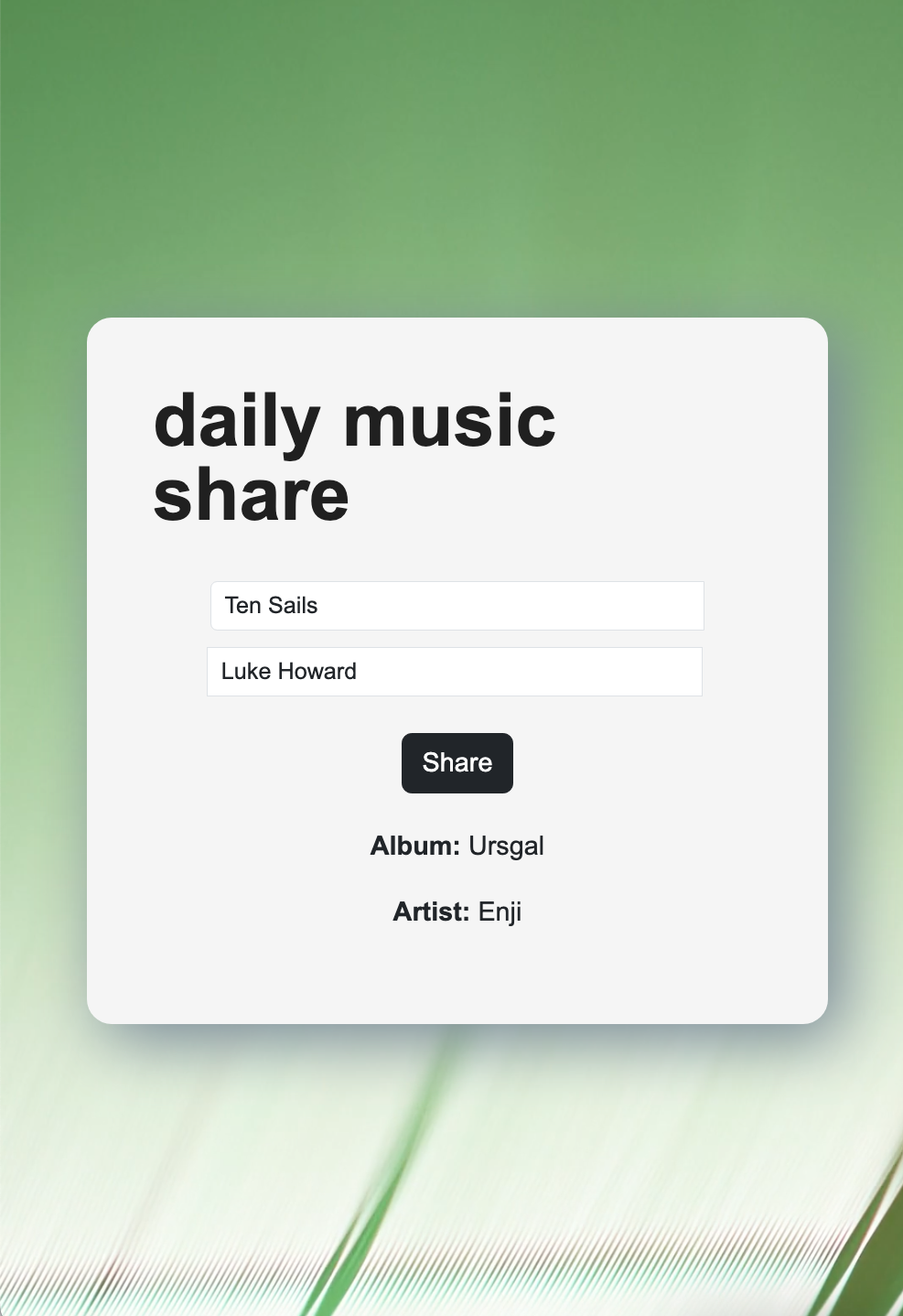
video document
- CRUD 구조 공부를 하기 위해, 작은 사이드 프로젝트를 했다.
- 동적 웹 페이지를 만들어 보았다.
- 유저의 추천 음악 앨범의 정보를 DB에 받는다.
- 지금까지 DB에 저장되어 있던 랜덤한 음악 앨범을 유저에게 돌려준다.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link
href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.1/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity="sha384-4bw+/aepP/YC94hEpVNVgiZdgIC5+VKNBQNGCHeKRQN+PtmoHDEXuppvnDJzQIu9"
crossorigin="anonymous"
/>
<title>:: DMS :: daily music share</title>
<link rel="stylesheet" type="text/css" href="../static/css/main.css">
</head>
<body>
<video id="video-background" autoplay loop muted>
<source
src="../static/img/pexels-eva-elijas-7606282.mp4"
type="video/mp4"
/>
</video>
<div class="box">
<a href="" class="title" id="title">daily music share</a>
<div class="inputer">
<div class="input-group input-group-sm mb-3">
<input
id="album_name"
type="text"
class="form-control"
aria-label="Sizing example input"
aria-describedby="inputGroup-sizing-sm"
placeholder="insert album title"
/><div class="input-group input-group-sm mb-3">
<br>
<input
id="artist"
type="text"
class="form-control"
aria-label="Sizing example input"
aria-describedby="inputGroup-sizing-sm"
placeholder="insert artist"
/><br>
</div>
</div>
<button onclick="save_album()" type="button" class="btn btn-dark">
Share
</button>
<div class="album-info" id="album-info"></div>
</div>
<script>
document.getElementById("title").addEventListener("click", function () {
window.location.href = "http://127.0.0.1:5500/index.html";
});
function show_album(album_info) {
if (!album_info || album_info.error) {
$("#album-info").text("No albums found.");
return;
}
$("#album-info").html(`
<p><strong>Album Name:</strong> ${album_info.album_name}</p>
<p><strong>Artist:</strong> ${album_info.artist}</p>
`);
}
function get_random_shared_album() {
fetch("/get_random_shared_album")
.then((response) => response.json())
.then((album_info) => {
show_album(album_info);
});
}
function save_album() {
let album_name = $("#album_name").val();
let artist = $("#artist").val();
let formData = new FormData();
formData.append("album_name", album_name);
formData.append("artist", artist);
fetch("/save_album", { method: "POST", body: formData })
.then((response) => response.json())
.then((data) => {
alert(data["msg"]);
get_random_shared_album();
});
}
</script>
<script
src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.1/dist/js/bootstrap.bundle.min.js"
integrity="sha384-HwwvtgBNo3bZJJLYd8oVXjrBZt8cqVSpeBNS5n7C8IVInixGAoxmnlMuBnhbgrkm"
crossorigin="anonymous"
></script>
</body>
</html>
from flask import Flask, render_template, request, jsonify
from flask_cors import CORS
import random
app = Flask(__name__)
CORS(app)
from pymongo import MongoClient
import certifi
import requests
from bs4 import BeautifulSoup
ca = certifi.where()
url = 'mongodb+srv://sparta:test@cluster0.fuzltx4.mongodb.net/?retryWrites=true&w=majority'
client = MongoClient(url, tlsCAFile=ca)
db = client.dbsparta
@app.route('/')
def home():
return render_template('index.html')
@app.route("/save_album", methods=["POST"])
def save_album():
try:
album_name = request.form.get('album_name', '').strip()
artist = request.form.get('artist', '').strip()
if album_name and artist:
album_info = {
"album_name": album_name,
"artist": artist,
}
db.albums.insert_one(album_info)
return jsonify({'msg': 'Thank you for sharing!'})
else:
return jsonify({'error': 'Invalid album information'})
except Exception as e:
return jsonify({'error': str(e)})
@app.route("/get_random_shared_album")
def get_random_shared_album():
try:
all_albums = list(db.albums.find({}, {'_id': False}))
if all_albums:
random_album = random.choice(all_albums)
return jsonify(random_album)
else:
return jsonify({'error': 'No albums found'})
except Exception as e:
return jsonify({'error': str(e)})
@app.route("/albums", methods=["GET"])
def albums_get():
all_id = list(db.albums.find({},{'_id':False}))
return jsonify({'result': all_id})
if __name__ == '__main__':
app.run('0.0.0.0', port=5000, debug=True)