1. Randomisation (무작위화)
import random
i = 100
j = 20e7
a = random.randrange(i, j)
try:
b = random.randrange(j, i)
except ValueError:
print('ValueError on randrange() since start > stop')
c = random.randint(100, 200)
try:
d = random.randint(200, 100)
except ValueError:
print('ValueError on randint() since 200 > 100')
print('i =', i, ' and j =', j)
print('randrange() generated number:', a)
print('randint() generated number:', c)
2. module
- module : 코드가 너무 길어지면 이해하기 어려움. 코드를 개별 모듈로 개발하고, 각 모듈은 서로 다른 기능을 담당하게 됨. randint 함수 또한 난수 생성을 쉽게 하기 위해 개발된 random 모듈의 기능 중 하나임.
pi = 3.14159246
import my_module #module import
print(my_module.pi) # 3.14159246 출력
3. quiz _ coins
import random
number = random.randint(0,1)
if number ==0 :
print("Tails")
else:
print("Heads")
4. 오프셋을 이해하고 리스트에 항목 추가하기
- 파이썬 리스트 : 데이터 구조, 데이터를 체계화하고 저장하는 방법. ex) fruits = ["cherry","apple","grape"]
- print(list_name[1]) : list의 두번째 값 가져오기. (python은 0부터 시작함)
- 0,1,2 -> 리스트 시작부분에서 얼만큼 이동했는지로 생각함.
- print(list_name[-1]) : list의 맨 마지막 값
- list_name[1] = "Pencilvania" -> 데이터 값을 바꿀 수 있음.
- list_name.append("Angelaland") -> list에 데이터 값 추가 가능.
- list_name.extend(["Angelaland","Jack Bauer land"]) : list에 list 자체를 추가 가능함.
- https://docs.python.org/3/tutorial/datastructures.html
5. quiz _ pay dinner
names_string = input("Give me everybody's names, separated by a comma. ")
names = names_string.split(", ")
import random
choice = random.choice(names)
print(f"{choice} is going to buy the meal today!")
num_items = len(names)
random_choice = random.randidnt(0,num_items-1)
person_who_will_pay = names[random_choice]
print((person_who_will_pay+"is going to buy the meatl today!"))
6. index error 및 중첩 리스트 활용하기
- off-by-one 오류 : 인덱스 숫자가 1만큼 차이가 나서 발생하는 오류 (리스트의 총 길이는 50인데, 51번째 아이템을 추출하려는 경우 등)
list_a =. ["a","b","c","d"]
list_b = ["f,"g","h","i"]
list = [list_a, list_b]
print(list)
- output : [["a","b","c","d"],["f,"g","h","i"]]
7. quiz
fruits = ["Strawberries", "Nectarines", "Apples", "Grapes", "Peaches", "Cherries", "Pears"]
vegetables = ["Spinach", "Kale", "Tomatoes", "Celery", "Potatoes"]
dirty_dozen = [fruits, vegetables]
print(dirty_dozen[1][1])
8. quiz _ treasure map
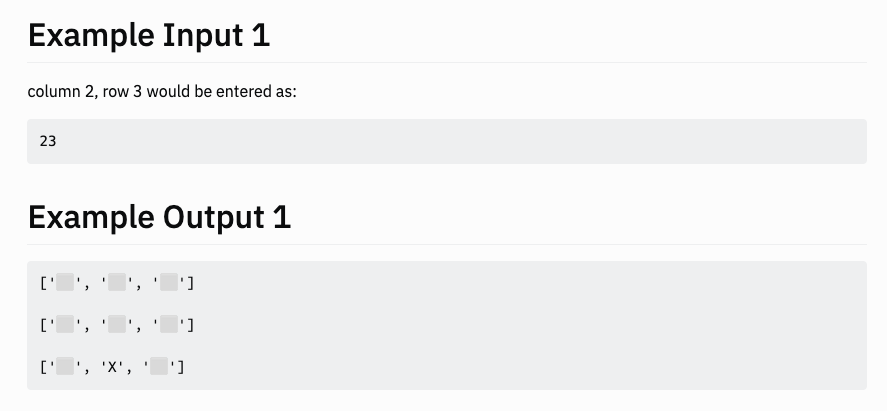
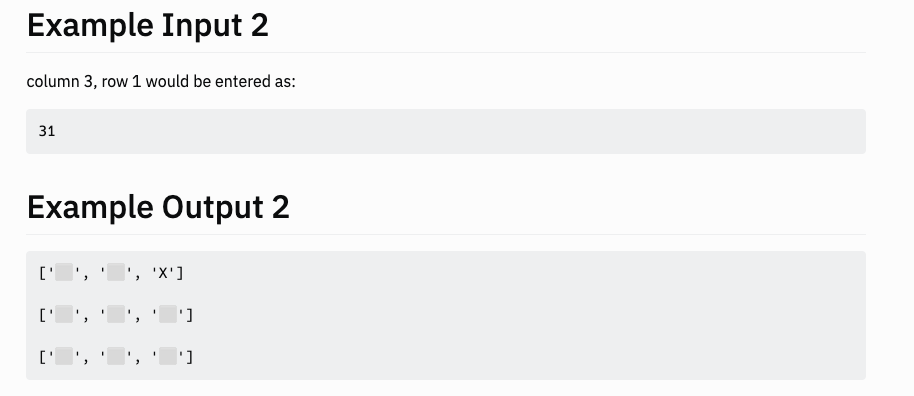
row1 = ["⬜️","⬜️","⬜️"]
row2 = ["⬜️","⬜️","⬜️"]
row3 = ["⬜️","⬜️","⬜️"]
map = [row1, row2, row3]
print(f"{row1}\n{row2}\n{row3}")
position = input("Where do you want to put the treasure? ")
horizonal = int(position[0])
vertical = int(position[1])
map[vertical-1][horizonal-1] = "X"
print(f"{row1}\n{row2}\n{row3}")
9. project - rock, scissor, paper
rock = '''
_______
---' ____)
(_____)
(_____)
(____)
---.__(___)
'''
paper = '''
_______
---' ____)____
______)
_______)
_______)
---.__________)
'''
scissors = '''
_______
---' ____)____
______)
__________)
(____)
---.__(___)
'''
import random
choice = int(input("What do you choose? Type 0 for Rock, 1 for Paper of 2 for Scissors. "))
com_choice = random.randint(0,2)
if choice == 0:
if com_choice == 1:
print("computer choose is paper, You lose")
print(paper)
elif com_choice ==0:
print(rock)
else:
print("You win")
print(rock)
elif choice == 1:
if com_choice == 2:
print("computer choose was scissors, you lose ")
print(scissors)
elif com_choice == 1:
print(paper)
else:
print("You win")
print(paper)
elif choice == 2:
if com_choice == 2 :
print("computer choose was rock, you lose")
print(rock)
elif com_choice == 1:
print(scissors)
else:
print("you win")
print(scissors)
else:
print("you typed wrong number! you lose")