✏️ Users
package LoginEx;
class User {
private String id;
private String pw;
private String name;
private String nickName;
private String gender;
public User(String id, String pw, String name, String nickName, String gender) {
setId(id);
setPw(pw);
setName(name);
setNickName(nickName);
setGender(gender);
}
public User(String id) {
setId(id);
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getPw() {
return pw;
}
public void setPw(String pw) {
this.pw = pw;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getNickName() {
return nickName;
}
public void setNickName(String nickName) {
this.nickName = nickName;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
@Override
public boolean equals(Object o) {
if(o == null || !(o instanceof User)) {
return false;
}
User temp = (User)o;
return id.equals(temp.getId());
}
@Override
public String toString() {
String info = "Id: " + id + "\n";
info += "Pw: " + pw + "\n";
info += "Name: " + name + "\n";
info += "NickName: " + nickName + "\n";
info += "gender: " + gender + "\n";
return info;
}
}
✏️ UserDataSet
package LoginEx;
import java.util.ArrayList;
public class UserDataSet {
private ArrayList<User> users;
public UserDataSet() {
users = new ArrayList<User>();
}
public void addUsers(User user) {
users.add(user);
}
public boolean isIdOverlap(String id) {
return users.contains(new User(id));
}
public void withdraw(String id) {
users.remove(getUser(id));
}
public User getUser(String id) {
return users.get(users.indexOf(new User(id)));
}
public boolean contains(User user) {
return users.contains(user);
}
}
- ID, Password 입력
: 가입내용 기반
- 회원가입 및 로그인 기능 제공
package LoginEx;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import javax.swing.text.JTextComponent;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.util.ArrayList;
public class LoginForm extends JFrame {
private UserDataSet users;
private JLabel lblId;
private JLabel lblPw;
private JTextField tfId;
private JPasswordField tfPw;
private JButton btnLogin;
private JButton btnJoin;
public LoginForm() {
users = new UserDataSet();
init();
setDisplay();
addListeners();
showFrame();
}
public void init() {
Dimension lblSize = new Dimension(80, 30);
int tfSize = 10;
Dimension btnSize = new Dimension(100, 25);
lblId = new JLabel("ID");
lblId.setPreferredSize(lblSize);
lblPw = new JLabel("Password");
lblPw.setPreferredSize(lblSize);
tfId = new JTextField(tfSize);
tfPw = new JPasswordField(tfSize);
btnLogin = new JButton("Login");
btnLogin.setPreferredSize(btnSize);
btnJoin = new JButton("Join");
btnJoin.setPreferredSize(btnSize);
}
public UserDataSet getUsers() {
return users;
}
public String getTfId() {
return tfId.getText();
}
public void setDisplay() {
FlowLayout flowLeft = new FlowLayout(FlowLayout.LEFT);
JPanel pnlNorth = new JPanel(new GridLayout(0, 1));
JPanel pnlId = new JPanel(flowLeft);
pnlId.add(lblId);
pnlId.add(tfId);
JPanel pnlPw = new JPanel(flowLeft);
pnlPw.add(lblPw);
pnlPw.add(tfPw);
pnlNorth.add(pnlId);
pnlNorth.add(pnlPw);
JPanel pnlSouth = new JPanel();
pnlSouth.add(btnLogin);
pnlSouth.add(btnJoin);
pnlNorth.setBorder(new EmptyBorder(0, 20, 0, 20));
pnlSouth.setBorder(new EmptyBorder(0, 0, 10, 0));
add(pnlNorth, BorderLayout.NORTH);
add(pnlSouth, BorderLayout.SOUTH);
}
public void addListeners() {
btnJoin.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
setVisible(false);
new JoinForm(LoginForm.this);
tfId.setText("");
tfPw.setText("");
}
});
btnLogin.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (tfId.getText().isEmpty()) {
JOptionPane.showMessageDialog(LoginForm.this,
"아이디를 입력하세요.",
"로그인폼",
JOptionPane.WARNING_MESSAGE);
} else if (users.contains(new User(tfId.getText()))) {
if(String.valueOf(tfPw.getPassword()).isEmpty()) {
JOptionPane.showMessageDialog(
LoginForm.this,
"비밀번호를 입력하세요.",
"로그인폼",
JOptionPane.WARNING_MESSAGE);
} else if (!users.getUser(tfId.getText()).getPw().equals(String.valueOf(tfPw.getPassword()))) {
JOptionPane.showMessageDialog(
LoginForm.this,
"비밀번호가 일치하지 않습니다.");
} else {
InformationForm infoForm = new InformationForm(LoginForm.this);
infoForm.setTaCheck(users.getUser(tfId.getText()).toString());
setVisible(false);
infoForm.setVisible(true);
tfId.setText("");
tfPw.setText("");
}
} else {
JOptionPane.showMessageDialog(
LoginForm.this,
"존재하지 않는 Id입니다."
);
}
}
});
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent we) {
int choice = JOptionPane.showConfirmDialog(
LoginForm.this,
"로그인 프로그램을 종료합니다.",
"종료",
JOptionPane.OK_CANCEL_OPTION,
JOptionPane.WARNING_MESSAGE
);
if (choice == JOptionPane.YES_OPTION) {
System.exit(0);
}
}
});
}
public void showFrame() {
setTitle("Login");
pack();
setLocationRelativeTo(null);
setDefaultCloseOperation(DO_NOTHING_ON_CLOSE);
setResizable(false);
setVisible(true);
}
public static void main(String[] args) {
new LoginForm();
}
}
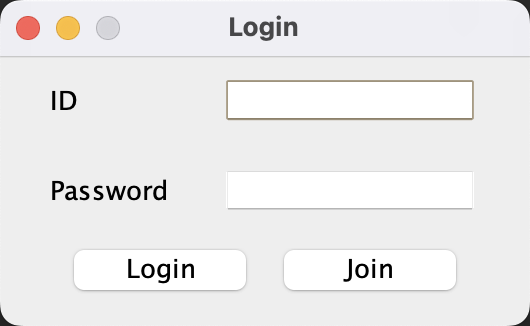
- 각 정보입력
- 모든 입력정보가 필수
- ID는 중복해서 존재할 수 없음
- 비밀번호는 두 가지 입력이 동일한 경우만 가능
: Password, Retry
package LoginEx;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import javax.swing.border.TitledBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class JoinForm extends JDialog {
private LoginForm owner;
private UserDataSet users;
private JLabel lblTitle;
private JLabel lblId;
private JLabel lblPw;
private JLabel lblRe;
private JLabel lblName;
private JLabel lblNickName;
private JRadioButton rbtnMale;
private JRadioButton rbtnFemale;
private JTextField tfId;
private JPasswordField tfPw;
private JPasswordField tfRe;
private JTextField tfName;
private JTextField tfNickName;
private JButton btnJoin;
private JButton btnCancel;
public JoinForm(LoginForm owner) {
super(owner, "Join", true);
this.owner = owner;
users = owner.getUsers();
init();
setDisplay();
addListeners();
showFrame();
}
private void init() {
int tfSize = 10;
Dimension lblSize = new Dimension(80, 35);
Dimension btnSize = new Dimension(100 ,25);
lblTitle = new JLabel("- Input your information");
lblTitle.setFont(new Font(Font.SANS_SERIF, Font.BOLD, 14));
lblId = new JLabel("ID", JLabel.LEFT);
lblId.setPreferredSize(lblSize);
lblPw = new JLabel("Password", JLabel.LEFT);
lblPw.setPreferredSize(lblSize);
lblRe = new JLabel("Retry", JLabel.LEFT);
lblRe.setPreferredSize(lblSize);
lblName = new JLabel("Name", JLabel.LEFT);
lblName.setPreferredSize(lblSize);
lblNickName = new JLabel("NickName", JLabel.LEFT);
lblNickName.setPreferredSize(lblSize);
tfId = new JTextField(tfSize);
tfPw = new JPasswordField(tfSize);
tfRe = new JPasswordField(tfSize);
tfName = new JTextField(tfSize);
tfNickName = new JTextField(tfSize);
rbtnMale = new JRadioButton("Male", true);
rbtnFemale = new JRadioButton("Female");
ButtonGroup group = new ButtonGroup();
group.add(rbtnMale);
group.add(rbtnFemale);
btnJoin = new JButton("Join");
btnJoin.setPreferredSize(btnSize);
btnCancel = new JButton("Cancel");
btnCancel.setPreferredSize(btnSize);
}
private void setDisplay() {
FlowLayout flowLeft = new FlowLayout(FlowLayout.LEFT);
JPanel pnlMain = new JPanel(new BorderLayout());
JPanel pnlMNorth = new JPanel(flowLeft);
pnlMNorth.add(lblTitle);
JPanel pnlMCenter = new JPanel(new GridLayout(0, 1));
JPanel pnlId = new JPanel(flowLeft);
pnlId.add(lblId);
pnlId.add(tfId);
JPanel pnlPw = new JPanel(flowLeft);
pnlPw.add(lblPw);
pnlPw.add(tfPw);
JPanel pnlRe = new JPanel(flowLeft);
pnlRe.add(lblRe);
pnlRe.add(tfRe);
JPanel pnlName = new JPanel(flowLeft);
pnlName.add(lblName);
pnlName.add(tfName);
JPanel pnlNickName = new JPanel(flowLeft);
pnlNickName.add(lblNickName);
pnlNickName.add(tfNickName);
pnlMCenter.add(pnlId);
pnlMCenter.add(pnlPw);
pnlMCenter.add(pnlRe);
pnlMCenter.add(pnlName);
pnlMCenter.add(pnlNickName);
JPanel pnlMSouth = new JPanel(new FlowLayout(FlowLayout.CENTER));
pnlMSouth.add(rbtnMale);
pnlMSouth.add(rbtnFemale);
pnlMSouth.setBorder(new TitledBorder("Gender"));
pnlMain.add(pnlMNorth, BorderLayout.NORTH);
pnlMain.add(pnlMCenter, BorderLayout.CENTER);
pnlMain.add(pnlMSouth, BorderLayout.SOUTH);
JPanel pnlSouth = new JPanel();
pnlSouth.add(btnJoin);
pnlSouth.add(btnCancel);
pnlMain.setBorder(new EmptyBorder(0, 20, 0, 20));
pnlSouth.setBorder(new EmptyBorder(0, 0, 10, 0));
add(pnlMain, BorderLayout.NORTH);
add(pnlSouth, BorderLayout.SOUTH);
}
private void addListeners() {
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent we) {
dispose();
owner.setVisible(true);
}
});
btnCancel.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent ae) {
dispose();
owner.setVisible(true);
}
});
btnJoin.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent ae) {
if(isBlank()) {
JOptionPane.showMessageDialog(
JoinForm.this,
"모든 정보를 입력해주세요."
);
} else {
if(users.isIdOverlap(tfId.getText())) {
JOptionPane.showMessageDialog(
JoinForm.this,
"이미 존재하는 Id입니다."
);
tfId.requestFocus();
} else if(!String.valueOf(tfPw.getPassword()).equals(String.valueOf(tfRe.getPassword()))) {
JOptionPane.showMessageDialog(
JoinForm.this,
"Password와 Retry가 일치하지 않습니다."
);
tfPw.requestFocus();
} else {
users.addUsers(new User(
tfId.getText(),
String.valueOf(tfPw.getPassword()),
tfName.getText(),
tfNickName.getText(),
getGender())
);
JOptionPane.showMessageDialog(
JoinForm.this,
"회원가입을 완료했습니다!"
);
dispose();
owner.setVisible(true);
}
}
}
});
}
public boolean isBlank() {
boolean result = false;
if(tfId.getText().isEmpty()) {
tfId.requestFocus();
return true;
}
if(String.valueOf(tfPw.getPassword()).isEmpty()) {
tfPw.requestFocus();
return true;
}
if(String.valueOf(tfRe.getPassword()).isEmpty()) {
tfRe.requestFocus();
return true;
}
if(tfName.getText().isEmpty()) {
tfName.requestFocus();
return true;
}
if(tfNickName.getText().isEmpty()) {
tfNickName.requestFocus();
return true;
}
return result;
}
public String getGender() {
if(rbtnMale.isSelected()) {
return rbtnMale.getText();
}
return rbtnFemale.getText();
}
private void showFrame() {
pack();
setLocationRelativeTo(owner);
setDefaultCloseOperation(DISPOSE_ON_CLOSE);
setResizable(false);
setVisible(true);
}
}
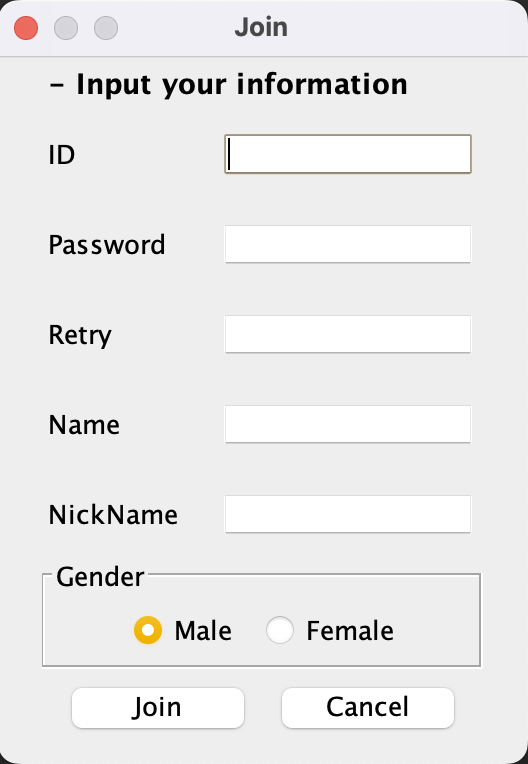
package LoginEx;
import javax.swing.*;
import javax.swing.border.LineBorder;
import javax.swing.border.TitledBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class InformationForm extends JDialog {
private LoginForm owner;
private UserDataSet users;
private JTextArea taCheck;
private JButton btnLogout;
private JButton btnWithdraw;
private JoinForm joinForm;
public InformationForm(LoginForm owner) {
super(owner, "Information", true);
this.owner = owner;
users = owner.getUsers();
init();
setDisplay();
addListeners();
showFrame();
}
private void init() {
Dimension btnsize = new Dimension(100, 25);
taCheck = new JTextArea(10, 30);
taCheck.setEditable(false);
btnLogout = new JButton("Logout");
btnLogout.setPreferredSize(btnsize);
btnWithdraw = new JButton("withdraw");
btnWithdraw.setPreferredSize(btnsize);
}
private void setDisplay() {
LineBorder lBorder = new LineBorder(Color.GRAY, 1);
TitledBorder border = new TitledBorder(lBorder, "check your Information");
taCheck.setBorder(border);
JPanel pnlSouth = new JPanel();
pnlSouth.add(btnLogout);
pnlSouth.add(btnWithdraw);
JPanel pnlMain = new JPanel(new BorderLayout());
pnlMain.add(new JScrollPane(taCheck), BorderLayout.NORTH);
pnlMain.add(pnlSouth, BorderLayout.CENTER);
add(pnlMain,BorderLayout.CENTER);
}
private void addListeners() {
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent we) {
dispose();
}
});
btnWithdraw.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent ae) {
users.withdraw(owner.getTfId());
JOptionPane.showMessageDialog(
InformationForm.this,
"회원 정보가 삭제되었습니다. 안녕히가세요."
);
dispose();
owner.setVisible(true);
}
});
btnLogout.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent ae) {
JOptionPane.showMessageDialog(
InformationForm.this,
"로그아웃 되었습니다."
);
dispose();
owner.setVisible(true);
}
});
}
public void setTaCheck(String userInfo) {
taCheck.setText(userInfo);
}
private void showFrame() {
pack();
setLocationRelativeTo(owner);
setDefaultCloseOperation(DISPOSE_ON_CLOSE);
setResizable(false);
}
}
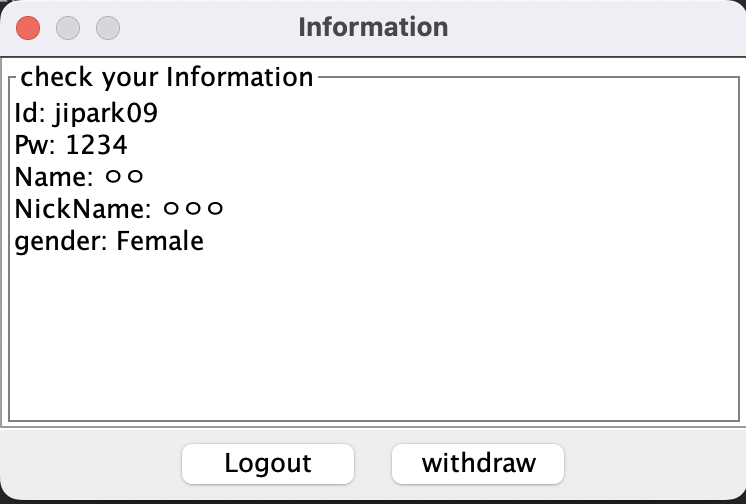