1. 코드 분석
1. 토이 프로젝트
1. camera_HAL.cpp
1. 코드
#include <stdio.h>
#include <stdlib.h>
#include <string>
#include <errno.h>
#include <iostream>
#include <cstdio>
#include <unistd.h>
#include "camera_HAL.h"
#include "ControlThread.h"
static ControlThread *control_thread;
using std::cout;
using std::endl;
int toy_camera_open(void)
{
cout << "toy_camera_open" << endl;
control_thread = new ControlThread();
if (control_thread == NULL) {
cout << "Memory allocation error!" << endl;
return -ENOMEM;
}
return 0;
}
int toy_camera_take_picture(void)
{
return control_thread->takePicture();
}
2. 분석
2. camera_HAL.h
1. 코드
#ifndef _CAMERA_HAL_H_
#define _CAMERA_HAL_H_
#ifdef __cplusplus
extern "C" {
#endif
#include <stdint.h>
int toy_camera_open(void);
int toy_camera_take_picture(void);
#ifdef __cplusplus
}
#endif
#endif
2. 분석
3. ControlThread.cpp
1. 코드
#include <iostream>
#include <cstdio>
#include <unistd.h>
#include "ControlThread.h"
using std::cout;
using std::endl;
ControlThread::ControlThread()
{
cout << "C++ 연동: 여기는 C++ 영역 입니다" << endl;
}
ControlThread::~ControlThread()
{
cout << "C++ 연동: 소멸자입니다." << endl;
}
int ControlThread::takePicture()
{
cout << "C++ 연동: 사진을 캡쳐합니다." << endl;
return 0;
}
2. 분석
4. ControlThread.h
1. 코드
#ifndef _CONTROL_THREAD_H_
#define _CONTROL_THREAD_H_
class ControlThread {
public:
ControlThread();
~ControlThread();
public:
int takePicture();
private:
};
#endif
2. 분석
2. tlpi-dist/threads/thread_cancel.c
1. 코드
#include <pthread.h>
#include "tlpi_hdr.h"
static void *
threadFunc(void *arg)
{
int j;
printf("New thread started\n");
for (j = 1; ; j++) {
printf("Loop %d\n", j);
sleep(1);
}
return NULL;
}
int
main(int argc, char *argv[])
{
pthread_t thr;
int s;
void *res;
s = pthread_create(&thr, NULL, threadFunc, NULL);
if (s != 0)
errExitEN(s, "pthread_create");
sleep(3);
s = pthread_cancel(thr);
if (s != 0)
errExitEN(s, "pthread_cancel");
s = pthread_join(thr, &res);
if (s != 0)
errExitEN(s, "pthread_join");
if (res == PTHREAD_CANCELED)
printf("Thread was canceled\n");
else
printf("Thread was not canceled (should not happen!)\n");
exit(EXIT_SUCCESS);
}
2. 동작 모습

3. 디버깅 및 분석
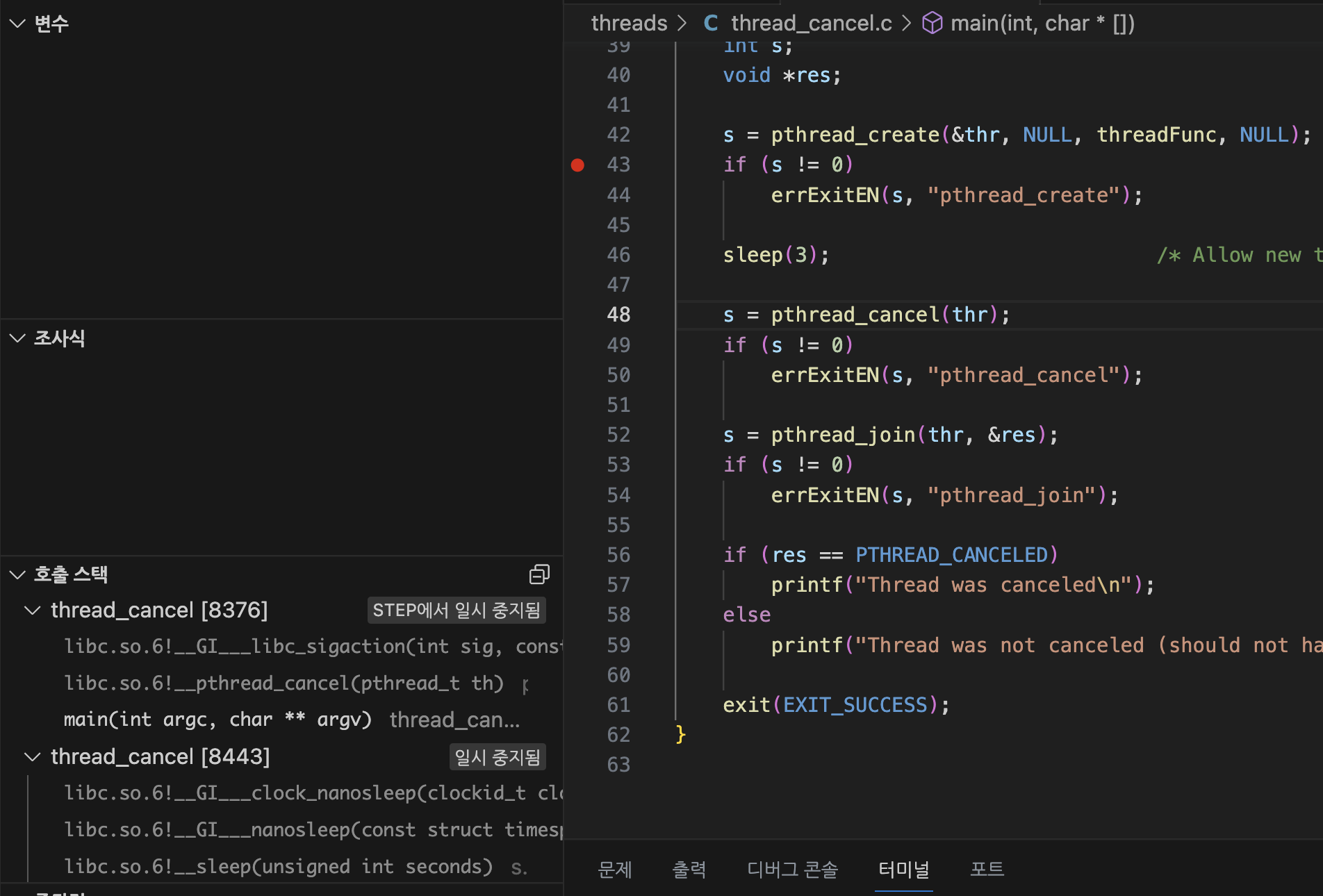