Spring JDBC
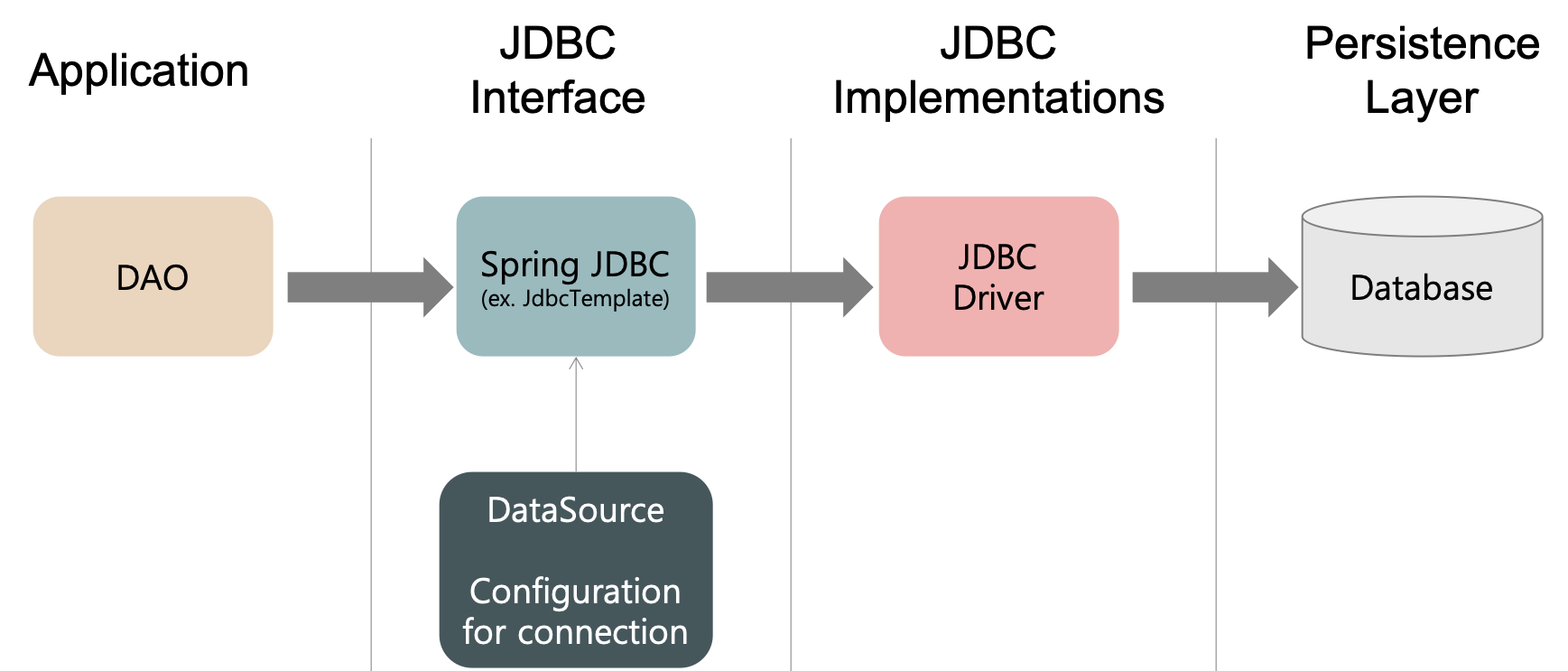
Connection Pool
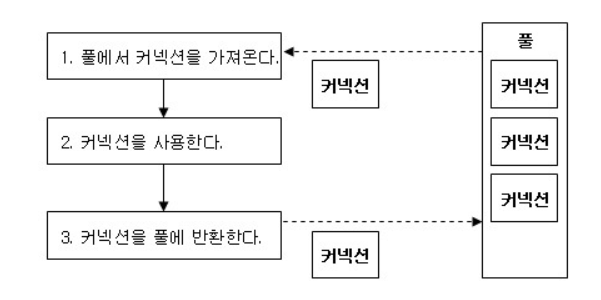
- DB와 연결한 객체들을 저장해두었다가 요청 시 꺼내서 사용하는 방식
- 모든 ConnectionPool 객체는 javax.sql.DataSource 인터페이스를 구현한 객체
- spring bean configuration file로 xml파일을 생성해서 관련 객체를 스프링 빈으로 등록
BasicDataSource
- commons-dbcp라이브러리의 Datasource 구현체
- DB접속을 위한 기본정보 제공
- jdbc의 드라이버클래스명, 접속URL, 사용자명, 비밀번호 입력
<bean id="basicDataSource" class="org.apache.commons.dbcp2.BasicDataSource">
<property name="driverClassName" value="oracle.jdbc.OracleDriver"></property>
<property name="url" value="jdbc:oracle:thin:@localhost:1521:xe"></property>
<property name="username" value="계정명"></property>
<property name="password" value="비밀번호"></property>
</bean>
JdbcTemplate
- spring-jdbc 라이브러리의 JdbcTemplate 클래스
- insert, update, delete,select 쿼리문을 실행하는 메서드 제공
- ConnectionPool을 주입받음
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="basicDataSource"></property>
</bean>
빈 등록
- db관련 작업이 구현된 클래스(DaoImpl)를 스프링 빈으로 등록
- SQL처리를 지원하는 JdbcTemplate를 주입받음
<bean id="daoImpl" class="com.sample.dao.DaoImpl">
<property name="template" ref="jdbcTemplate"></property>
</bean>
- 사용자 요청을 처리하는 서비스 클래스(ServiceImpl)를 스프링 빈으로 등록
- 관련 db작업을 지원하는 객체(DaoImpl)를 주입받아야함
<bean id="serviceImpl" class="com.sample.service.ServiceImpl">
<property name="dao" ref="daoImpl"></property>
</bean>
로직 구현
Dao interface
public interface EmployeeDao {
void insert(Employee employee);
Employee getEmployeeById(int employeeId);
List<Employee> getAllEmployees();
}
DaoImpl class
- Dao인터페이스를 상속받아 DB엑세스 작업을 구현한 클래스
public class EmployeeDaoImpl implements EmployeeDao {
// 쿼리를 처리하는 JdbcTemplate의 멤버변수와 setter 메서드 정의
private JdbcTemplate template;
public void setTemplate(JdbcTemplate template) {
this.template = template;
}
@Override
public void insert(Employee employee) {
String sql = "insert into employees "
+ "(employee_id, first_name, last_name) "
+ "values "
+ "(employees_seq.nextval, ?, ?)";
template.update(sql,
employee.getFirstName(),
employee.getLastName());
}
// 쿼리문 수행결과가 한 개
// 객체 그대로 반환
@Override
public Employee getEmployeeById(int employeeId) {
String sql = "select * from employees where employee_id = ?";
return template.queryForObject(sql, new EmployeeRowMapper(), employeeId);
}
// 쿼리문 수행결과가 한 개 이상
// List로 반환
@Override
public List<Employee> getAllEmployees() {
String sql = "select * from employees";
return template.query(sql, new EmployeeRowMapper());
}
// RowMapper 구현
public class EmployeeRowMapper implements RowMapper<Employee> {
@Override
public Employee mapRow(ResultSet rs, int rowNum) throws SQLException {
Employee emp = new Employee();
emp.setId(rs.getInt("employee_id"));
emp.setFirstName(rs.getString("first_name"));
emp.setLastName(rs.getString("last_name"));
return emp;
}
}
}
JdbcTemplate.query 메서드
JdbcTemplate.query(쿼리문[, 바인딩변수], RowMapper<Type>
- 쿼리문: 수행할 SQL문
- 바인딩변수: ? 값이 있다면 넣어줄 것
RowMapper<Type>
: 원하는 형태의 결과값을 반환해주는 인터페이스
Service interface
- 사용자 요청을 처리하는 서비스메서드가 정의된 인터페이스
예제를 위해 만든 메서드이므로 참고
public interface EmployeeService {
void addNewEmployee(Employee employee);
List<Employee> getAllEmployees();
Employee getEmployeeDetail(int employeeId);
}
ServiceImpl class
public class EmployeeServiceImpl implements EmployeeService {
// DB접근을 지원하는 클래스를 제공받아 사용
private EmployeeDao employeeDao;
public void setEmployeeDao(EmployeeDao employeeDao) {
this.employeeDao = employeeDao;
}
@Override
public void addNewEmployee(Employee employee) {
employeeDao.insert(employee);
}
@Override
public List<Employee> getAllEmployees() {
return employeeDao.getAllEmployees();
}
@Override
public Employee getEmployeeDetail(int employeeId) {
return employeeDao.getEmployeeById(employeeId);
}
}
어플리케이션
관련 메서드
resource
String resource = "classpath:/spring/context-jdbc.xml";
- Spring XML Configuration file 경로를 저장해 둔 문자열 객체
ApplicationContext
ApplicationContext ctx = new ClassPathXmlApplicationContext(resource);
- 빈 객체를 생성/관리하는 BeanFactory를 상속 받음
- Bean객체 생성/관리 및 트랜잭션, AOP처리 기능
ClassPathXmlApplicationContext(xml파일명)
- ClassPath에 위치한 xml 설정 파일을 읽어옴
ApplicationContext.getBean()
EmployeeService service = ctx.getBean(EmployeeService.class);
- EmployeeService클래스의 bean객체를 얻어옴
직원 조회
public class EmployeeSelectAllApplication {
public static void main(String[] args) {
String resource = "classpath:/spring/context-jdbc.xml";
ApplicationContext ctx = new ClassPathXmlApplicationContext(resource);
EmployeeService service = ctx.getBean(EmployeeService.class);
List<Employee> employeeList = service.getAllEmployees();
for (Employee emp : employeeList) {
System.out.println(emp.getId() + ", " + emp.getFirstName());
}
}
}