A
/ \
B---C
| A | B | C |
---|---|---|---|
A | 0 | 1 | 1 |
---|---|---|---|
B | 1 | 0 | 1 |
---|---|---|---|
C | 1 | 1 | 0 |
---|---|---|---|
INF = 999999999
graph = [
[0, 7, 5],
[7, 0, INF],
[5, INF, 0]
]
from QueType import *
from StackType import *
NULL_EDGE = 0
def index_is(vertices, vertex):
index = 0
while index < len(vertices) and vertex != vertices[index]:
index += 1
if not index < len(vertices):
return -1
else:
return index
class GraphType:
def __init__(self, maxV=50):
self.numVertices = 0
self.maxVertices = maxV
self.vertices = [None] * maxV
self.edges = [[NULL_EDGE] * maxV for _ in range(maxV)]
self.marks = [None] * maxV
def add_vertex(self, vertex):
self.vertices[self.numVertices] = vertex
for index in range(self.numVertices):
self.edges[self.numVertices][index] = NULL_EDGE
self.edges[index][self.numVertices] = NULL_EDGE
self.numVertices += 1
def add_edge(self, fromVertex, toVertex, weight):
row = index_is(self.vertices, fromVertex)
col = index_is(self.vertices, toVertex)
self.edges[row][col] = weight
def weight_is(self, fromVertex, toVertex):
row = index_is(self.vertices, fromVertex)
col = index_is(self.vertices, toVertex)
return self.edges[row][col]
def get_to_vertices(self, vertex, adjVertices):
fromIndex = index_is(self.vertices, vertex)
for toIndex in range(self.numVertices):
if(self.edges[fromIndex][toIndex] != NULL_EDGE):
adjVertices.enqueue(self.vertices[toIndex])
def clear_marks(self):
for index in range(self.numVertices):
self.marks[index] = False
def is_marked(self, vertex):
index = index_is(self.vertices, vertex)
return self.marks[index]
def mark_vertex(self, vertex):
index = index_is(self.vertices, vertex)
self.marks[index] = True
def delete_edge(self, fromVertex, toVertex):
row = index_is(self.vertices, fromVertex)
col = index_is(self.vertices, toVertex)
self.edges[row][col] = NULL_EDGE
template<class VertexType>
class GraphType
{
public:
GraphType();
GraphType(int maxV);
~GraphType();
void AddVertex(VertexType vertex);
void AddEdge(VertexType fromVertex, VertexType toVertex, int weight);
int WeightIs(VertexType fromVertex, VertexType toVertex);
void ClearMarks();
bool IsMarked(VertexType vertex);
void MarkVertex(VertexType vertex);
void GetToVertices(VertexType vertex, QueType<VertexType>& adjVertices);
private:
int numVertices;
int maxVertices;
VertexType* vertices;
int edges[50][50];
bool* marks;
};
// Constructor vertices라는 vertex 저장하는 1차원 array 생성
template<class VertexType>
GraphType<VertexType>::GraphType()
{
numVertices = 0;
maxVertices = 50;
vertices = new VertexType[50];
marks = new bool[50];
}
// Destructor for문 돌면서 vertex에 해당하는 edge들도 삭제
template<class VertexType>
GraphType<VertexType>::~GraphType()
{
delete[] vertices;
for (int i - 0; i < maxVertices; i++)
delete[] edges[i];
delete[] marks;
}
// AddVertex vertex array에 새로운 vertex를 넣어 주기
// 새로운 vertex와 연결된 edge는 모두 null로 초기화
// numVertices 하나 증가
template<class VertexType>
void GraphType<VertexType>::AddVertex(VertexType vertex)
{
vertices[numVertices] = vertex;
for (int index = 0; index < numVertices; index++)
{
edges[numVertices][index] = NULL_EDGE;
edges[index][numVertices] = NULL_EDGE;
}
numVertices++;
}
// AddEdges Edges array에 새로운 edge를 넣어 주기
// IndexIs vertex의 index 정볼ㄹ 저장하는 1차원 배열 vertices와 찾고자 하는 vertex 정보를 입력하면 해당 vertex의 index를 반환해 주는 함수
// row에는 edge가 뻗어나가는 vertex index 저장
// col에는 edge가 들어오는 vertex index 저장
// adjacent matrix에서 row, col 위치에 해당하는 곳에 edge 삽입
template<class VertexType>
int IndexIs(VertexType* vertices, VertexType vertex)
{
int index = 0;
while (!(vertex == vertices[index]))
index++;
return index;
}
template<class VertexType>
void GraphType<VertexType>::AddEdge(VertexType fromVertex, VertexType toVertex, int weight)
{
int row;
int col;
row = IndexIs(vertices, fromVertex);
col = IndexIs(vertices, toVertex);
edges[row][col] = weight;
}
// WeightIs fromVertex에서 toVertex로 가는 연결에 있는 가중치 반환하는 함수
//IndexIs vertex의 index 정보를 저장하는 1차원 배열 vertices와 찾고자 하는 vertex 정보를 입력하면 해당 vertex의 index를 반환해 주는 함수
// row에는 edge가 뻗어나가는 vertex index 저장
// col에는 edge가 들어오는 vertex index 저장
// adjacenct matrix에서 row, col 위치에 해당하는 곳의 edge 반환
template<class VertexType>
int GraphType<VertexType>::WeightIs (VertexType fromVertex, VertexType toVertex)
{
int row;
int col;
row = IndexIs(vertices, fromVertex);
col = IndexIs(vertices, toVertex);
return edges[row][col];
}
// travel 시에 방문했던 기록을 남기는 marks array 초기화
template<class VertexType>
void GraphType<VertexType>::ClearMarks()
{
for (int i = 0; i < numVertices; i++)
marks[i] = false;
}
// 해당 vertex가 방문한 기록이 있는지 물어보는 메소드
template<class VertexType>
bool GraphType<VertexType>::IsMarked(VertexType vertex)
{
index = IndexIs(vertices, vertex);
return (marks[index] == true);
}
// 해당 vertex를 방문했다고 기록하는 메소드
template<class VertexType>
void GraphType<VertexType>::MarkVertex(VertexType vertex)
{
index = IndexIs(vertices, vertex);
marks[index] = true;
}
// vertex에서 뻗어나간 edge랑 연결된 vertex를 adjVertices에 Enqueue하는 메소드
template<class VertexType>
void GraphType<VertexType>::GetToVertices(VertexType vertex, QueType<VertexType>& adjVertices)
{
int fromIndex;
int toIndex;
fromIndex = IndexIs(vertices, vertex);
for (toIndex = 0; toIndex < numVertices; toIndex++)
if (edges[fromIndex][toIndex] != NULL_EDGE)
adjVertices.Enqueue(vertices[toIndex]);
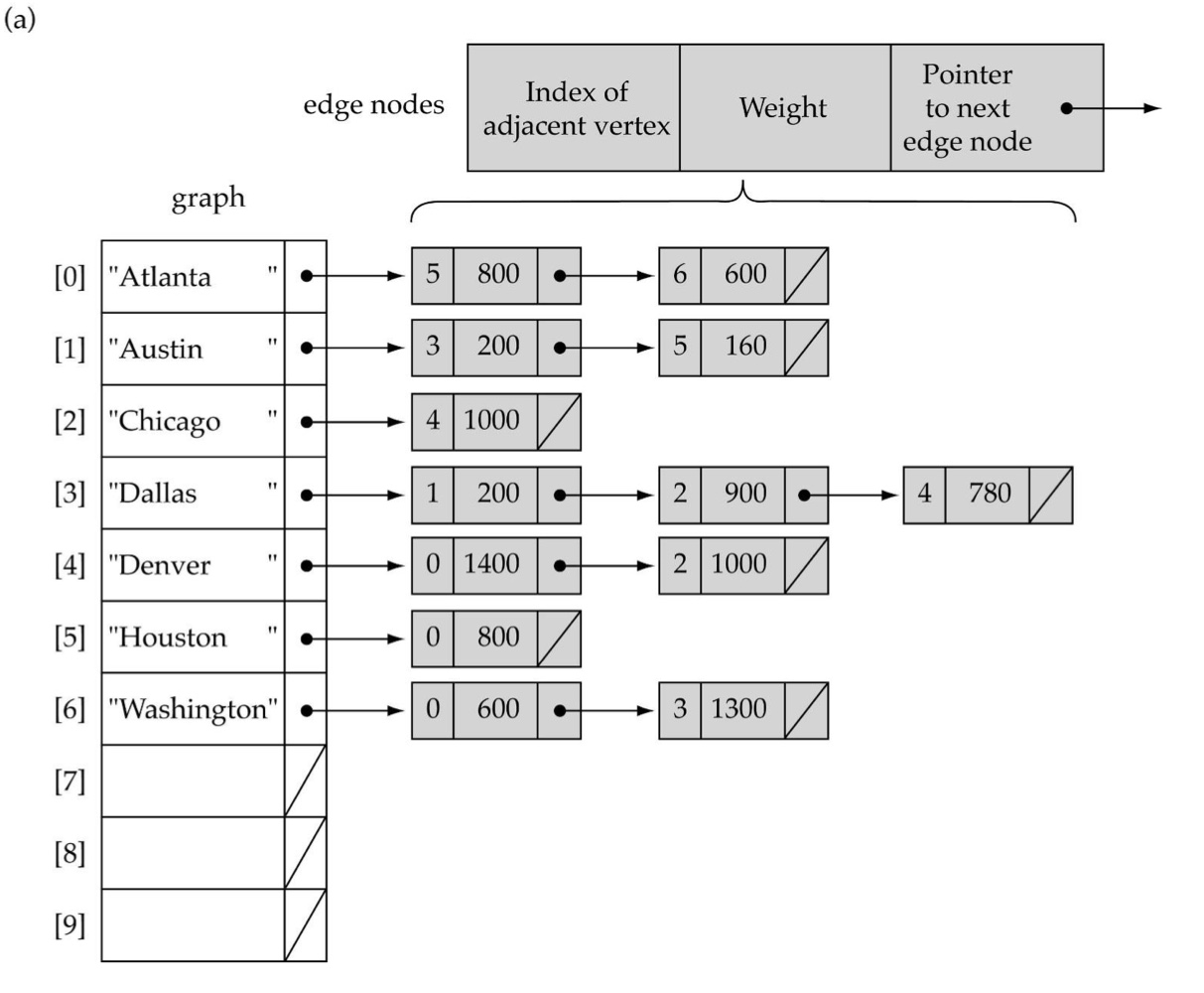
A
/ \
B---C
A -> [B, C]
B -> [A, C]
C -> [A, B]
graph = [[] for _ in range(3)]
graph[0].append((1, 7))
graph[0].append((2, 5))
graph[1].append((0, 7))
graph[2].append((0, 5))