✍ 수강신청 준비하기
- 수강신청 조건
- "information technology" 과목 >> 1학년 신청 불가
- "commerce" 과목 >> 4학년 신청 불가
- 수강생이 5명 미만인 강의는 폐강
- 문제
- 기존 DataFrame에 "status"라는 이름의 column 추가
- 수강 가능 상태이면 "allowed"
- 수강 불가능한 상태이면 "not allowed"
import pandas as pd
df = pd.read_csv('data/enrolment_1.csv')
df["status"] = "allowed"
- "information technology" 과목을 수강하는 1학년
# 원하는 조건의 값만 변경하는 방법
df.loc[조건1 & 조건2, "coulmn 이름"] = "변경 값"
boolean1 = df["course name"] == information technology"
boolean2 = df["year"] == 1
df.loc[boolean1 & boolean2, "status"] = "not allowed"
boolean3 = df["course name"] == "commerce"
boolean4 = df["year"] == 4
df.loc[boolean3 & boolean4, "status"] = "not allowed"
# status가 allowed인 데이터만 모으기
# 위 두 조건에 해당하지 않는 데이터만 추출
allowed = df["status"] == "allowed"
# "course name"에서 allowed 조건에 해당하는 셀들의 수
course_counts = df.loc[allowed, "course name"].value_counts()
course_counts
arts 158
science 124
commerce 101
english 56
education 41
...
chemstry 1
jmc 1
b.ed 1
pgdca 1
sciences 1
Name: course name, Length: 296, dtype: int64
# Series에서 값이 True인 row들만 선택
Series[Series] or Series[조건]
# Series에서 row(index)만 추출
Series.index
closed_courses = list(course_counts[course_counts < 5].index)
closed_courses
['refactoring', 'computer architecture', ... , 'pg.diploma', 'aqua culture']
- 폐강 과목의 "status"를 "not allowed"로 설정하기
df.loc[df['course name'] == 'course1', 'status'] = 'not allowed' # 반복
for course in closed_courses:
df.loc[df["course name"] == course, "status"] = "not allowed"
df
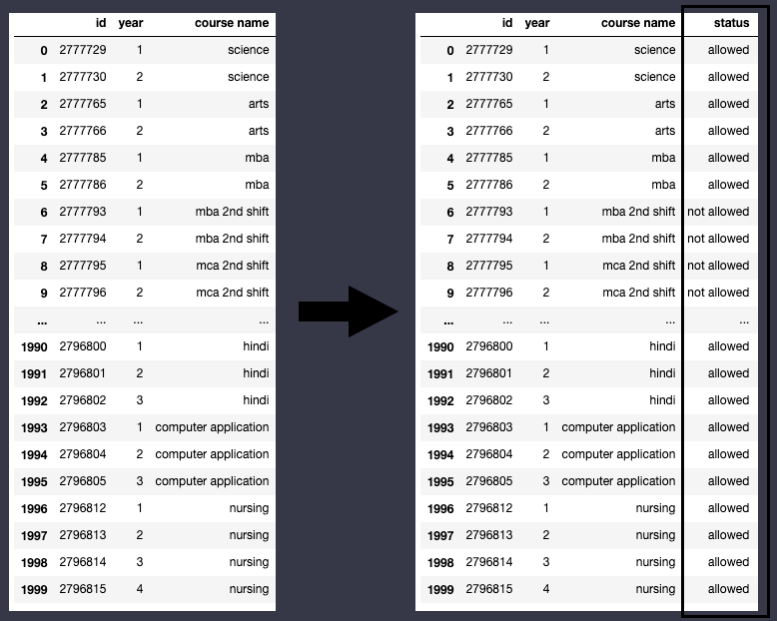
import pandas as pd
df = pd.read_csv('data/enrolment_1.csv')
# 코드를 작성하세요.
df['status']='allowed'
first=(df['year']==1) & (df['course name']=='information technology')
df.loc[first, 'status'] = 'not allowed'
fourth=(df['year']==4) & (df['course name']=='commerce')
df.loc[fourth, 'status'] = 'not allowed'
students=df['course name'].value_counts() < 5
students_2=students[students] # 5명 아래인 코스들
students_list=list(students_2.index) # 리스트
students_list
for i in students_list:
df.loc[df['course name'] == i, 'status'] = 'not allowed'
# 정답 출력
df
✍ 강의실 배정하기
- 조건
- 80명 이상의 학생이 수강하는 과목 >> "Auditorium"
- 40명 이상, 80명 미만의 학생이 수강하는 과목 >> "Large room"
- 15명 이상, 40명 미만의 학생이 수강하는 과목 >> "Medium room"
- 5명 이상, 15명 미만의 학생이 수강하는 과목 >> "Small room"
- status가 "not allowed"인 수강생의 room assignment >> "not assigned"
- "status"가 "allowed"인 course들의 수강 인원
import pandas as pd
df = pd.read_csv('data/enrolment_2.csv')
allowed = df["status"] == "allowed"
course_counts = df.loc[allowed, "course name"].value_counts()
course_counts
arts 158
science 124
commerce 101
english 56
...
mca 5
interior design 5
building construction and mangement 5
nanotechnology 5
Name: course name, Length: 82, dtype: int64
- 각 강의실 규모에 해당하는 과목 리스트 만들기
auditorium_list = list(course_counts[course_counts >= 80].index)
large_list = list(course_counts[(80 > course_counts) & (course_counts >= 40)].index)
medium_list = list(course_counts[(40 > course_counts) & (course_counts >= 15)].index)
small_list = list(course_counts[(15 > course_counts) & (course_counts > 4)].index)
- for문으로 room assignment 값 정하기
# not allowed 과목
not_allowed = df["status"] == "not allowed"
df.loc[not_allowed, "room assignment"] == "not assigned"
# allowed 과목
for course in auditorium_list:
df.loc[(df["course name"] == course) & allowed, "room assignment"] = "Auditorium"
for course in large_list:
df.loc[(df["course name"] == course) & allowed, "room assignment"] = "Large room"
for course in medium_list:
df.loc[(df["course name"] == course) & allowed, "room assignment"] = "Medium room"
for course in small_list:
df.loc[(df["course name"] == course) & allowed, "room assignment"] = "Small room"
df
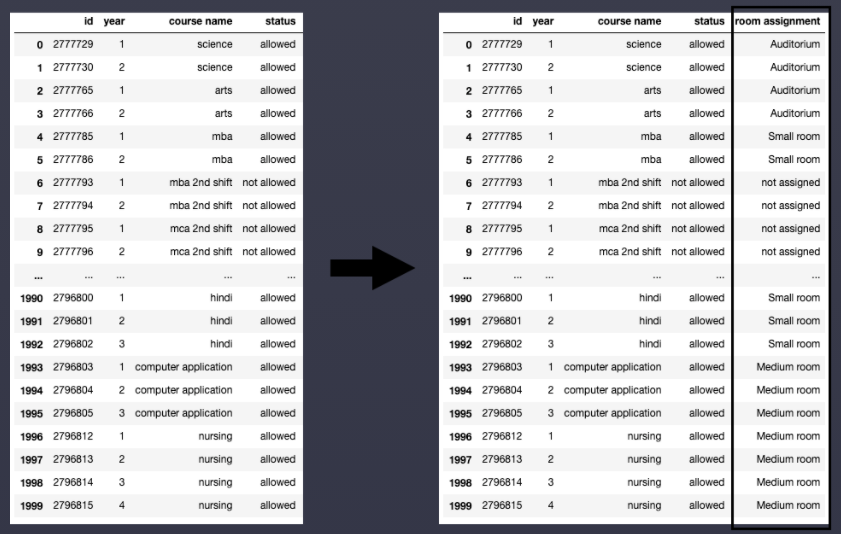
import pandas as pd
df = pd.read_csv('data/enrolment_2.csv')
# 코드를 작성하세요.
df['room assignment']='not assigned'
auditorium=(df['course name'].value_counts())>=80
auditorium=auditorium[auditorium]
auditorium_list=list(auditorium.index)
for i in auditorium_list:
df.loc[df['course name'] == i, 'room assignment'] = 'Auditorium'
large=((df['course name'].value_counts())>=40) & ((df['course name'].value_counts())<80)
large=large[large]
large_list=list(large.index)
for j in large_list:
df.loc[df['course name'] == j, 'room assignment'] = 'Large room'
medium=((df['course name'].value_counts())>=15) & ((df['course name'].value_counts())<40)
medium=medium[medium]
medium_list=list(medium.index)
for k in medium_list:
df.loc[df['course name'] == k, 'room assignment'] = 'Medium room'
small=((df['course name'].value_counts())>=5) & ((df['course name'].value_counts())<15)
small=small[small]
small_list=list(small.index)
for l in small_list:
df.loc[df['course name'] == l, 'room assignment'] = 'Small room'
no_room=(df['status']=='not allowed')
df.loc[no_room,'room assignment']='not assigned'
# 정답 출력
df
✍ 강의실 배정하기 2
- 문제
- room assignment에 따라 강의실 이름 지정하기
- 조건
- 알파벳 순서대로 강의실 번호 배정하기
- "arts" >> "Auditorium-1" / "commerce" >> "Auditorium-2"
- "status" column이 "not allowed"인 수강생
- "room assignment" column을 그대로 "not assigned"로 남겨두기
- "room assignment" column의 이름을 "room number"로 바꾸기
- "status"가 "allowed"인 수강생들의 과목별 수강 인원
import pandas as pd
df = pd.read_csv('data/enrolment_3.csv')
allowed = df["status"] == "allowed"
course_counts = df.loc[allowed, "course name"].value_counts()
- 각 강의실 규모에 해당되는 과목 리스트 만들기
auditorium_list = list(course_counts[course_counts >= 80].index)
large_list = list(course_counts[(80 > course_counts) & (course_counts >= 40)].index)
medium_list = list(course_counts[(40 > course_counts) & (course_counts >= 15)].index)
small_list = list(course_counts[(15 > course_counts) & (course_counts > 4)].index)
for i in range(len(auditorium_list)):
df.loc[(df["course name"] == sorted(auditorium_list)[i]) & allowed, "room assignment"] = "Auditorium- + str(i+1)
for i in range(len(large_list)):
df.loc[(df["course name"] == sorted(large_list)[i]) & allowed, "room assignment"] = "Large-" + str(i + 1)
for i in range(len(medium_list)):
df.loc[(df["course name"] == sorted(medium_list)[i]) & allowed, "room assignment"] = "Medium-" + str(i + 1)
for i in range(len(small_list)):
df.loc[(df["course name"] == sorted(small_list)[i]) & allowed, "room assignment"] = "Small-" + str(i + 1)
df.rename(columns={"room assignment":"room number"}, inplace=True)
df
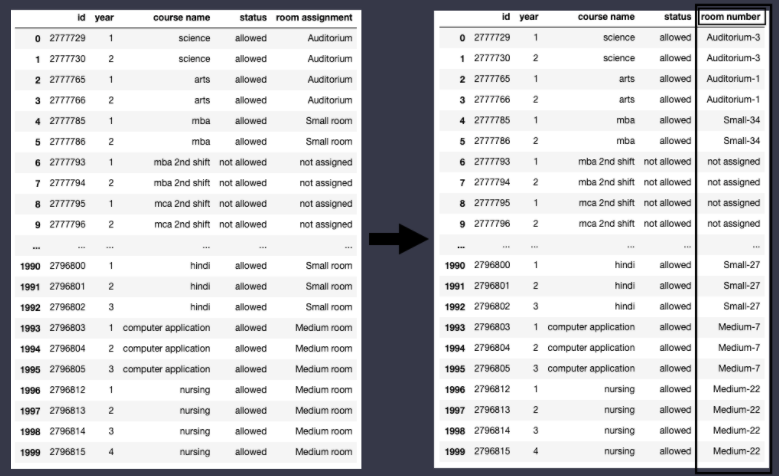
import pandas as pd
df = pd.read_csv('data/enrolment_3.csv')
# 코드를 작성하세요.
auditorium=(df['room assignment']=='Auditorium')
auditorium=df.loc[auditorium, 'course name'].value_counts()
auditorium_list=list(sorted(auditorium.index))
for i in range(len(auditorium_list)):
df.loc[df['course name'] == auditorium_list[i], 'room assignment'] = 'Auditorium' + "-" + str(i + 1)
large=(df['room assignment']=='Large room')
large=df.loc[large, 'course name'].value_counts()
large_list=list(sorted(large.index))
for j in range(len(large_list)):
df.loc[df['course name'] == large_list[j], 'room assignment'] = 'Large' + "-" + str(j + 1)
medium=(df['room assignment']=='Medium room')
medium=df.loc[medium, 'course name'].value_counts()
medium_list=list(sorted(medium.index))
for k in range(len(medium_list)):
df.loc[df['course name'] == medium_list[k], 'room assignment'] = 'Medium' + "-" + str(k + 1)
small=(df['room assignment']=='Small room')
small=df.loc[small, 'course name'].value_counts()
small_list=list(sorted(small.index))
for l in range(len(small_list)):
df.loc[df['course name'] == small_list[l], 'room assignment'] = 'Small' + "-" + str(l + 1)
no_room=(df['status']=='not allowed')
df.loc[no_room,'room assignment']='not assigned'
df.rename(columns={'room assignment':'room number'}, inplace=True)
# 정답 출력
df
* 출처: CODEIT - 데이터 사이언스 입문