흐름
- VO 작성
- Mapper 작성
- Dao 작성
- Service 작성
- Controller 작성
- View(Jsp) 작성
VO
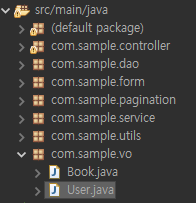
private int no;
private String id;
private String password;
private String name;
private String email;
private int point;
private String disabled;
private Date updatedDate;
private Date createdDate;
// + getter, setter
Mapper
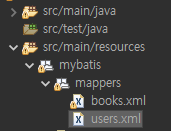
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.sample.dao.UserDao">
<resultMap id="UserResultMap" type="com.sample.vo.User">
<id column="no" property="no" />
<result column="user_id" property="id" />
<result column="user_password" property="password" />
<result column="user_name" property="name" />
<result column="user_email" property="email" />
<result column="user_point" property="point" />
<result column="user_disabled" property="disabled" />
<result column="user_updated_date" property="updatedDate" />
<result column="user_created_date" property="createdDate" />
</resultMap>
<select id="getUser" parameterType="map" resultMap="UserResultMap">
select
*
from
sample_spring_book_users
<choose>
<when test="no != null">
where user_no = #{no}
</when>
<when test="id != null">
where user_id = #{id}
</when>
<when test="email != null">
where user_email = #{email}
</when>
</choose>
</select>
<select id="getUserById" parameterType="string" resultMap="UserResultMap">
select
*
from
sample_spring_book_users
where
user_id = #{value}
</select>
ResultMap
- Mapper에서 중복되는 ResultMap을 사용할 때, 공통 ResultMap을 선언하여 함께 사용
DAO
@Mapper
public interface UserDao {
User getUser(Map<String, Object> param);
User getUserById(String id);
}
Service
@Service
public class UserService {
@Autowired
private UserDao userDao;
public User login(String id, String password) {
User user = userDao.getUserById(id);
if(user == null) {
throw new RuntimeException("회원정보가 존재하지 않습니다.");
}
if("Y".equals(user.getDisabled())) {
throw new RuntimeException("탈퇴처리된 회원아이디입니다.");
}
if(!password.equals(user.getPassword())) {
throw new RuntimeException("비밀번호가 일치하지 않습니다.");
}
return user;
}
}
Controller
public class HomeController {
@Autowired
UserService userService;
@GetMapping("/login.do")
public String loginform() {
return "loginform.jsp";
}
@PostMapping("/login.do")
public String login(String id, String password, Model model) {
if(!StringUtils.hasText(id) || !StringUtils.hasText(password)) {
model.addAttribute("error", "아이디와 비밀번호는 필수입력값입니다.");
return "loginform.jsp";
}
try {
User user = userService.login(id, password);
SessionUtils.addAttribute("LOGIN_USER", user);
return "redirect:home.do";
} catch(RuntimeException e) {
model.addAttribute("error",e.getMessage());
return "loginform.jsp";
}
}
}
View
<div class="container">
<div class="row mb-3">
<div class="col">
<h1>로그인 폼</h1>
</div>
</div>
<div class="row mb-3">
<div class="col-8">
<c:if test="${not empty error }">
<div class="mb-3 alert alert-danger">
<strong>오류</strong> ${error }
</div>
</c:if>
<form class="border p-3 bg-light" method="post" action="login.do">
<div class="mb-3">
<label class="form-label">아이디</label>
<input type="text" class="form-control" name="id" />
</div>
<div class="mb-3">
<label class="form-label">비밀번호</label>
<input type="password" class="form-control" name="password" />
</div>
<div class="mb-3 text-end">
<a href="home.do" class="btn btn-secondary">취소</a>
<button class="btn btn-primary">로그인</button>
</div>
</form>
</div>
</div>
</div>