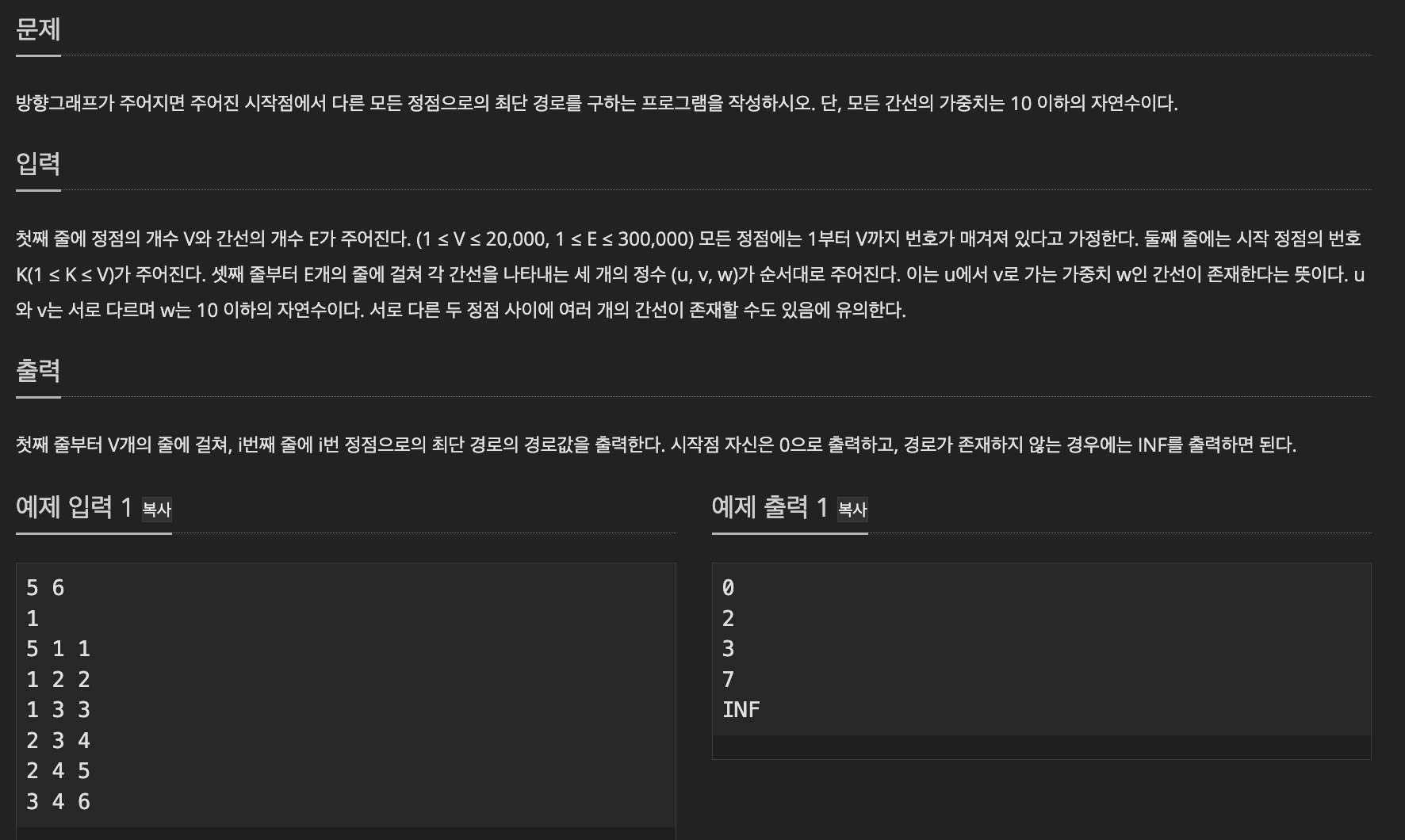
✅ 동작과정
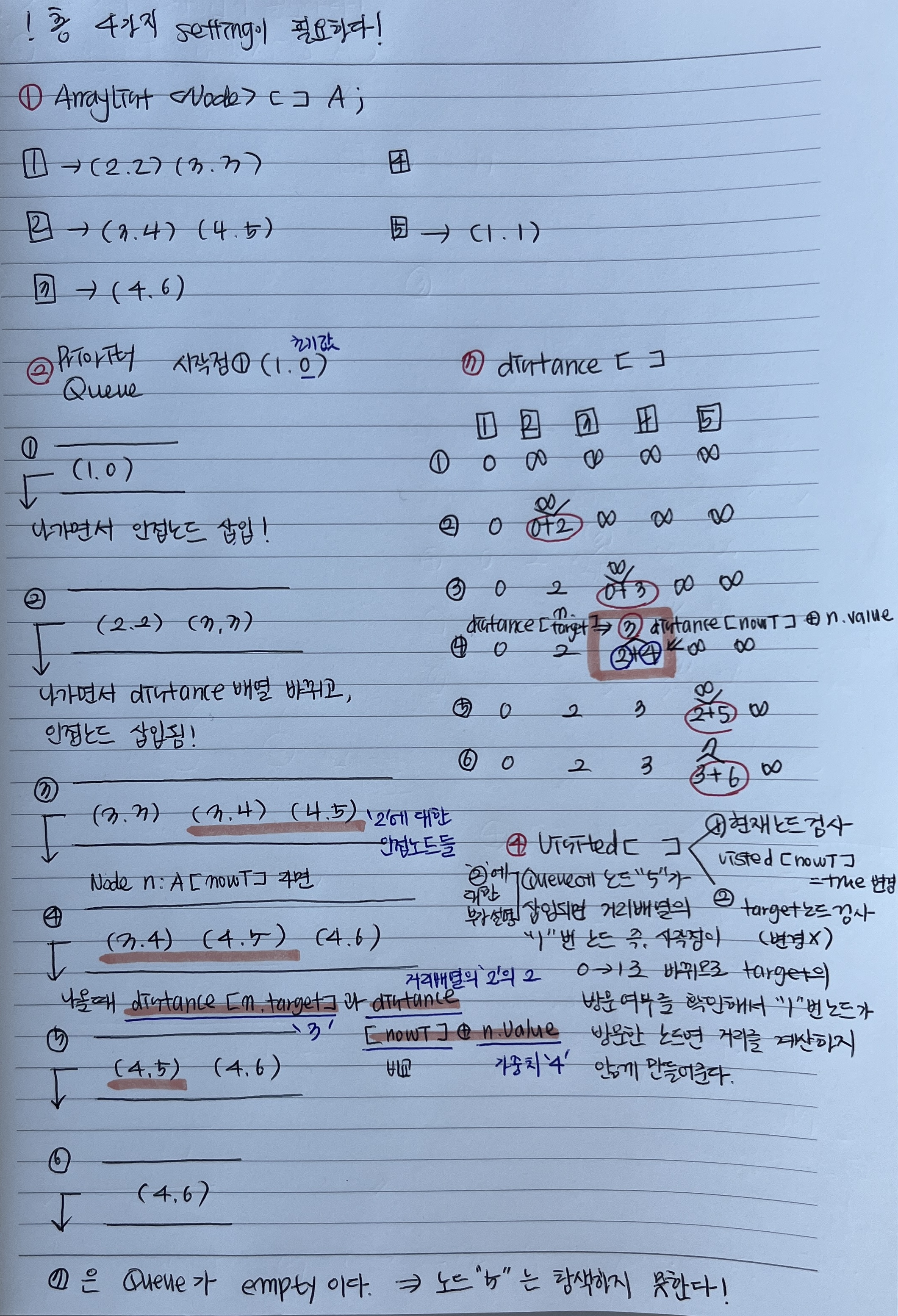
✅ 코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.PriorityQueue;
import java.util.StringTokenizer;
class Node implements Comparable<Node> {
int target;
int value;
public Node(int target, int value) {
this.target = target;
this.value = value;
}
public int compareTo(Node w) {
return value - w.value;
}
}
public class Main {
static ArrayList<Node>[] A;
static int[] distance;
static boolean[] visited;
static PriorityQueue<Node> Q = new PriorityQueue<>();
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st;
st = new StringTokenizer(br.readLine());
int V = Integer.parseInt(st.nextToken());
int E = Integer.parseInt(st.nextToken());
int K = Integer.parseInt(br.readLine());
A = new ArrayList[V + 1];
distance = new int[V + 1];
visited = new boolean[V + 1];
Arrays.fill(distance, Integer.MAX_VALUE);
distance[K] = 0;
for (int i = 1; i <= V; i++) {
A[i] = new ArrayList<>();
}
for (int i = 0; i < E; i++) {
st = new StringTokenizer(br.readLine());
int s = Integer.parseInt(st.nextToken());
int e = Integer.parseInt(st.nextToken());
int w = Integer.parseInt(st.nextToken());
A[s].add(new Node(e, w));
}
Q.add(new Node(K, 0));
while (!Q.isEmpty()) {
Node now = Q.poll();
int nowT = now.target;
if (!visited[nowT]) {
visited[nowT] = true;
for (Node n : A[nowT]) {
if (!visited[n.target] && distance[n.target] > distance[nowT] + n.value) {
distance[n.target] = distance[nowT] + n.value;
Q.add(new Node(n.target, distance[n.target]));
}
}
}
}
for (int i = 1; i <= V; i++) {
if (visited[i]) {
System.out.println(distance[i]);
} else {
System.out.println("INF");
}
}
}
}
