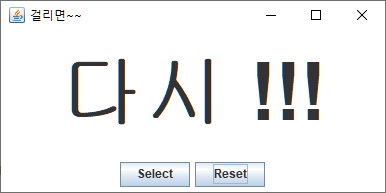
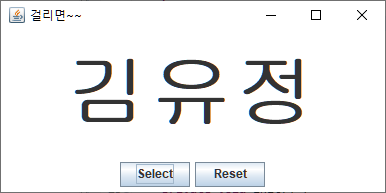
✏️ SelectStudent
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
import java.util.*;
class SelectStudent extends Frame implements ActionListener {
private JLabel lblName;
private JButton btnStop;
private JButton btnReset;
private boolean isStop;
private Vector<String> names;
public SelectStudent() {
super("걸리면~~");
init();
setLayout();
addListener();
showFrame();
start();
}
private void init() {
loadStudentList();
Dimension btnSize = new Dimension(70, 25);
lblName = new JLabel("ㅋㅋㅋ", JLabel.CENTER);
lblName.setFont(new Font("Dialog", Font.BOLD, 80));
btnStop = new JButton("Select");
btnStop.setPreferredSize(btnSize);
btnReset = new JButton("Reset");
btnReset.setPreferredSize(btnSize);
}
private void setLayout() {
add(lblName, BorderLayout.CENTER);
Panel pnlSouth = new Panel();
pnlSouth.add(btnStop);
pnlSouth.add(btnReset);
add(pnlSouth, BorderLayout.SOUTH);
}
private void addListener() {
btnStop.addActionListener(this);
btnReset.addActionListener(this);
addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent we) {
System.exit(0);
}
});
}
private void showFrame() {
setLocation(400, 200);
setSize(400, 200);
setVisible(true);
}
private boolean loadStudentList() {
try {
BufferedReader br = new BufferedReader(new FileReader("list.dat"));
names = new Vector<String>();
String temp = null;
while ((temp = br.readLine()) != null) {
names.add(temp);
}
return true;
} catch (IOException ioe) {
return false;
}
}
public void actionPerformed(ActionEvent ae) {
if (ae.getSource() == btnStop) {
isStop = !isStop;
if (!isStop) {
start();
}
} else {
loadStudentList();
lblName.setText("다시 !!!");
}
}
private void start() {
Thread t = new Thread(new RandomList(this));
t.start();
}
public void setName(String name) {
lblName.setText(name);
}
public boolean isStop() {
return isStop;
}
public Vector<String> getNames() {
return names;
}
public void setNames(Vector<String> names) {
names = names;
}
public static void main(String[] args) {
new SelectStudent();
}
}
✏️ RandomList
import java.io.*;
import java.util.*;
class RandomList implements Runnable {
private Vector<String> names;
private SelectStudent owner;
public RandomList(SelectStudent owner) {
this.owner = owner;
names = owner.getNames();
if (names == null) {
System.exit(0);
}
}
public void run() {
try {
int idx = 0;
String name = "";
while (!owner.isStop()) {
Thread.sleep(30);
name = names.get(idx++);
owner.setName(name);
if (idx == names.size()) {
idx = 0;
}
}
names.remove(name);
Collections.shuffle(names);
} catch (Exception e) {}
}
}