이번 글은
- 구축된 데이터들을 연계하여 분석하는데 필요한 토지 형상 데이터(연속지정도형정보)를 업로드합니다.
- 데이터 출처 : 국가공간정보포털 오픈API 中 연속지적도형정보
- 코드 파일 첨부(github)
소스 데이터 읽기
import geopandas as gpd
lot_polygon = gpd.read_file(
'D:/2022 공간빅데이터 경진대회/연속지적도형정보/AL_11_D002_20220903.zip!AL_11_D002_20220903.shp',
encoding='CP949'
)
lot_polygon
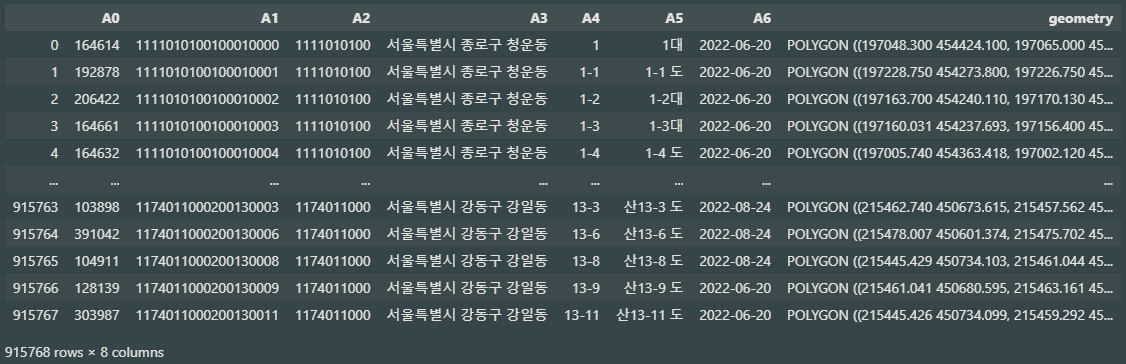
컬럼 확인
- A0 : 일련번호
- A1 : PNU(토지고유번호), ID
- A2 : 지역코드, PNU 앞 10자리와 동일
- A3 : 지역이름
- A4 : 지번
- A5 : 대지/산 여부 + 지번 + 지목(한 글자)
- A6 : 생성일
- geometry : 도형정보
활용 컬럼 선별
- no : A0
- pnu : A1
- address : A2 + 대지/산 여부 + A4
- jimok : A5의 지목
- created_at : A6
- geometry : geometry
데이터 편집
주소 컬럼 만들기
lot_polygon['address'] = lot_polygon.A3 + [
' 산 ' if pnu[10] == '2'
else ' '
for pnu
in lot_polygon.A1
] + lot_polygon.A4
지목 컬럼 만들기
import re
lot_polygon['jimok'] = [
txt[-1] if re.match('[가-힣]', txt[-1])
else None
for txt
in lot_polygon.A5
]
좌표계 변환(to EPSG:5179)
lot_polygon.crs
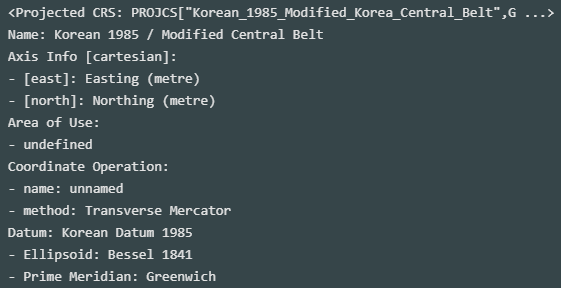
lot_polygon = lot_polygon.to_crs(5179)
lot_polygon.crs
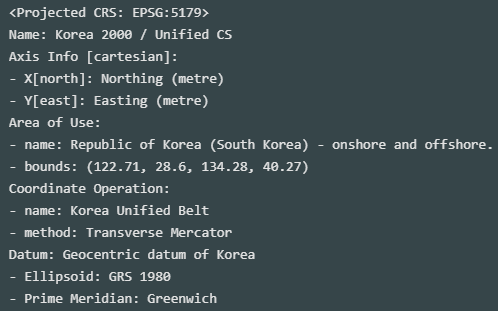
컬럼 정리
lot_polygon = lot_polygon[['A0', 'A1', 'address', 'jimok', 'A6', 'geometry']]
lot_polygon.columns = ['no', 'pnu', 'address', 'jimok', 'created_at', 'geometry']
테이블 스키마 설정
create table lot_polygon (
no int,
pnu varchar(19) primary key,
address varchar,
jimok varchar(1),
created_at date,
geometry public.geometry(geometry, 5179),
uploaded_at timestamp default current_timestamp
);
create index index_lot_polygon_on_pnu on lot_polygon (pnu);
create index index_lot_polygon_on_geometry on lot_polygon using GIST(geometry);
DB 연결
import psycopg2
from sqlalchemy import create_engine
conn = psycopg2.connect(
'host=localhost port=5432 dbname=postgres user=postgres password=postgres',
options='-c search_path=sbd'
)
conn.set_session(autocommit=True)
cur = conn.cursor()
gconn = create_engine(
'postgresql://postgres:postgres@localhost:5432/postgres'
)
테이블 생성
cur.execute(
f'''
select count(*)
from information_schema.tables
where
table_name ~ 'lot_polyton' and
table_schema = 'sbd'
'''
)
if not cur.fetchone()[0]:
cur.execute(
open('./sql/sbd-create_table_lot_polygon.sql').read()
)
cur.execute(
'delete from lot_polygon'
)
lot_polygon.to_postgis(
'lot_polygon',
gconn,
'sbd',
if_exists='append',
chunksize=100_000
)
업로드 데이터 확인
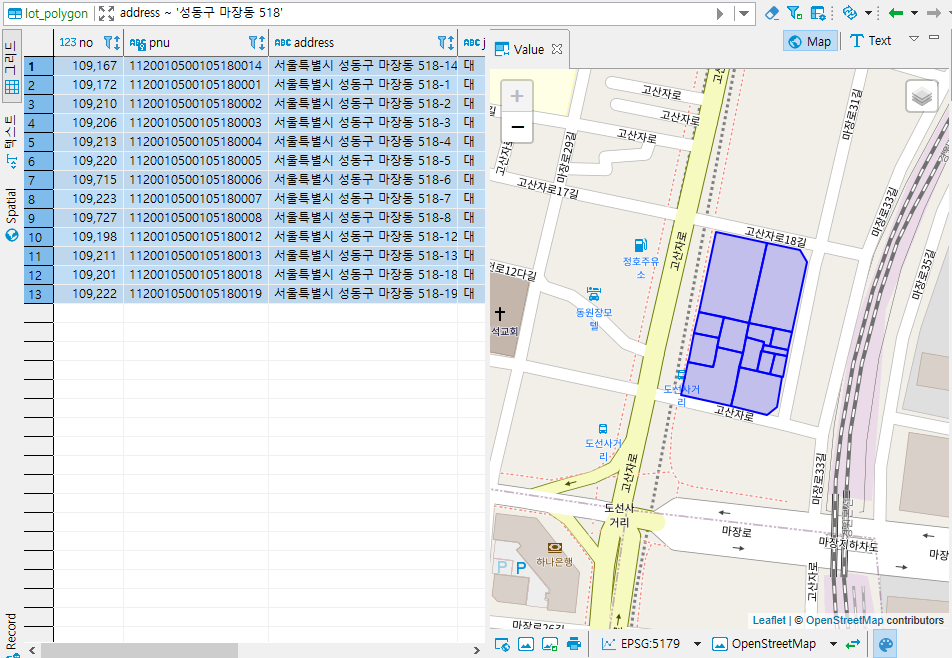