Annotation
- 코드 사이에서 특별한 기능을 수행하게 하는 메타데이터
- 메타데이터: 다른 데이터를 설명해주는 데이터. 데이터의 표현과 검색을 용이하게 해줌
주요 어노테이션
- built-in 어노테이션: Java코드에 바로 적용되는 어노테이션
- meta 어노테이션: 다른 어노테이션에 적용하는 어노테이션
@Bean
- 직접 제어가 불가능한 외부 라이브러리등을 Bean으로 등록
@Component
@Autowired
@Controller
@RestController
@Service
- 비즈니스 로직을 수행하는 Service로 사용할 클래스 지정
@Repository
@RequestMapping
@ModelAttribute
- view에서 받은 Parameter를 VO나 DTO의 멤버변수로 바인딩
@~Mapping
- @GetMapping / @PostMapping / @PutMapping / @PatchMapping / @DeleteMapping
- 요청method와 URL별로 수행 될 클래스 지정
@SessionAttributes
@SessionAttributes("student") // Model에 키 값이 "student"로 지정되는 값은 모두 세션에 저장됨
@RequestParam
http://localhost/detail?studentNo=13
@GetMapping("/detail")
public String studentDetail(@RequestParam("studentNo") int studentNo){ ... }
@RequestBody
- JSON이나 XML형식의 데이터를 자바객체로 변환
@ControllerAdvice / @RestControllerAdvice
- 어플리케이션 내 모든 Controller/RestController의 예외를 처리
@Transactional
- db의 begin, commit, rollback을 자동으로 수행
- service에서 사용
@Scheduled
사용자정의 어노테이션

@Target({ElementType.적용위치}) // 1
@Retention(RetentionPolicy.적용범위) // 2
public @interface LogExclusion {
// ...
}
- 반드시 @interface로 정의
- 반환값은 기본타입과 String, Enum, Class, 어노테이션만 사용가능
@Target
- 어노테이션을 적용 할 위치 지정
- @Target({Element.위치1,Element위치2}) 형식으로 여러 위치 지정 가능
이름 | 위치 |
---|
package | 패키지 |
type | 타입 |
constructor | 생성자 |
field | 멤버변수 |
local_variable | 지역변수 |
parameter | 매개변수 |
type_parameter | 패러미터타입(제네릭타입 변수) |
type_use | 어떤 타입에도 적용 가능 |
@Retention
- 어노테이션이 적용/유지되는 범위 설정
- 디폴트 값은 @Retention(RetentionPolicy.CLASS)
이름 | 범위 |
---|
runtime | 컴파일 이후에도 사용 가능(로깅에 주로 사용) |
class | 클래스 참조 시까지 |
source | 컴파일 전까지. 컴파일 이후 소멸 |
@Inherited
- 작성 시, 어노테이션이 선언 된 클래스가 상속 될 때 함께 상속됨
@Documented
예제
- 어노테이션 정의
@Target({Element.TYPE, Element.FIELD})
@Retention(RetentionPolicy.runtime)
public @interface testAnnotation{}
- 사용
@testAnnotation
public class Example{
@testAnnotation
private static A = "student1";
@testAnnotation // 컴파일에러. field와 type에만 적용 가능
public Student(){}
}
참고
https://gmlwjd9405.github.io/2018/12/02/spring-annotation-types.html
https://velog.io/@gillog/Spring-Annotation-%EC%A0%95%EB%A6%AC
Interceptor
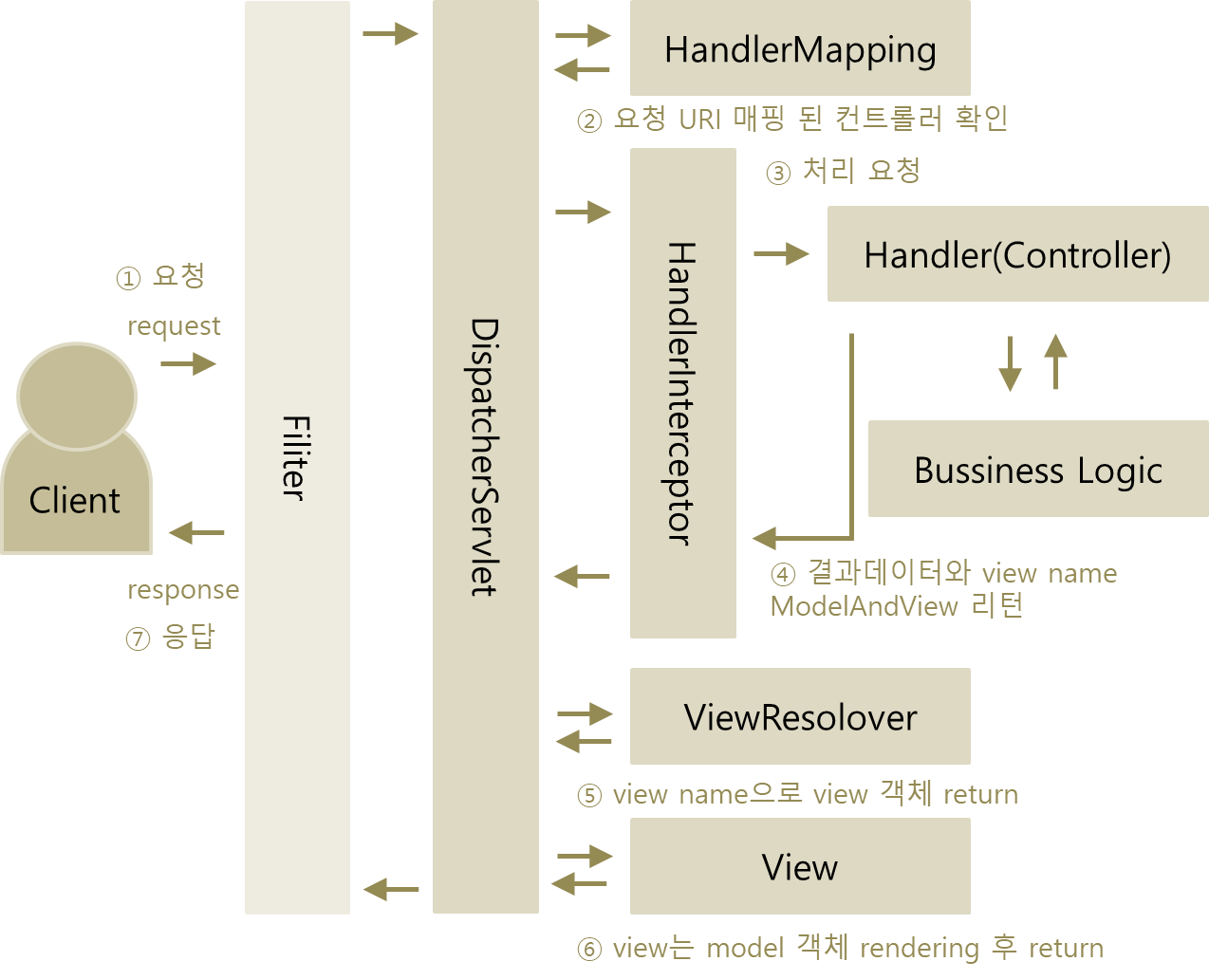
- 특정 uri 요청 시 컨트롤러에 들어오는 요청과 응답을 가로채서 처리
- 필터는 같은 어플리케이션 내에서만 접근이 가능하나 인터셉터는 스프링 내 모든 객체에 접근 가능
- 인터셉터를 이용하면 매번 확인용 코드를 입력하지 않아도 되므로 코드가 간단해짐
구현
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${org.springframework-version}</version>
</dependency>
- 인터셉터 생성
- HandlerInterceptor를 상속받아 메서드 오버라이딩
메서드 | 설명 |
---|
preHandle | 요청이 컨트롤러 도착하기 전에 실행 |
postHandle | 요청 처리 후 실행. 예외 발생시 실행안됨 |
afterCompletion | 컨트롤러에서 뷰를 통해 응답이 전송 된 후 실행 |
@Component
public class LoginCheckInterceptor implements HandlerInterceptor{
// 요청이 컨트롤러에 도착 전 실행됨
// 로그인여부 확인
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler)
throws Exception {
User user =(User) SessionUtils.getAttribute("LOGIN_USER");
if (user == null) {
request.setAttribute("error", "로그인 후 사용 가능한 서비스 입니다.");
request.getRequestDispatcher("/WEB-INF/jsp/loginForm").forward(request, response);
return false;
}
return true;
}
// 요청이 컨트롤러에서 처리 된 후 실행(view 전)
@Override
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler,
ModelAndView modelAndView) throws Exception {
HandlerInterceptor.super.postHandle(request, response, handler, modelAndView);
}
// 응답이 view로 전달 된 후 실행
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex)
throws Exception {
HandlerInterceptor.super.afterCompletion(request, response, handler, ex);
}
}