이터레이션 프로토콜
- 순회 가능한 데이터 컬렉션(자료구조)를 만들기 위해 미리 약속한 규칙
for...of, spread
- 기본자료 구조로는 Array, String, Map, Set
- 이터러블 프로토콜과 이터레이터 프로토콜 두가지가 존재
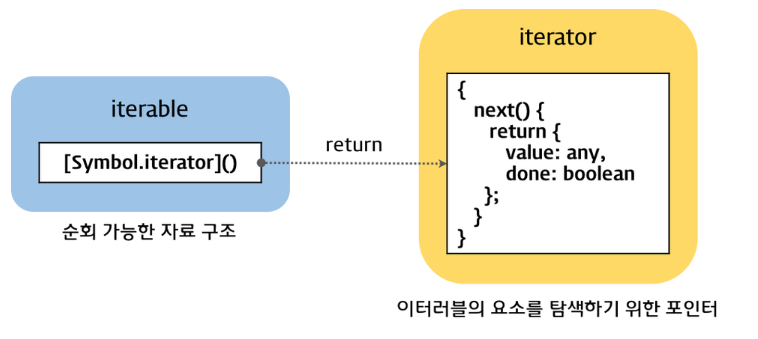
이터러블
[Symblo.iterator](): Iterator;
- 심볼정의를 가진 객체나, 특정한 함수가 Iterator를 리턴한다는 것은 순회 가능한 객체
- 순회가 가능하면 for..of, spread 연산자 사용가능
const array = [1, 2 ,3];
for(const item of array.entries()){
console.log(item);
}
const obj = { 0:1, 1:2};
for(const item of obj){
console.log(item);
}
이터레이터
- next()는 이터러블의 각 요소를 순회하기 위한 포인터의 역할을 한다. 호출하면 이터러블을 순차적으로 한 단계씩 순회하며 이터레이터 리절트 객체를 반환
- result.value : 현재 순회 중인 이터러블 값 반환
- result.done : 이터러블의 순회 완료 여부 반환
const array = [1, 2 ,3];
const iterator = array.values();
console.log(iterator.next().value);
console.log(iterator.next().value);
console.log(iterator.next().value);
console.log(iterator.next().done);
제너레이터 Generator
- 이터레이션 프로토콜을 준수한 방식보다 간편하게 이터러블 구현 가능
- 제너레이터 함수는 이터러블을 생성하는 함수
- yield 문 : next를 호출해야 순회 사용하는 사람에게 제어권을 양도한다.
function* multipleGenerator(){
for(let i = 0 ; i <10; i++){
yield i **2;
}
const multiple = multipleGenerator();
let next = multiple.next();
console.log(next.value, next.done);
스프레드 Spread
- Spread 연산자, 전개구문
- 모든 iterable은 Spread 될 수 있다
- 순회가 가능한 모든 것들은 촤르르륵 펼쳐 질 수 있다.
func(...iterable)
[...iterable]
{...obj}
function add(a, b, c){
return a + b + c;
}
const nums = [1, 2, 3]
console.log(add(...nums));
function sum(first, second, ...nums) {
console.log(nums);
}
sum(1, 2, 0, 1, 2, 4);
const fruits1 = ['🍏','🥝']
const fruits2 = ['🍓','🍌']
let arr = fruits1.concat(fruits2);
console.log(arr);
arr=[...fruits1, ...fruits2];
console.log(arr);
const ellie = {name: 'Ellie', age:20};
const updated= {
...ellie,
job: 's/w engineer',
};
console.log(ellie);
console.log(updated);
구조 분해 할당 Desturcturing Assignment
- 배열이나 객체의 속성을 해체하여 그 값을 개별 변수에 담을 수 있게 하는 표현식
- 데이터 뭉치(그룹화)를 쉽게 만들 수 있다
const fruits =['🍏','🥝','🍓','🍌'];
const [first, second, ...others] = fruits;
console.log(first);
function createEmoji(){
return ['apple','🍎'];
}
const [title, emoji] = createEmoji();
console.log(title);
console.log(emoji);
const ellie = {name: 'Ellie', age:20, job: 's/w engineer'};
function display({name, age, job}){
console.log('이름', 'name');
console.log('나이', 'age');
console.log('직업', 'job');
}
display(ellie);
const {name, age, job: occupation, pet = '강아지' } = ellie;
console.log(name);
console.log(age);
console.log(job);
console.log(pet);