통합테스트
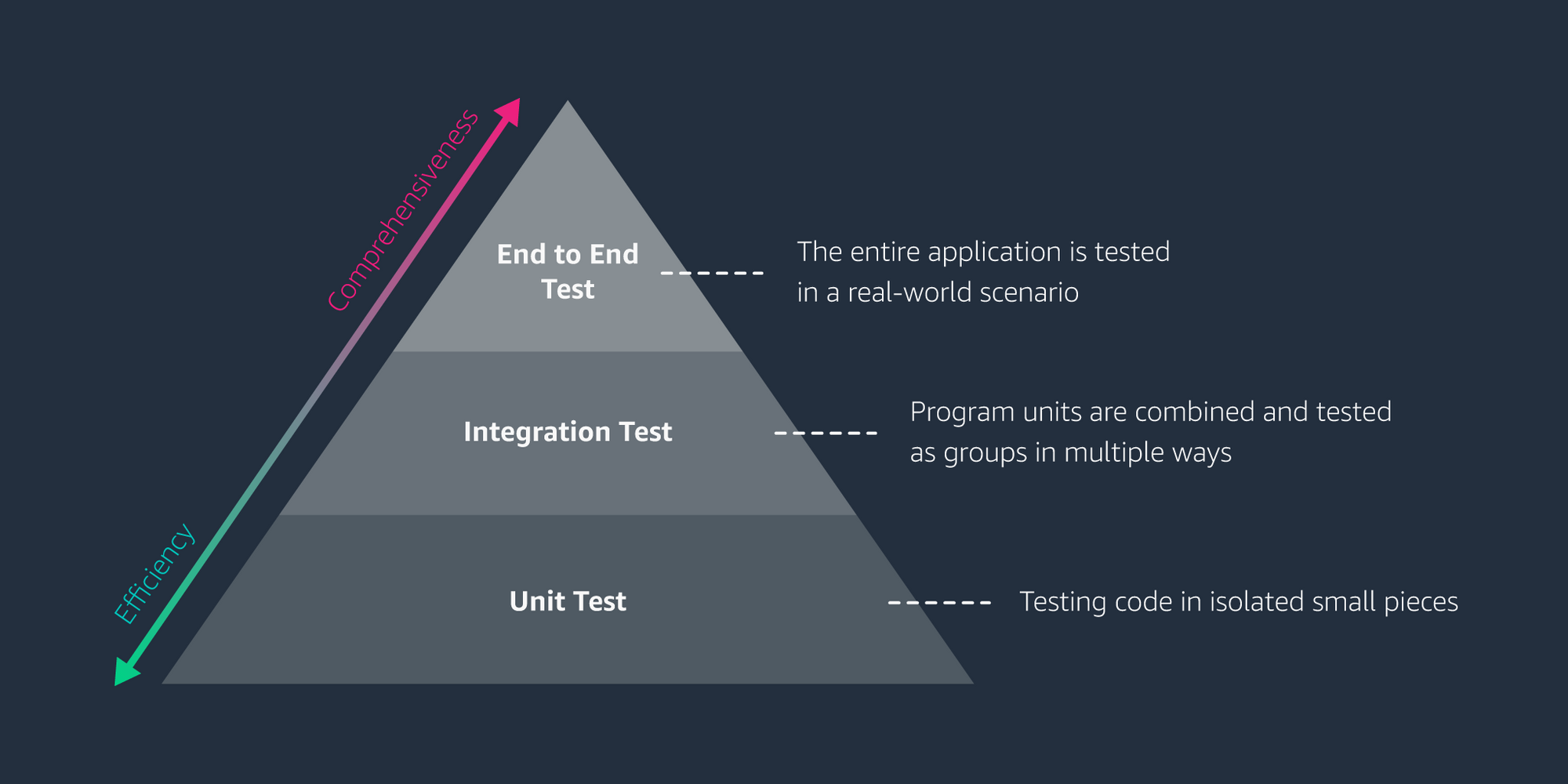
- 단위 테스트 (Unit Test)
- 하나의 모듈이나 클래스에 대해 세밀한 부분까지 테스트가 가능하다.
- 하지만 모듈 간에 상호 작용 검증은 할 수 없다.
- 통합 테스트 (Integration Test)
- 두 개 이상의 모듈이 연결된 상태를 테스트할 수 있다.
- 모듈 간의 연결에서 발생하는 에러 검증 가능하다.
Spring Boot를 이용한 통합 테스트 구현
- 통합 테스트
- 여러 단위 테스트를 하나의 통합된 테스트로 수행한다.
- 단위 테스트 시 Spring은 동작되지 않는다.
@SpringBootTest
- 스프링이 동작되도록 해주는 애너테이션
- 테스트 수행 시 스프링이 동작한다.
- Spring IoC/DI 기능을 사용 가능하다.
- Repository를 사용해 DB CRUD가 가능하다.
@SpringBootTest(webEnvironment = SpringBootTest.WebEnvironment.RANDOM_PORT)
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
@TestMethodOrder(MethodOrderer.OrderAnnotation.class)
class ProductServiceIntegrationTest {
@Autowired
ProductService productService;
@Autowired
UserRepository userRepository;
User user;
ProductResponseDto createdProduct = null;
int updatedMyPrice = -1;
@Test
@Order(1)
@DisplayName("신규 관심상품 등록")
void test1() {
String title = "Apple <b>에어팟</b> 2세대 유선충전 모델 (MV7N2KH/A)";
String imageUrl = "https://shopping-phinf.pstatic.net/main_1862208/18622086330.20200831140839.jpg";
String linkUrl = "https://search.shopping.naver.com/gate.nhn?id=18622086330";
int lPrice = 173900;
ProductRequestDto requestDto = new ProductRequestDto(
title,
imageUrl,
linkUrl,
lPrice
);
user = userRepository.findById(1L).orElse(null);
ProductResponseDto product = productService.createProduct(requestDto, user);
assertNotNull(product.getId());
assertEquals(title, product.getTitle());
assertEquals(imageUrl, product.getImage());
assertEquals(linkUrl, product.getLink());
assertEquals(lPrice, product.getLprice());
assertEquals(0, product.getMyprice());
createdProduct = product;
}
@Test
@Order(2)
@DisplayName("신규 등록된 관심상품의 희망 최저가 변경")
void test2() {
Long productId = this.createdProduct.getId();
int myPrice = 173000;
ProductMypriceRequestDto requestDto = new ProductMypriceRequestDto();
requestDto.setMyprice(myPrice);
ProductResponseDto product = productService.updateProduct(productId, requestDto);
assertNotNull(product.getId());
assertEquals(this.createdProduct.getTitle(), product.getTitle());
assertEquals(this.createdProduct.getImage(), product.getImage());
assertEquals(this.createdProduct.getLink(), product.getLink());
assertEquals(this.createdProduct.getLprice(), product.getLprice());
assertEquals(myPrice, product.getMyprice());
this.updatedMyPrice = myPrice;
}
@Test
@Order(3)
@DisplayName("회원이 등록한 모든 관심상품 조회")
void test3() {
Page<ProductResponseDto> productList = productService.getProducts(user,
0, 10, "id", false);
Long createdProductId = this.createdProduct.getId();
ProductResponseDto foundProduct = productList.stream()
.filter(product -> product.getId().equals(createdProductId))
.findFirst()
.orElse(null);
assertNotNull(foundProduct);
assertEquals(this.createdProduct.getId(), foundProduct.getId());
assertEquals(this.createdProduct.getTitle(), foundProduct.getTitle());
assertEquals(this.createdProduct.getImage(), foundProduct.getImage());
assertEquals(this.createdProduct.getLink(), foundProduct.getLink());
assertEquals(this.createdProduct.getLprice(), foundProduct.getLprice());
assertEquals(this.updatedMyPrice, foundProduct.getMyprice());
}
}