1. 코드 분석
1. tlpi-dist/pmsg/pmsg_create.c
1. 코드
#include <mqueue.h>
#include <sys/stat.h>
#include <fcntl.h>
#include "tlpi_hdr.h"
static void
usageError(const char *progName)
{
fprintf(stderr, "Usage: %s [-cx] [-m maxmsg] [-s msgsize] mq-name "
"[octal-perms]\n", progName);
fprintf(stderr, " -c Create queue (O_CREAT)\n");
fprintf(stderr, " -m maxmsg Set maximum # of messages\n");
fprintf(stderr, " -s msgsize Set maximum message size\n");
fprintf(stderr, " -x Create exclusively (O_EXCL)\n");
exit(EXIT_FAILURE);
}
int
main(int argc, char *argv[])
{
int flags, opt;
mode_t perms;
mqd_t mqd;
struct mq_attr attr, *attrp;
attrp = NULL;
attr.mq_maxmsg = 10;
attr.mq_msgsize = 2048;
flags = O_RDWR;
while ((opt = getopt(argc, argv, "cm:s:x")) != -1) {
switch (opt) {
case 'c':
flags |= O_CREAT;
break;
case 'm':
attr.mq_maxmsg = atoi(optarg);
attrp = &attr;
break;
case 's':
attr.mq_msgsize = atoi(optarg);
attrp = &attr;
break;
case 'x':
flags |= O_EXCL;
break;
default:
usageError(argv[0]);
}
}
if (optind >= argc)
usageError(argv[0]);
perms = (argc <= optind + 1) ? (S_IRUSR | S_IWUSR) :
getInt(argv[optind + 1], GN_BASE_8, "octal-perms");
mqd = mq_open(argv[optind], flags, perms, attrp);
if (mqd == (mqd_t) -1)
errExit("mq_open");
exit(EXIT_SUCCESS);
}
2. 동작 모습

3. 디버깅 및 분석
2. tlpi-dist/pmsg/pmsg_send.c
1. 코드
#include <mqueue.h>
#include <fcntl.h>
#include "tlpi_hdr.h"
static void
usageError(const char *progName)
{
fprintf(stderr, "Usage: %s [-n] mq-name msg [prio]\n", progName);
fprintf(stderr, " -n Use O_NONBLOCK flag\n");
exit(EXIT_FAILURE);
}
int
main(int argc, char *argv[])
{
int flags, opt;
mqd_t mqd;
unsigned int prio;
flags = O_WRONLY;
while ((opt = getopt(argc, argv, "n")) != -1) {
switch (opt) {
case 'n': flags |= O_NONBLOCK; break;
default: usageError(argv[0]);
}
}
if (optind + 1 >= argc)
usageError(argv[0]);
mqd = mq_open(argv[optind], flags);
if (mqd == (mqd_t) -1)
errExit("mq_open");
prio = (argc > optind + 2) ? atoi(argv[optind + 2]) : 0;
if (mq_send(mqd, argv[optind + 1], strlen(argv[optind + 1]), prio) == -1)
errExit("mq_send");
exit(EXIT_SUCCESS);
}
2. 동작 모습
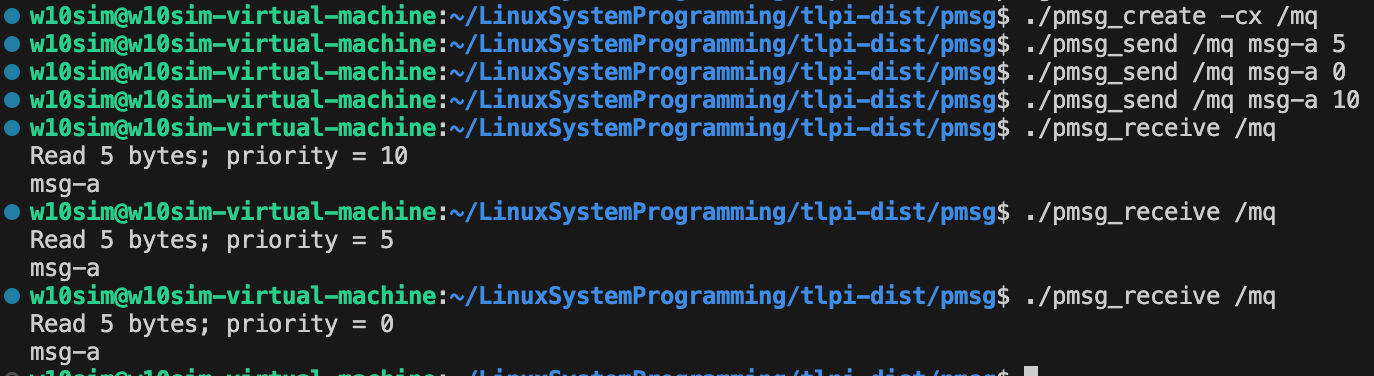
3. 디버깅 및 분석
3. tlpi-dist/pmsg/pmsg_receive.c
1. 코드
#include <mqueue.h>
#include <fcntl.h>
#include "tlpi_hdr.h"
static void
usageError(const char *progName)
{
fprintf(stderr, "Usage: %s [-n] mq-name\n", progName);
fprintf(stderr, " -n Use O_NONBLOCK flag\n");
exit(EXIT_FAILURE);
}
int
main(int argc, char *argv[])
{
int flags, opt;
mqd_t mqd;
unsigned int prio;
void *buffer;
struct mq_attr attr;
ssize_t numRead;
flags = O_RDONLY;
while ((opt = getopt(argc, argv, "n")) != -1) {
switch (opt) {
case 'n': flags |= O_NONBLOCK; break;
default: usageError(argv[0]);
}
}
if (optind >= argc)
usageError(argv[0]);
mqd = mq_open(argv[optind], flags);
if (mqd == (mqd_t) -1)
errExit("mq_open");
if (mq_getattr(mqd, &attr) == -1)
errExit("mq_getattr");
buffer = malloc(attr.mq_msgsize);
if (buffer == NULL)
errExit("malloc");
numRead = mq_receive(mqd, buffer, attr.mq_msgsize, &prio);
if (numRead == -1)
errExit("mq_receive");
printf("Read %ld bytes; priority = %u\n", (long) numRead, prio);
if (write(STDOUT_FILENO, buffer, numRead) == -1)
errExit("write");
write(STDOUT_FILENO, "\n", 1);
exit(EXIT_SUCCESS);
}
2. 동작 모습
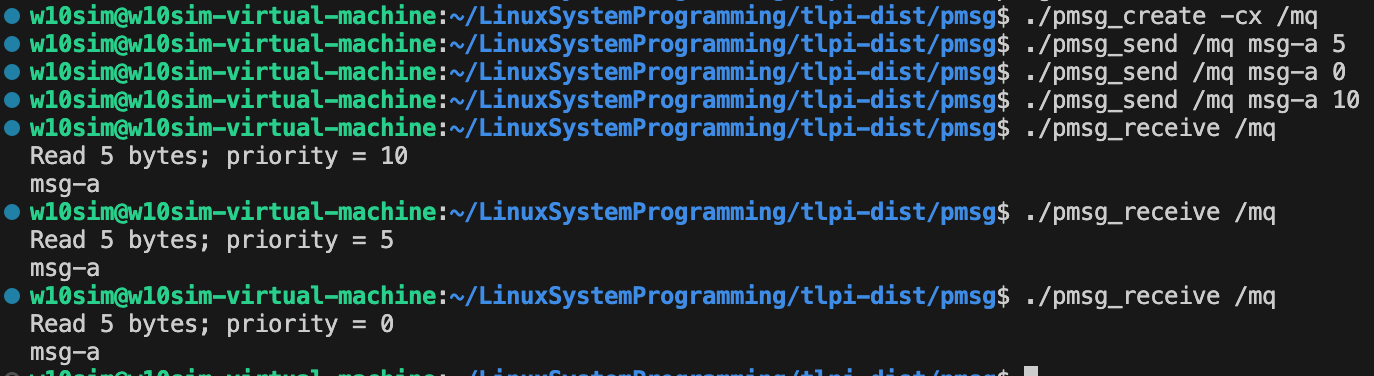
3. 디버깅 및 분석