String 메소드
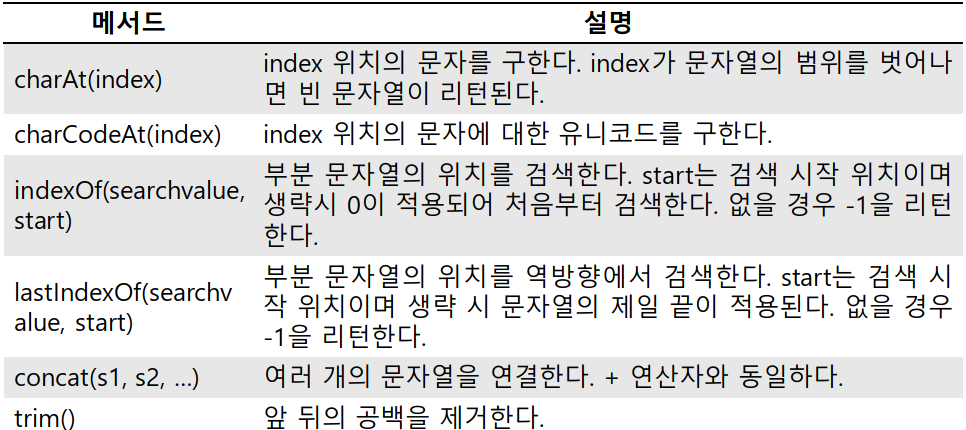
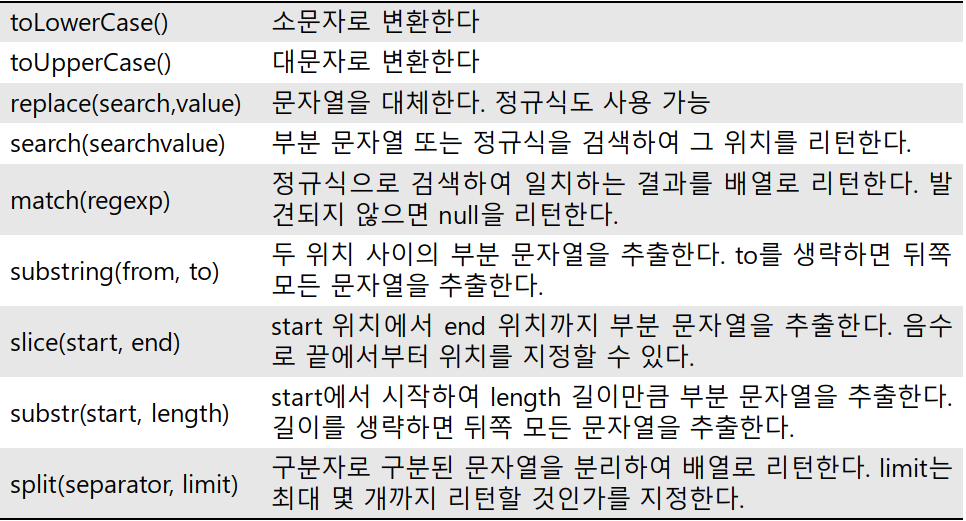
charAt(index)
charCodeAt(index)
- 지정된 index 위치의 문자 코드를 리턴한다.
substring(start, end)
slice(start, end)
substr(start, length)
split(구분자, 갯수)
- 구분자를 기준으로 문자열을 분리하여 배열로 생성되어 저장한다.
갯수는 생략가능하다. 생략시 전체를 대상으로 한다.
replace(oldvalue, newvalue)
- oldvalue를 newvalue로 변경한다.
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="../css/mystyle.css" type="text/css">
<script type="text/javascript">
str = "korea 우리 나라 좋은 나라 대한 민국 Korea";
function proc1(){
a = str.charAt(4);
codea = str.charCodeAt(4);
res = str + "<br>";
res += "srt.charAt(4): "+a+"<br>";
res += "str.charCodeAt(4): "+codea+"<br>";
document.getElementById('result1').innerHTML = res;
}
function proc2(){
subs = str.substring(3, 7);
slic = str.slice(3, 7);
sublen = str.substr(3, 5);
res = str + "<br>";
res += "str.substring(3, 7): "+ subs + "<br>";
res += "str.slice(3, 7): "+ slic+ "<br>";
res += "str.subststr(3, 7): "+ sublen+ "<br>";
document.getElementById('result2').innerHTML = res;
}
function proc3(){
arr = str.split(" ");
document.getElementById('result3').innerHTML = arr;
}
function proc4(){
area = document.getElementsByTagName('textarea')[0].value;
reparea = area.replace(/\r/g,"").replace(/\n/g, "<br>");
aa = str.replace(/korea/gi,'꾞릮아');
alert(aa);
document.getElementById('result4').innerHTML = reparea;
}
function proc5(){
input = prompt("찾는 문자열을 입력하세요");
value1 = str.match(/korea/gi);
console.log(value1);
value2 = str.match(/Good/gi);
console.log(value2);
document.getElementById('result5').innerHTML = "str.match(/korea/gi) <br>";
document.getElementById('result5').innerHTML += "배열을 리턴한다:" + value1 + "<br>";
document.getElementById('result5').innerHTML += "str.match(/Good/gi) <br>";
document.getElementById('result5').innerHTML += "null을 리턴한다:"+value2;
}
</script>
</head>
<body>
<div class="box">
charAt(index): 지정된 index 위치의 문자를 리턴한다.<br>
charCodeAt(index): 지정된 index 위치의 문자 코드를 리턴한다.<br>
<br>
<button type="button" onclick="proc1()">확인</button>
<div id="result1"></div>
</div>
<div class="box">
문자열 추출<br>
substring(start, end): start ~ end 사이의 문자열 추출<br>
slice(start, end): start ~ end 사이의 문자열 추출<br>
substr(start, length): start부터 length갯수만큼 추출<br>
<br>
<button type="button" onclick="proc2()">확인</button>
<div id="result2"></div>
</div>
<div class="box">
문자열 분리<br>
split(구분자, 갯수): 구분자를 기준으로 문자열을 분리하여 배열로 생성되어 저장한다.<br>
갯수는 생략가능하다. 생략시 전체를 대상으로 한다.<br>
<br>
<button type="button" onclick="proc3()">확인</button>
<div id="result3"></div>
</div>
<div class="box">
문자열 대체- 수정<br>
replace(oldvalue, newvalue): oldvalue를 newvalue로 변경한다.<br>
textarea에 여러줄로 입력된 문자를 script로 보낸다. - value <br>
id가 result4번인 요소에 출력한다. <br>
출력시 \r\n을 <br> 태그로 변경하여 출력한다.<br><br>
<textarea rows="5" cols="50"></textarea>
<button type="button" onclick="proc4()">확인</button>
<div id="result4"></div>
</div>
<div class="box">
문자열 검색<br>
match(value):value를 검색한다.<br>
검색된 문자열을 배열로리턴하고 검색문자가 없으면 null를 리턴한다. <br>
id가 result4번인 요소에 출력한다. <br>
출력시 \r\n을 <br> 태그로 변경하여 출력한다.<br><br>
<button type="button" onclick="proc5()">확인</button>
<div id="result5"></div>
</div>
</body>
</html>
주민등록번호를 입력 받아 생년월일과 성별을 출력하는 프로그램
주민번호 유효성 검사 예제
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link rel="stylesheet" href="../css/mystyle.css" type="text/css">
<script type="text/javascript">
function proc1(){
today = new Date();
num = prompt("주민번호를 입력하세요.", "-없이 입력");
month = num.substring(2, 4);
day = num.substring(4, 6);
if(num.substr(6, 1) == 1 || num.substr(6, 1) == 2){
year = "19"+num.substring(0, 2);
}
else{
year = "20"+num.substring(0, 2);
}
if(num.substr(6, 1)==1 || num.substr(6, 1)==3){
gender = "남자"
}else{
gender = "여자"
}
today.getFullYear()
age = (today.getFullYear()-year)+1;
str = "생일: " + year+"년"+ month+"월"+ day+ "일"+"<br>";
str += "성별: "+gender+"<br>";
str += "나이:"+age;
document.getElementById('result1').innerHTML = str;
}
function proc2(){
code = prompt("주민번호 입력", '-없이 입력');
sum = 0;
for(i=0; i<code.length-1; i++){
sum += code.substr(i,1) * (i % 8 + 2);
}
res = 11 - sum % 11
res = res % 10;
if(res == code.substr(code.length-1,1)){
alert("옳바른 주민번호다.");
}else{
alert("틀린 주민번호다.");
}
}
</script>
</head>
<body>
<div class="box">
주민등록번호를 입력 받아 ( -없이 입력 ) 생년월일과 성별을 출력하는 프로그램을 작성하시오.(입력은 prompt로 입력받는다.)<br>
예) 주민등록번호를 110326-4(1,2 - 1900/ 3,4-2000 ,1,3남자)432618로 입력 받은 경우<br>
생일 : 2011년 3월 26일<br>
성별 : 여자 나이: <br>
<br>
<button type="button" onclick="proc1()">확인</button>
<div id="result1"></div>
</div>
<div class="box">
주민등록번호를 입력 받아 주민등록번호의 유효성을 검사하는 프로그램을 작성하시오.(ABCDEF-GHIJKLM)<br>
1. A*2 + B*3 + ... + H*9 + I*2 + ... + L*5 의 총합을 구한다.<br>
110326-4432618<br>
2. 1번의 합을 11로 나눈 나머지를 구한다.<br>
3. 11에서 2번의 결과를 뺀다.<br>
4. 3번의 결과가 0~9이면 값 그대로, 10이면 0, 11이면 1로 변환<br>
5. 4번의 결과와 M자리의 값이 같으면 맞는 번호이다.<br>
<br>
<button type="button" onclick="proc2()">확인</button>
<div id="result2"></div>
</div>
</body>
</html>