1. 강서구 민원상담 데이터 시각화
강서구민의 민원의 제목 데이터를 워드클라우드로
시각화해 구민들에게 중요한 이슈가 무엇인지 분석
2. 코드
import pandas as pd
from konlpy.tag import Okt
from collections import Counter
from wordcloud import WordCloud, STOPWORDS
import matplotlib.pyplot as plt
import matplotlib as mp
df = pd.read_csv('complain_title1.csv', encoding='cp949')
df = df.drop(['Unnamed: 0'], axis=1)
df.head(2)
|
title |
0 |
주차구획선 |
1 |
코로나 확진 격리 통지문자 |
script = df['title']
script.head(2)
0 주차구획선
1 코로나 확진 격리 통지문자
Name: title, dtype: object
script.to_csv('word.txt', encoding='utf-8-sig')
text = open('word.txt', encoding='utf-8-sig').read()
spwords=set(STOPWORDS)
def token_konlpy(text):
okt=Okt()
return [word for word in okt.nouns(text) if len(word)>1]
noun = token_konlpy(text)
len(noun)
34560
noun_set = set(noun)
len(noun_set)
4447
count = Counter(noun)
count.pop('강서구')
count.pop('강서')
count.pop('문의')
count.pop('신고')
count.pop('관련')
count.pop('불법')
count.pop('구청')
count.pop('요청')
count.pop('처리')
count.pop('민원')
count.pop('대한')
count.pop('문제')
190
word = dict(count.most_common(15))
wc = WordCloud(max_font_size=200,
font_path = 'C:\Windows\Fonts\malgun.ttf',
stopwords=spwords, background_color="white",
width=2000, height=500).generate_from_frequencies(word)
plt.figure(figsize = (40,40))
plt.imshow(wc)
plt.tight_layout(pad=0)
plt.axis('off')
plt.show()
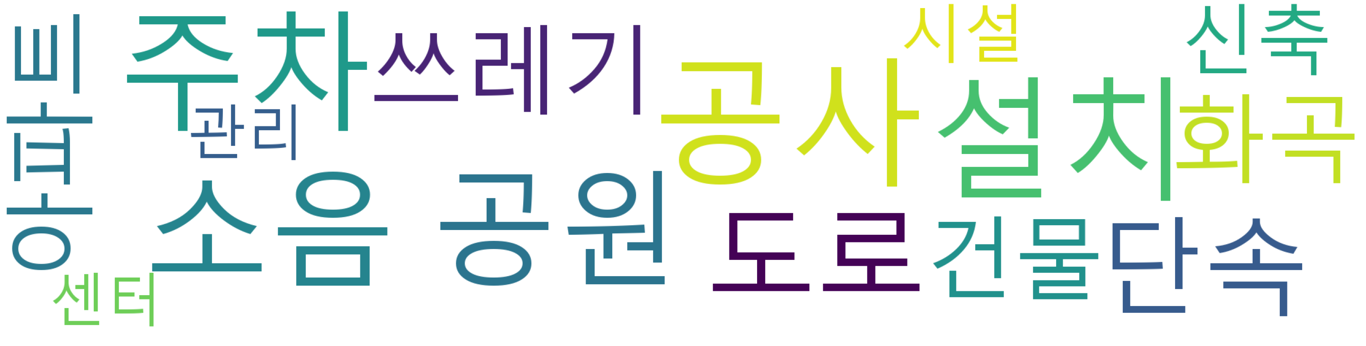
from matplotlib import font_manager, rc
def showGraph(wordInfo):
font_location = 'C:\Windows\Fonts\HANDotumB.ttf'
font_name = font_manager.FontProperties(fname=font_location).get_name()
plt.xlabel('주요 단어',fontsize=20)
plt.ylabel('빈도수', fontsize=20)
plt.grid(True)
Sorted_Dict_Values = sorted(wordInfo.values(), reverse=True)
Sorted_Dict_Keys = sorted(wordInfo, key=wordInfo.get, reverse=True)
plt.bar(range(len(wordInfo)), Sorted_Dict_Values, align='center')
plt.xticks(range(len(wordInfo)), list(Sorted_Dict_Keys), rotation='70')
plt.rcParams["figure.figsize"] = (50,30)
plt.show()
word2= dict(count.most_common(10))
word2
{'공사': 489,
'설치': 455,
'주차': 429,
'소음': 419,
'공원': 408,
'도로': 313,
'단속': 285,
'쓰레기': 234,
'화곡': 226,
'아파트': 206}