가상환경 만들기(conda)
# 가상환경 새로 만들기 ("가상환경이름" - 변경 해야하는 부분)
conda create -n 가상환경이름 python=3.9
# 현재 사용 할 가상환경 설정하기
conda activate 가상환경이름
# 패키지 설치 (ex.matplotlib, jupyter)
conda install numpy
conda install matplotlib
conda install jupyter
# 현재 디렉토리 탐색, 디렉토리 이동
ls
cd next
# 현재 디렉토리에서 jupyter 실행
jupyter notebook
# 가상환경 사용 중지
conda deactivate
Exception Handling
try ~ except
- 예외 발생 시 런타임 종료되지 않고 예외처리 후 계속 수행
try :
예외 발생 가능 코드
except 처리할예외:
print("error")
for i in range(10):
try:
print(10 / i)
except ZeroDivisionError:
print("Not divided by 0")
for i in range(10):
try:
print(10 / i)
print(a[i])
print(v)
except ZeroDivisionError:
print("Not divided by 0")
except IndexError as e:
print(e)
except Exception as e:
print(e)
try~except~finally
- finall : 예외 발생 여부와 상관없이 항상 실행될 코드
try:
for i in range(1, 10):
result = 10 // i
print(result)
except ZeroDivisionError:
print("Not divided by 0")
finally:
print("종료되었습니다.")
built-in exception
raise
- 강제로 Exception을 발생시켜서 코드 수행 종료
while True:
value = input("변환할 정수 값을 입력해주세요")
for digit in value:
if digit not in "0123456789":
raise ValueError("숫자값을 입력하지 않으셨습니다")
print("정수값으로 변환된 숫자 -", int(value))
assert
- 구문이 False를 반환하면 error발생시켜서 코드 수행 종료
def get_binary_nmubmer(decimal_number):
assert isinstance(decimal_number, int)
return bin(decimal_number)
print(get_binary_nmubmer(10))
파일의 종류
Binary 파일
- binary 형식으로 저장된 파일
- 특정 어플리케이션에서만 열 수 있다(종속)
- 메모장으로 열면 깨져서 알 수 없는 내용이 출력
- 엑셀, 워드, 한글 파일 등
Text 파일
- 문자열 형식으로 저장된 파일
- 메모장으로 열면 내용 확인 가능
- txt, HTML, 파이썬 코드 파일 등
- 실제로는 바이너리 형태로 저장된다.
: ASCII 코드 형태
: ex. A -> 65(2)로 저장
File I/O
open, close
- 파일 처리위한 키워드
- 처리할 파일의 주소 지정
f = open("파일이름", "접근모드")
f.close()
- close()
- 열려있는 파일 객체 닫아줌
- 프로그램 종료 때 일괄로 닫히긴한다.
- 쓰기모드로 열었던 파일 닫지 않고 다시 사용하려고 하면 오류 발생
-> 직접 닫아주는 것이 좋다.
read
f = open("i_have_a_dream.txt", "r" )
contents = f.read()
print(contents)
f.close()
with open("i_have_a_dream.txt", "r") as my_file:
contents = my_file.read()
print (type(contents), contents)
- readlines()
: 한 줄씩 읽어 list 타입으로 반환
with open("i_have_a_dream.txt", "r") as my_file:
content_list = my_file.readlines()
print(type(content_list))
print(content_list)
- readline()
한 번에 한 줄씩만 가져오기
with open("i_have_a_dream.txt", "r") as my_file:
i = 0
while True:
line = my_file.readline()
if not line:
break
print (str(i) + " === " + line.replace("\n", ""))
i = i + 1
write
f = open("count_log.txt", 'w', encoding="utf8")
for i in range(1, 11):
data = "%d번째 줄입니다.\n" % i
f.write(data)
f.close()
f = open("count_log.txt", 'ㅁ', encoding="utf8")
for i in range(1, 11):
data = "%d번째 줄입니다.\n" % i
f.write(data)
f.close()
Directory
os 모듈
import os
os.mkdir("log")
if not os.path.isdir("log"):
os.mkdir("log")
try:
os.mkdir("abc")
except FileExistError as e:
print("Already created")
pathlib 모듈
- path를 객체로 다룬다.
- os별로 다른 path 표현을 통일해서 관리할 수 있다.
>>> import pathlib
>>>
>>> cwd = pathlib.Path.cwd()
>>> cwd
WindowsPath('D:/workspace')
>>> cwd.parent
WindowsPath('D:/')
>>> list(cwd.parents)
[WindowsPath('D:/')]
>>> list(cwd.glob("*"))
[WindowsPath('D:/workspace/ai-pnpp'), WindowsPath('D:/workspace/cs50_auto_grader'),
WindowsPath('D:/workspace/data-academy'), WindowsPath('D:/workspace/DSME-AI-SmartYard'), WindowsPath('D:/workspace/introduction_to_python_TEAMLAB_MOOC'),
pickle
- 수행동안 메모리에 존재하는 객체 데이터를 저장
: 영속화
- python에 특화된 binary 파일
log
logging
- log
- 프로그램이 실행되는 동안의 일어나는 정보
- 유저의 접근, 프로그램 Exception, 특장 함수 사용 등
- Console 화면에 출력 (print), 파일에 write, DB에 남기기
- 실행시점에 남겨야 하는 기록
개발시점에 남겨야 하는 기록
logging 모듈
import logging
logging.debug("틀렸잖아!")
logging.info("확인해")
logging.warning("조심해!")
logging.error("에러났어!!!")
logging.critical ("망했다...")
logging level
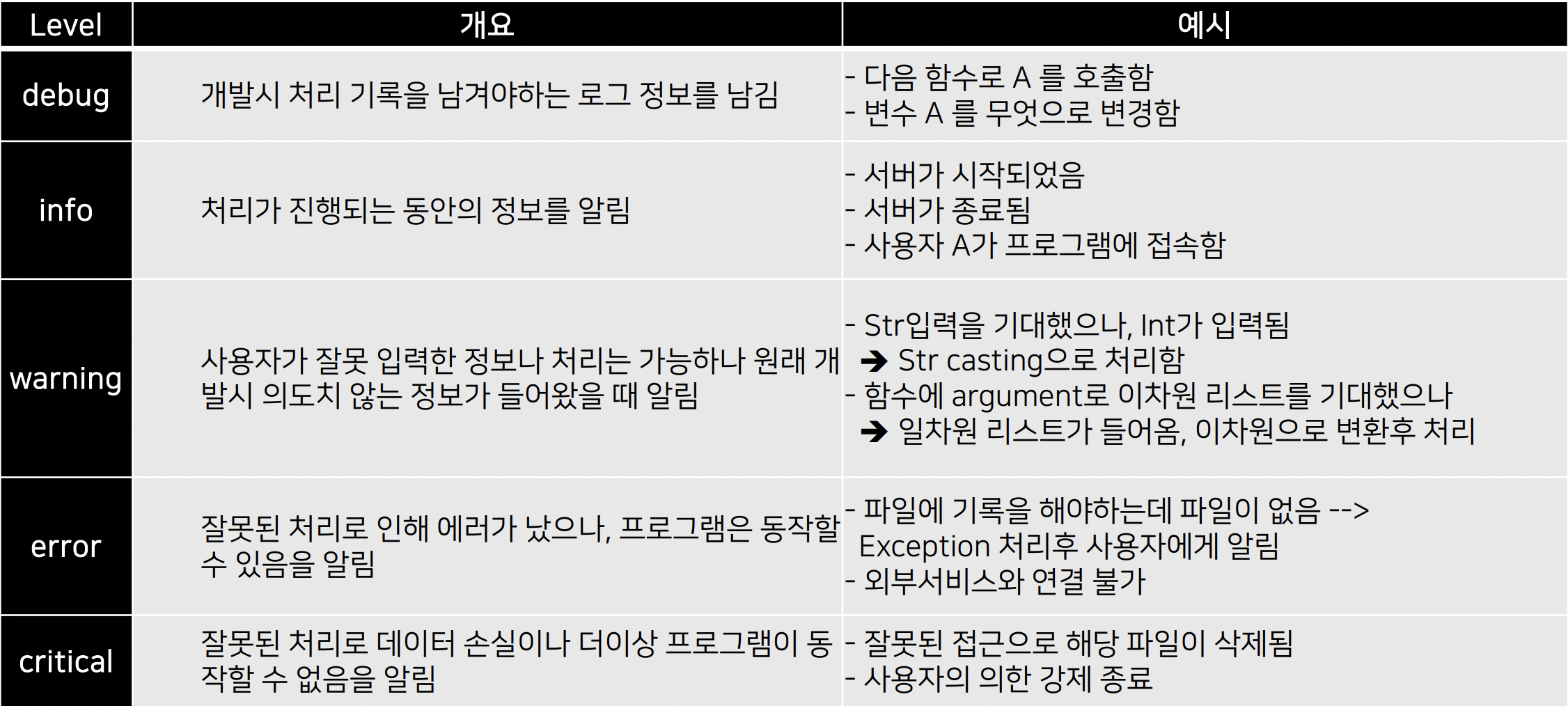
- python logging level 기본 세팅
: warning 부터
-> warning 이전 로그 출력 안 된다.
- warning level 세팅
logging.basicConfig(level=logging.DEBUG)
logger = logging.getLogger("main")
logger.setLevel(logging.INFO)
사전설정
- configparser
- 파일에
- Section, Key, Value 값 in 설정 파일
- 설정파일 Dict Type으로 호출
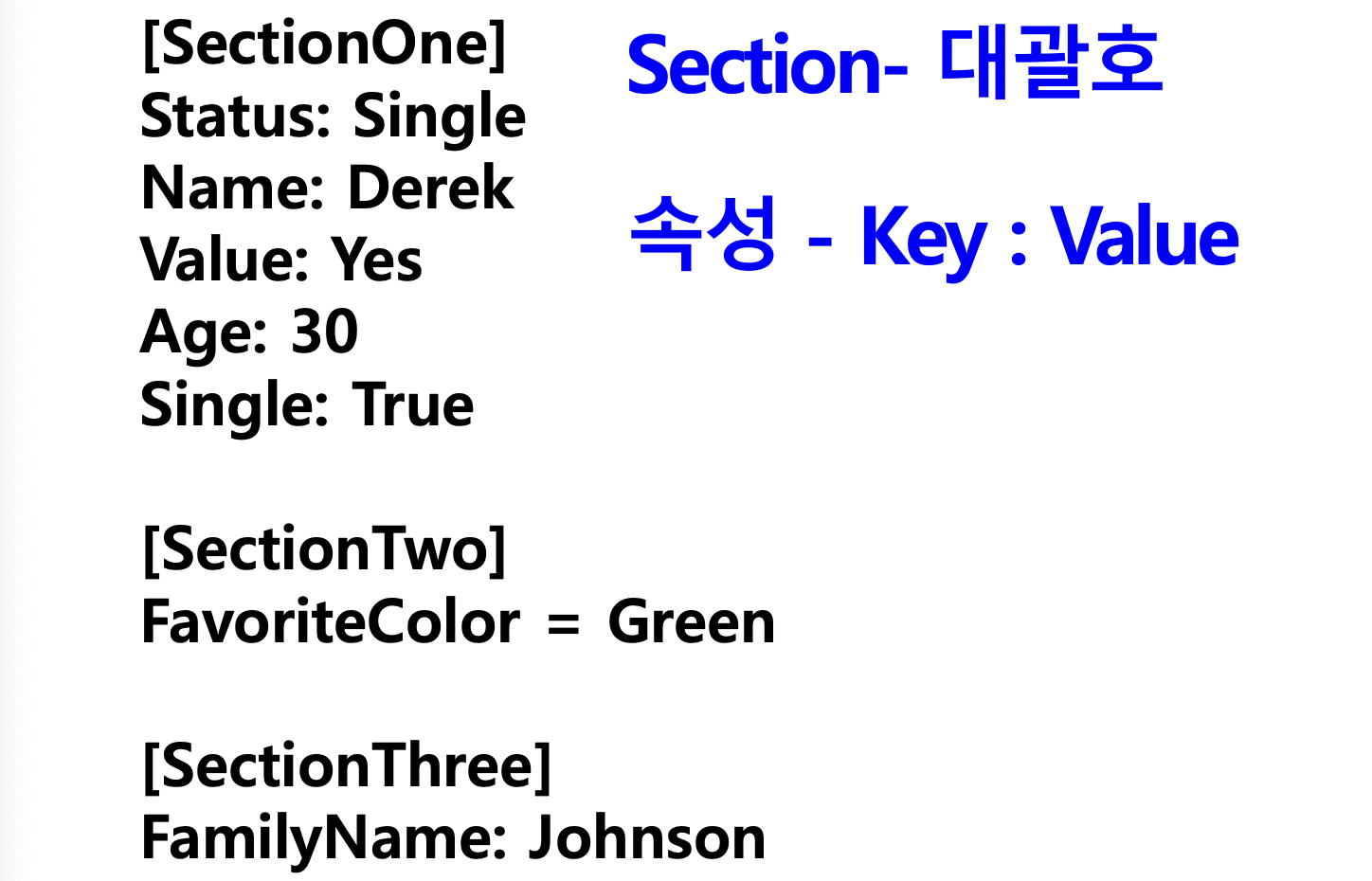
import configparser
config = configparser.ConfigParser()
config.sections()
config.read('example.cfg')
config.sections()
for key in config['SectionOne']:
value = config['sectionTwo'][key]
print(f"{key} : {value}")
config['SectionOne']["status"]
- argperser
- Console 창에서 프로그램 실행 설정 저장
- Command-Line Option 이라고 부름
ls --help
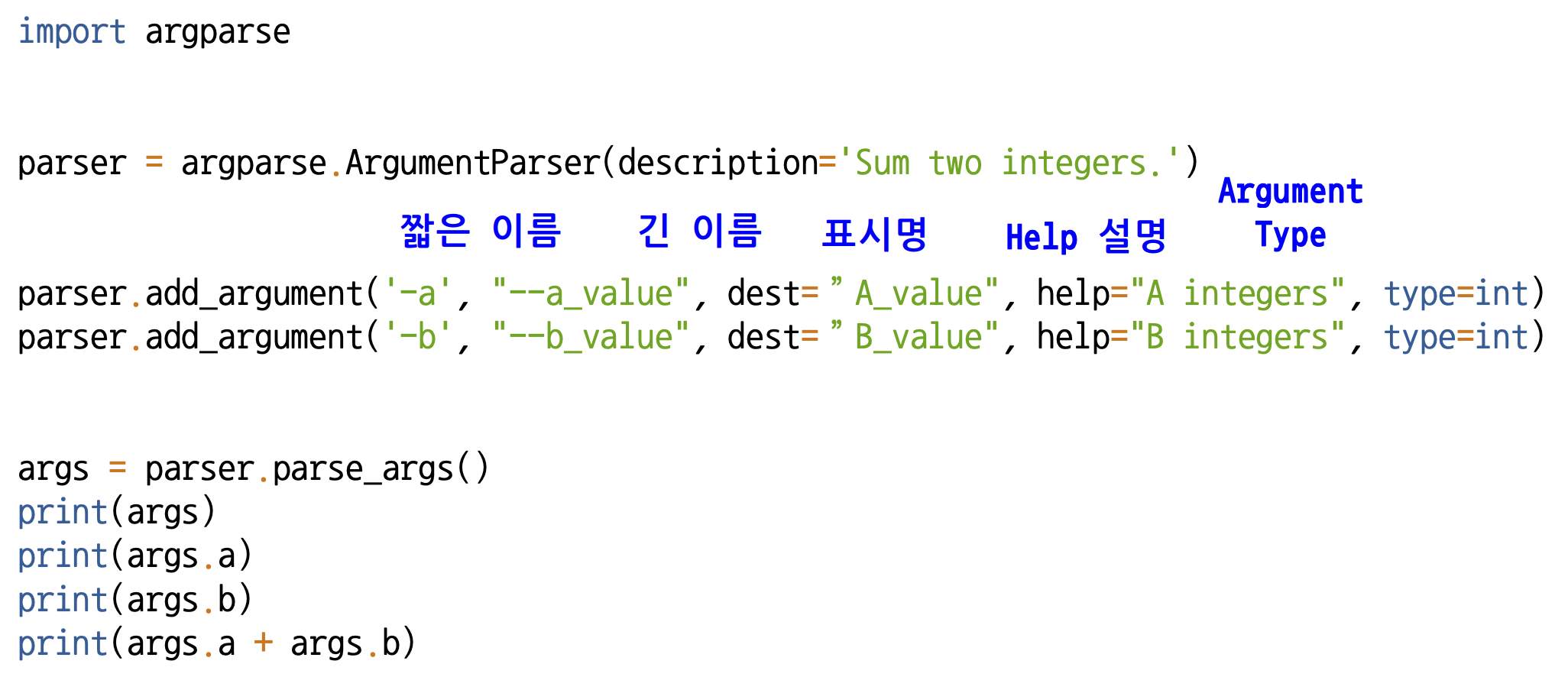