트리거 발생 시 다리가 올라오는 현상 구현
// Fill out your copyright notice in the Description page of Project Settings.
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Actor.h"
#include "Components/BoxComponent.h"
#include "BricStairs.generated.h"
UCLASS()
class BRICKGAMEPROJECT_API ABricStairs : public AActor
{
GENERATED_BODY()
public:
ABricStairs();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
UPROPERTY(EditAnywhere)
UStaticMeshComponent* StairMesh;
UPROPERTY(EditAnywhere)
UBoxComponent* TriggerBox;
UPROPERTY(EditAnywhere)
float MoveSpeed = 100.f;
FVector StartRelativeLocation;
FVector TargetRelativeLocation;
bool bShouldMove = false;
UFUNCTION()
void OnTriggerBegin(UPrimitiveComponent* OverlappedComp, AActor* OtherActor,
UPrimitiveComponent* OtherComp, int32 OtherBodyIndex,
bool bFromSweep, const FHitResult& SweepResult);
};
#include "Character/BricStairs.h"
ABricStairs::ABricStairs()
{
PrimaryActorTick.bCanEverTick = true;
RootComponent = CreateDefaultSubobject<USceneComponent>(TEXT("RootComponent"));
StairMesh = CreateDefaultSubobject<UStaticMeshComponent>(TEXT("StairMesh"));
StairMesh->SetupAttachment(RootComponent);
TriggerBox = CreateDefaultSubobject<UBoxComponent>(TEXT("TriggerBox"));
TriggerBox->SetupAttachment(RootComponent);
TriggerBox->OnComponentBeginOverlap.AddDynamic(this, &ABricStairs::OnTriggerBegin);
}
void ABricStairs::BeginPlay()
{
Super::BeginPlay();
TargetRelativeLocation = StairMesh->GetRelativeLocation();
StartRelativeLocation = TargetRelativeLocation - FVector(0.f, 0.f, 300.f);
StairMesh->SetRelativeLocation(StartRelativeLocation);
}
void ABricStairs::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
if (bShouldMove)
{
FVector CurrentLocation = StairMesh->GetRelativeLocation();
FVector NewLocation = FMath::VInterpConstantTo(CurrentLocation, TargetRelativeLocation, DeltaTime, MoveSpeed);
StairMesh->SetRelativeLocation(NewLocation);
if (FVector::Dist(NewLocation, TargetRelativeLocation) < 1.f)
{
bShouldMove = false;
}
}
}
void ABricStairs::OnTriggerBegin(UPrimitiveComponent* OverlappedComp, AActor* OtherActor, UPrimitiveComponent* OtherComp, int32 OtherBodyIndex, bool bFromSweep, const FHitResult& SweepResult)
{
if (OtherActor && OtherActor != this)
{
bShouldMove = true;
}
}
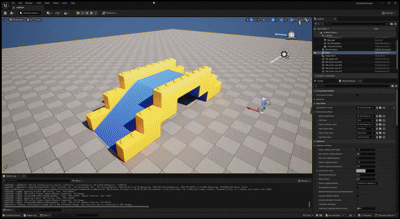