbDeposits) 출력하면 됨
makeWithdrawal( int withdrawal )
[19920104_091532] index:0;p_amount:47;withdrawal:refused
[19920104_091532] index:1;p_amount:819;withdrawal:34;amount:785;nb_withdrawals:1
[19920104_091532] index:2;p_amount:1521;withdrawal:657;amount:864;nb_withdrawals:1
[19920104_091532] index:3;p_amount:434;withdrawal:4;amount:430;nb_withdrawals:1
[19920104_091532] index:4;p_amount:1321;withdrawal:76;amount:1245;nb_withdrawals:1
[19920104_091532] index:5;p_amount:23;withdrawal:refused
[19920104_091532] index:6;p_amount:763;withdrawal:657;amount:106;nb_withdrawals:1
[19920104_091532] index:7;p_amount:16596;withdrawal:7654;amount:8942;nb_withdrawals:1
- 인자로 받은 출금액을 멤버변수(_amount)와 static 변수(_totalAmount)에서 빼주면 됨.
- 입금과 달리 출금할 금액이 amount보다 크면 refused 돼야 한다.
checkAmount(void) const
- return (_amount > 0) 으로 정의해주고 출금 조건에서 사용해 주었음 (if (checkAmount() && _amount >= withdrawal))
소멸자 ~Account( void )
[19920104_091532] index:0;amount:47;closed
[19920104_091532] index:1;amount:785;closed
[19920104_091532] index:2;amount:864;closed
[19920104_091532] index:3;amount:430;closed
[19920104_091532] index:4;amount:1245;closed
[19920104_091532] index:5;amount:23;closed
[19920104_091532] index:6;amount:106;closed
[19920104_091532] index:7;amount:8942;closed
- 똑같이 양식에 맞게 변수값들 출력해주고 closed 출력해주면 됨.