DFS와 BFS [C++]
난이도: ⚪⚪
문제 설명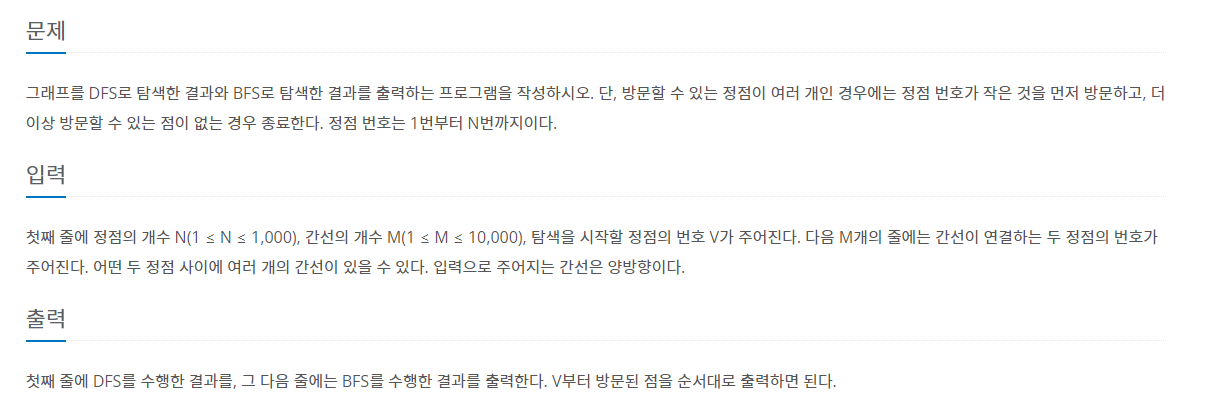
문제 접근
- DFS는 재귀를 이용하고, BFS는 queue자료형을 이용하여 구현한다.
- 정점 번호가 작은 것을 먼저 방문하기 때문에 연결된 정점들을 정렬한다.
- 두 정점 사이에 여러 간선이 있을 수 있고, 양뱡향임을 유의한다.
제출 코드
#include<iostream>
#include<vector>
#include<queue>
#include<algorithm>
using namespace std;
vector<int> graph[1001];
bool visited[1001];
void dfs(int node)
{
visited[node] = true;
cout << node << " ";
for (int i = 0; i < graph[node].size(); i++)
if (!visited[graph[node][i]])
dfs(graph[node][i]);
}
void bfs(int node)
{
visited[node] = true;
cout << node << " ";
queue<int> q;
q.push(node);
while (!q.empty())
{
int cur_node = q.front();
q.pop();
for (int i = 0; i < graph[cur_node].size(); i++)
{
if (visited[graph[cur_node][i]] == false)
{
visited[graph[cur_node][i]] = true;
cout << graph[cur_node][i] << " ";
q.push(graph[cur_node][i]);
}
}
}
}
int main(void)
{
int N, M, V;
cin >> N >> M >> V;
for (int i = 0; i < M; i++)
{
int node1, node2;
cin >> node1 >> node2;
graph[node1].push_back(node2);
graph[node2].push_back(node1);
}
for (int i = 0; i < N; i++)
sort(graph[i + 1].begin(), graph[i + 1].end());
dfs(V);
for (int i = 0; i < sizeof(visited); i++)
visited[i] = false;
cout << '\n';
bfs(V);
return 0;
}
결과