1.문제
An n x n matrix is valid if every row and every column contains all the integers from 1 to n (inclusive).
Given an n x n integer matrix matrix, return true if the matrix is valid. Otherwise, return false.
nxn 행렬이 주어질 때 각 행과 열의 숫자가 1~n 범위의 숫자들로 (중복없이) 구성되어있다면 true를 리턴하는 문제이다.
Example 1
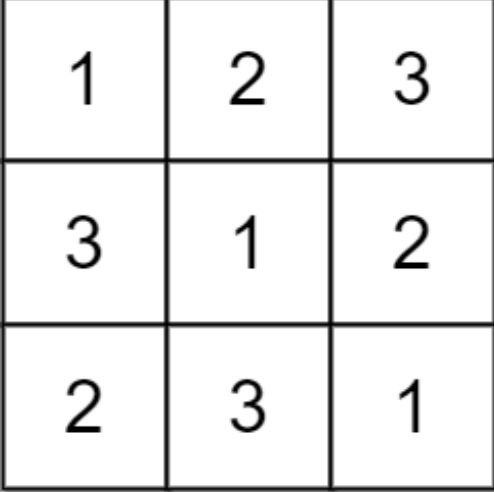
Input: matrix = [[1,2,3],[3,1,2],[2,3,1]]
Output: true
Explanation: In this case, n = 3, and every row and column contains the numbers 1, 2, and 3.
Hence, we return true.
Example 2
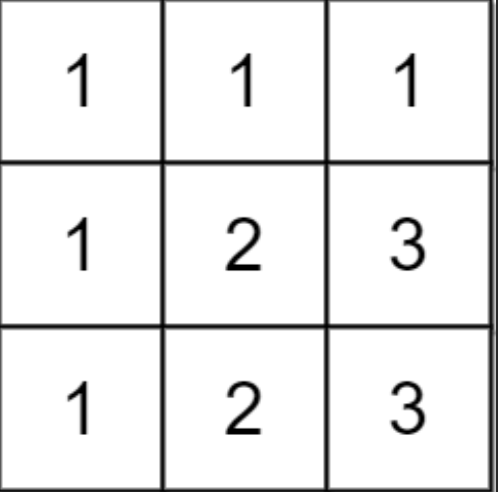
Input: matrix = [[1,1,1],[1,2,3],[1,2,3]]
Output: false
Explanation: In this case, n = 3, but the first row and the first column do not contain the numbers 2 or 3.
Hence, we return false.
Constraints:
- n == matrix.length == matrix[i].length
- 1 <= n <= 100
- 1 <= matrix[i][j] <= n
2.풀이
- 각 행의 배열을 중복을 없앤 후 n과 길이를 비교하여 다르면 바로 false를 리턴한다.
- 각 행과 열을 체크한다.
/**
* @param {number[][]} matrix
* @return {boolean}
*/
const checkValid = function (matrix) {
const n = matrix.length;
const column = [];
// matrix의 각 행을 체크한다.
for (let i = 0; i < n; i++) {
const set = [...new Set(matrix[i])];
if (set.length !== n) return false;
}
// matrix의 column 배열을 만든다
for (let i = 0; i < n; i++) {
let temp = [];
for (let j = 0; j < n; j++) {
temp.push(matrix[j][i]);
}
column.push(temp);
}
// matrix의 column을 체크한다.
for (let i = 0; i < n; i++) {
const set = [...new Set(column[i])];
if (set.length !== n) return false;
}
return true;
};
3.결과
