Part 5. 메인 구조
1) MainPage.jsx
구성
코드
- Mainpage
import { ScrollView } from "native-base";
import CardItem from "../components/CardItem";
const MainPage = () => {
return (
<ScrollView>
<CardItem />
<CardItem />
<CardItem />
</ScrollView>
);
};
export default MainPage;
- CardItem
import {
Box,
Image,
Text,
AspectRatio,
Center,
Stack,
HStack,
Heading,
} from "native-base";
const CardItem = () => {
return (
<Box alignItems="center">
<Box
margin={3}
maxW="100%"
rounded="lg"
overflow="hidden"
borderColor="coolGray.200"
borderWidth="1"
>
<Box>
<AspectRatio w="100%" ratio={16 / 9}>
<Image
source={{
uri: "https://www.holidify.com/images/cmsuploads/compressed/Bangalore_citycover_20190613234056.jpg",
}}
alt="image"
/>
</AspectRatio>
</Box>
<Stack p="4" space={3}>
<Stack space={2}>
<Heading size="md" ml="-1">
The Garden City
</Heading>
<Text
fontSize="xs"
fontWeight="500"
ml="-0.5"
mt="-1">
The Silicon Valley of India.
</Text>
</Stack>
<Text fontWeight="400">
Bengaluru (also called Bangalore) is the center of India's high-tech
industry. The city is also known for its parks and nightlife.
</Text>
<HStack alignItems="center" space={4} justifyContent="space-between">
<HStack alignItems="center">
<Text
color="coolGray.600"
fontWeight="400">
6 mins ago
</Text>
</HStack>
</HStack>
</Stack>
</Box>
</Box>
);
};
export default CardItem;
구현 결과
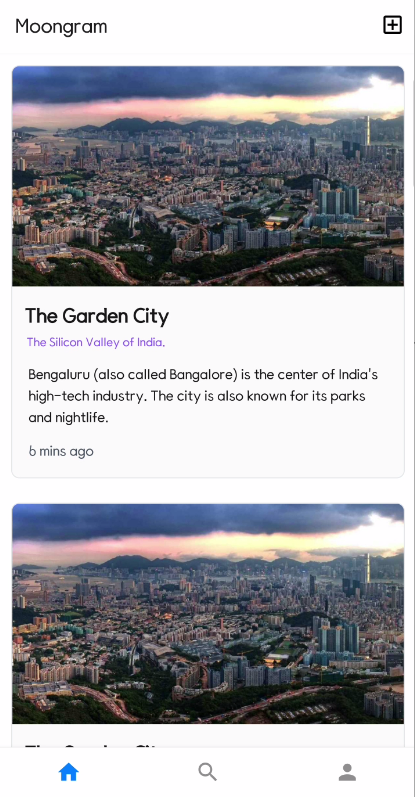
2) SearchPage.jsx
구성
코드
import { StyleSheet } from "react-native";
import { SafeAreaView } from "react-native-safe-area-context";
import {
Box,
Center,
Input,
Icon,
Divider,
HStack,
VStack,
ScrollView,
Image,
FlatList,
} from "native-base";
import { MaterialIcons } from "@expo/vector-icons";
import ImageItem from "../components/ImageItem";
const data = require("../data.json");
const SearchPage = () => {
const SearchBar = () => {
return (
<VStack>
<Center>
<Input
mx="3"
w="90%"
mt="3"
variant="rounded"
placeholder="검색"
InputLeftElement={
<Icon
as={<MaterialIcons name="search" />}
size={5}
ml="2"
color="muted.400"
/>
}
/>
</Center>
<Divider mt={2} />
</VStack>
);
};
return (
<SafeAreaView>
<FlatList
ListHeaderComponent={() => {
return <SearchBar />;
}}
numColumns={3}
data={data.diary}
renderItem={({ item }) => {
return <ImageItem image={item.image} key={item.id}></ImageItem>;
}}
/>
</SafeAreaView>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "white",
},
});
export default SearchPage;
구현 결과
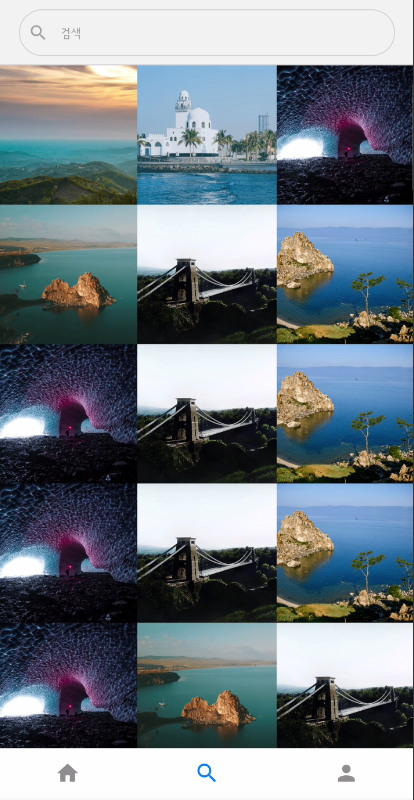
3) MyPage.jsx
구성
- 로그인한 정보, 작성한 게시글 수, 작성한 게시글 리스트
코드
import { StyleSheet } from "react-native";
import {
Box,
Button,
FlatList,
HStack,
Avatar,
Text,
VStack,
Center,
} from "native-base";
import ImageItem from "../components/ImageItem";
const data = require("../data.json");
const MyPage = () => {
const MyPageHeader = () => {
return (
<VStack>
<Box>
<HStack space={5}>
{}
<Avatar
margin={5}
alignSelf="center"
size="lg"
bg="indigo.500"
source={{
uri: "https://images.unsplash.com/photo-1614289371518-722f2615943d?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=687&q=80",
}}>
프로필 이미지
</Avatar>
<Center>
<VStack alignItems="center">
<Text>0</Text>
<Text>게시물</Text>
</VStack>
</Center>
<Center>
<VStack alignItems="center">
<Text>0</Text>
<Text>좋아요</Text>
</VStack>
</Center>
</HStack>
</Box>
<Box h="20" pl={5}>
<Text>안녕하세요.</Text>
</Box>
<Box h="20" p={5}>
<Button>프로필 편집</Button>
</Box>
</VStack>
);
};
return (
<FlatList
style={styles.container}
numColumns={3}
data={data.diary}
ListHeaderComponent={() => {
return <MyPageHeader />;
}}
renderItem={({ item }) => {
return <ImageItem image={item.image} key={item.id}></ImageItem>;
}}></FlatList>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "white",
},
});
export default MyPage;
구현 결과
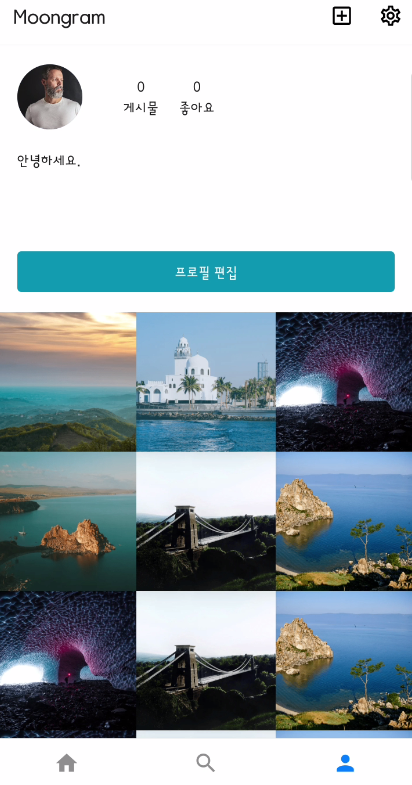