onPressed
- Column 내부에 RaisedButton을 지정해줍니다.
- onPressed를 이용해서 click을 사용할 수 있습니다.
- child를 이용해서 Text를 지정할 수 있습니다.
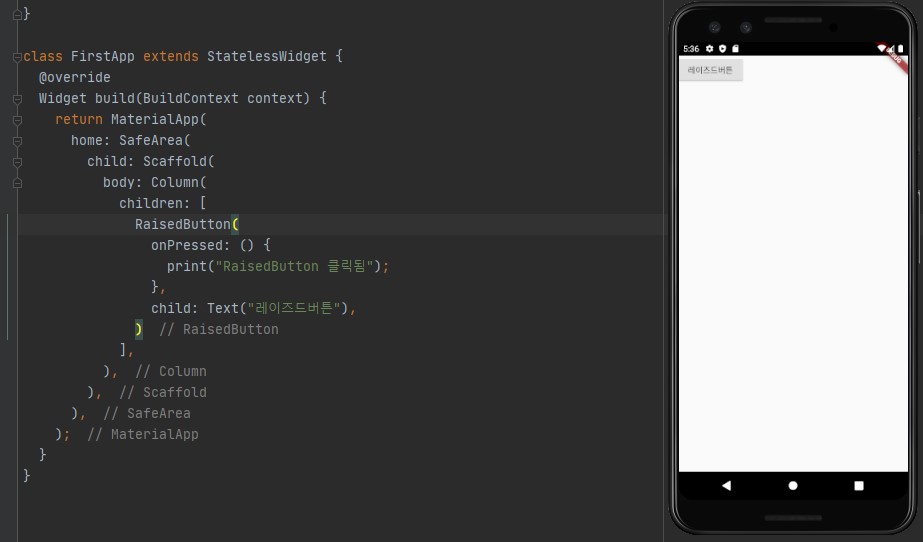
class FirstApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SafeArea(
child: Scaffold(
body: Column(
children: [
RaisedButton(
onPressed: () {
print("RaisedButton 클릭됨");
},
child: Text("레이즈드버튼"),
)
],
),
),
),
);
}
}
elevation
- 버튼의 그림자 효과를 줘서 높이감을 나타낼 수 있으며, 값에 따라 높이감을 더 많이 줄 수 있습니다.
borderRadius
- shape를 이용해서 버튼의 모서리를 둥글게 바꿔줄 수 있습니다.
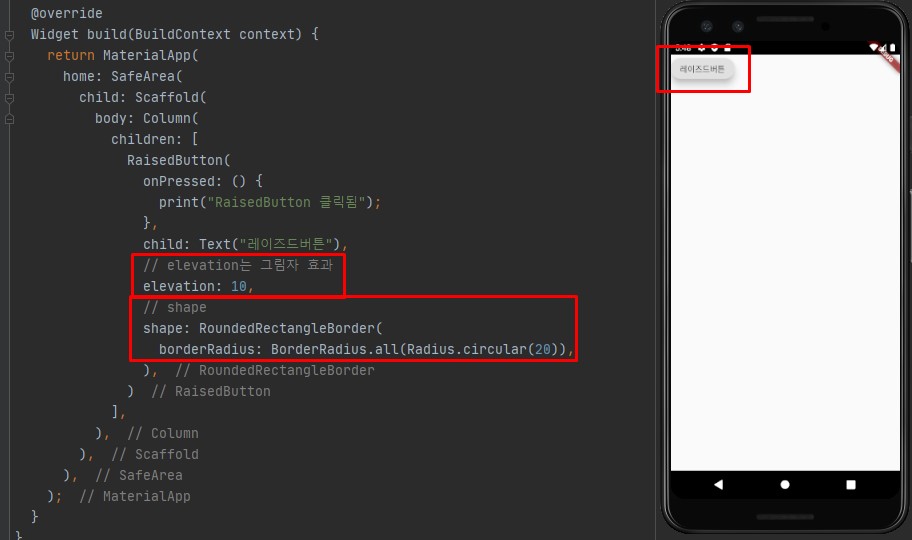
...
RaisedButton(
onPressed: () {
print("RaisedButton 클릭됨");
},
child: Text("레이즈드버튼"),
// elevation는 그림자 효과
elevation: 10,
// shape
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(27.5),
),
)
...
padding
- 다른 언어에서와 마찬가지로 버튼 내부에 여백을 지정할 수 있습니다.
- 테두리선이 없는 버튼처럼 생기지 않은 버튼입니다.
- FlatButton은 직접 커스터마이징을 좀 해줘야 합니다.
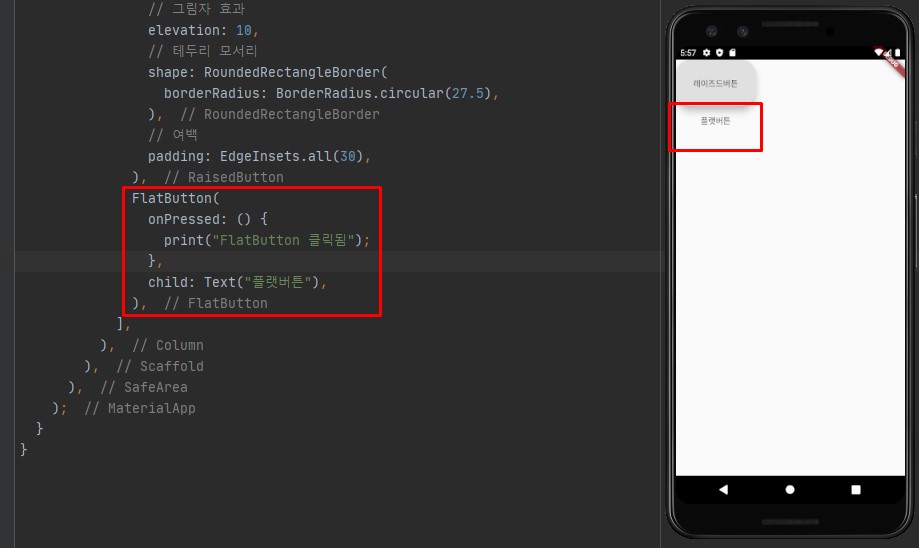
...
FlatButton(
onPressed: () {
print("FlatButton 클릭됨");
},
child: Text("플랫버튼"),
),
...
- RaisedButton이 FlatButton보다 기본 elevation이 더 높아 조금 더 버튼같아 보입니다.
- 여러 종류의 버튼을 동시에 사용할 필요가 없으므로 RaisedButton을 사용하는 것을 권장합니다.
커스텀 버튼
- 직접 버튼을 만들기 위해서 Container를 하나 지정해주고 색상과 문자를 설정해줍니다.
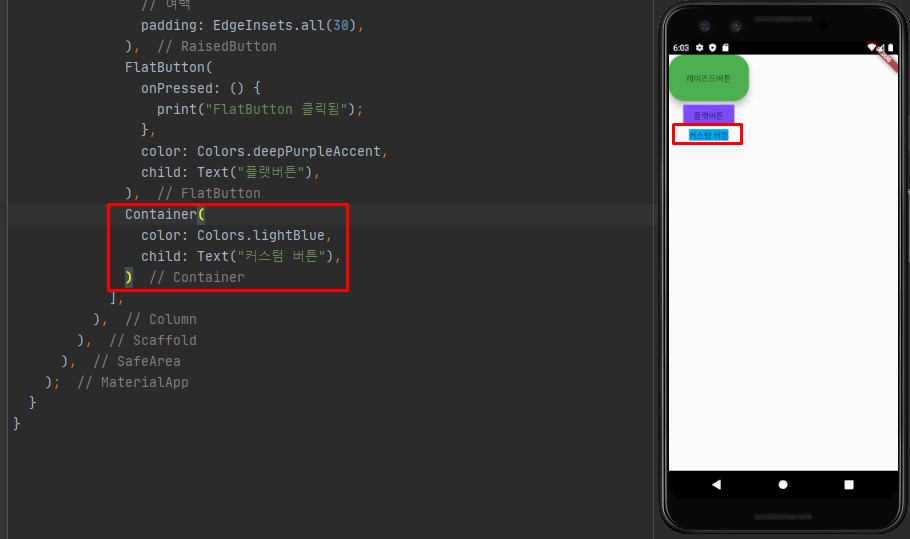
...
Container(
color: Colors.lightBlue,
child: Text("커스텀 버튼"),
)
...
- Container를 Widget으로 감싸 InkWell로 바꿔줍니다.
- InkWell로 감싸서 onTap을 적용시켜 주면 버튼처럼 작동을 할 수 있습니다.
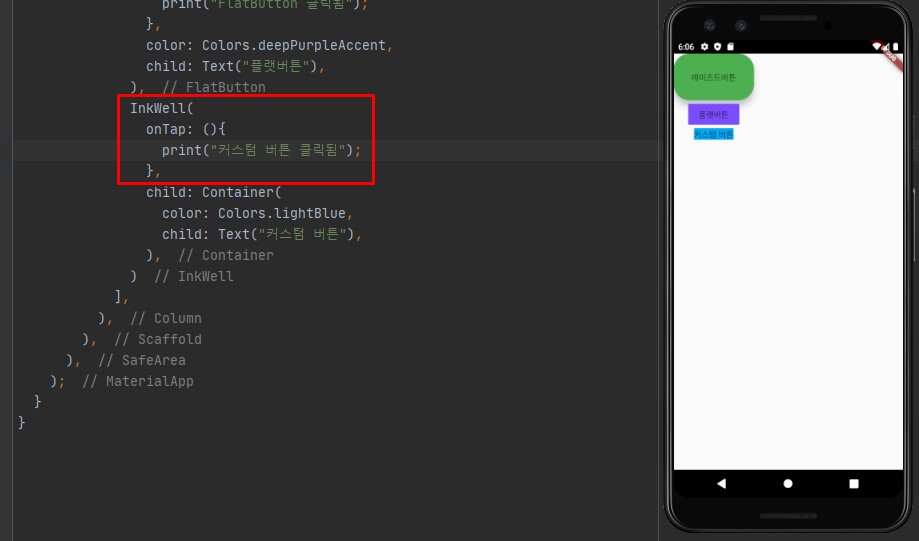
...
InkWell(
onTap: (){
print("커스텀 버튼 클릭됨");
},
child: Container(
color: Colors.lightBlue,
child: Text("커스텀 버튼"),
),
)
...
전체 코드
// ignore_for_file: prefer_const_literals_to_create_immutables, prefer_const_constructors
import 'package:flutter/material.dart';
// main 스레드는 runApp 을 실행시키고 종료됩니다.
void main() {
// 비동기로 실행됨(이벤트 루프에 등록된다)
runApp(FirstApp());
// sleep(Duration(seconds: 2));
// print("main 종료");
}
class FirstApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: SafeArea(
child: Scaffold(
body: Column(
children: [
RaisedButton(
onPressed: () {
print("RaisedButton 클릭됨");
},
color: Colors.green,
child: Text("레이즈드버튼"),
// 그림자 효과
elevation: 10,
// 테두리 모서리
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(27.5),
),
// 여백
padding: EdgeInsets.all(30),
),
FlatButton(
onPressed: () {
print("FlatButton 클릭됨");
},
color: Colors.deepPurpleAccent,
child: Text("플랫버튼"),
),
InkWell(
onTap: (){
print("커스텀 버튼 클릭됨");
},
child: Container(
color: Colors.lightBlue,
child: Text("커스텀 버튼"),
),
)
],
),
),
),
);
}
}