controller가 하나인 경우
- controller에서 ExceptionHandler 추가
@ExceptionHandler(DMakerException.class)
public DMakerErrorResponse handleException(DMakerException e, HttpServletRequest request){
log.error("errorCode : {}, url : {}, message : {}",
e.getDMakerErrorCode(), request.getRequestURL(), e.getDetailMessage());
return DMakerErrorResponse.builder()
.errorCode(e.getDMakerErrorCode())
.errorMessage(e.getDetailMessage())
.build();
}
- DMakerErrorResponse 클래스 생성
package com.fastcampus.programming.dmaker.dto;
import com.fastcampus.programming.dmaker.exception.DMakerErrorCode;
import lombok.*;
@Getter
@Setter
@AllArgsConstructor
@NoArgsConstructor
@Builder
public class DMakerErrorResponse {
private DMakerErrorCode errorCode;
private String errorMessage;
}
- 테스트
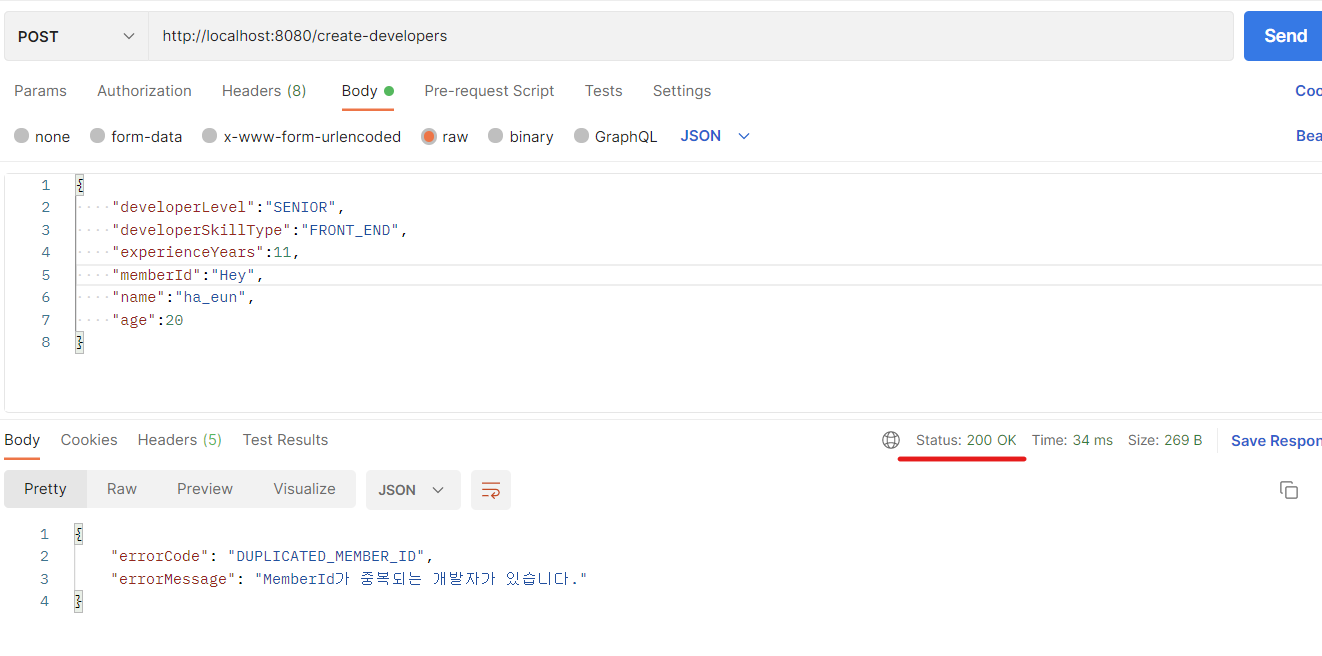
- Response의 상태코드 200을 변경하고 싶을 때
@ResponseStatus(value = HttpStatus.CONFLICT)
어노테이션 붙여줌
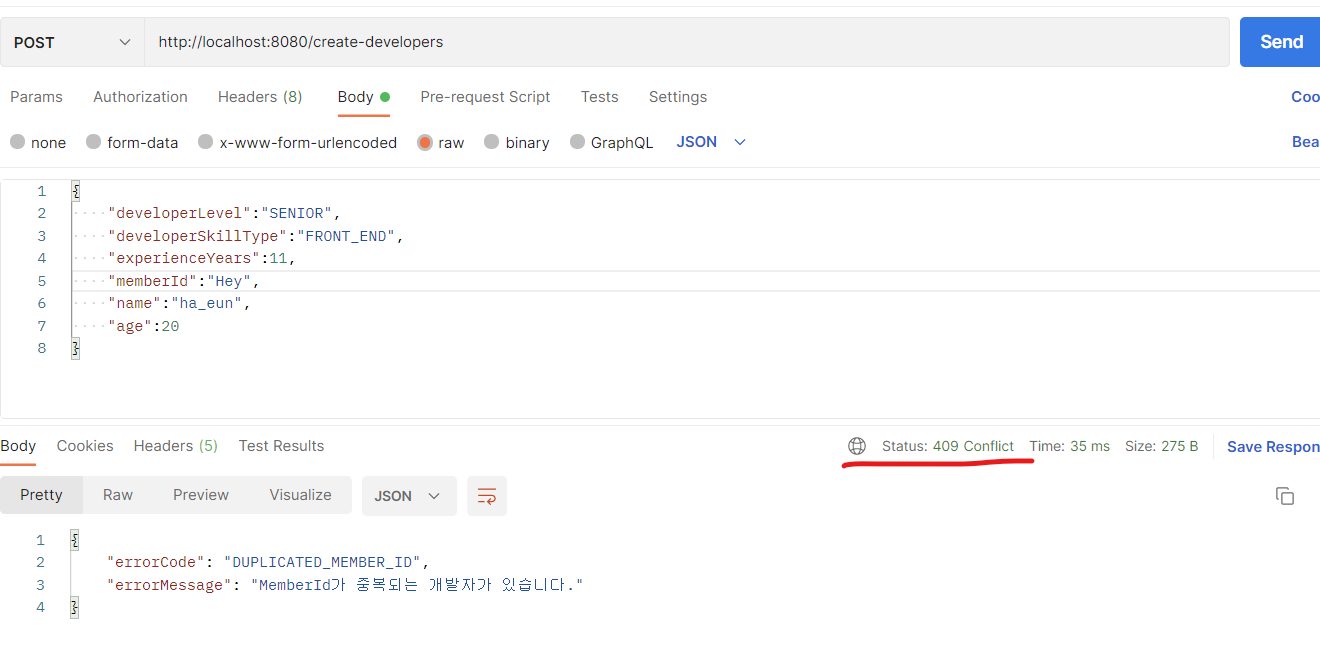
- 상태코드가 409 Conflict로 변경됨
-> 실무에서는 잘 사용하지 않음
-> 상태코드는 200으로 하고 세부적인 에러 내용 넘겨줌
cotroller가 여러개인 경우
- DMakerExceptionHandler 클래스 생성
@Slf4j
@RestControllerAdvice
public class DMakerExceptionHandler {
@ExceptionHandler(DMakerException.class)
public DMakerErrorResponse handleException(DMakerException e, HttpServletRequest request){
log.error("errorCode : {}, url : {}, message : {}",
e.getDMakerErrorCode(), request.getRequestURL(), e.getDetailMessage());
return DMakerErrorResponse.builder()
.errorCode(e.getDMakerErrorCode())
.errorMessage(e.getDetailMessage())
.build();
}
}
@RestControllerAdvice
어노테이션 적용
- 모든 @RestController에 대한, 전역적으로 발생할 수 있는 예외를 잡아서 처리할 수 있다.
- DMakerException이 발생했을 때 DMakerErrorCode와 메시지를 리턴한다.
@ExceptionHandler(value = {
HttpRequestMethodNotSupportedException.class,
MethodArgumentNotValidException.class
})
public DMakerErrorResponse handleBadRequest(Exception e, HttpServletRequest request){
log.error("url : {}, message : {}",
request.getRequestURL(), e.getMessage());
return DMakerErrorResponse.builder()
.errorCode(INVALID_REQUEST)
.errorMessage(INVALID_REQUEST.getMessage())
.build();
}
HttpRequestMethodNotSupportedException
: 지원하지 않은 HTTP method 호출 할 경우 발생 ex) put 요청을 post 요청으로 했을 때
MethodArgumentNotValidException
: validation이 실패했을 때 발생
@ExceptionHandler(Exception.class)
public DMakerErrorResponse handleException(Exception e, HttpServletRequest request){
log.error("url : {}, message : {}",
request.getRequestURL(), e.getMessage());
return DMakerErrorResponse.builder()
.errorCode(INTERNAL_SERVER_ERROR)
.errorMessage(INTERNAL_SERVER_ERROR.getMessage())
.build();
}
- 테스트
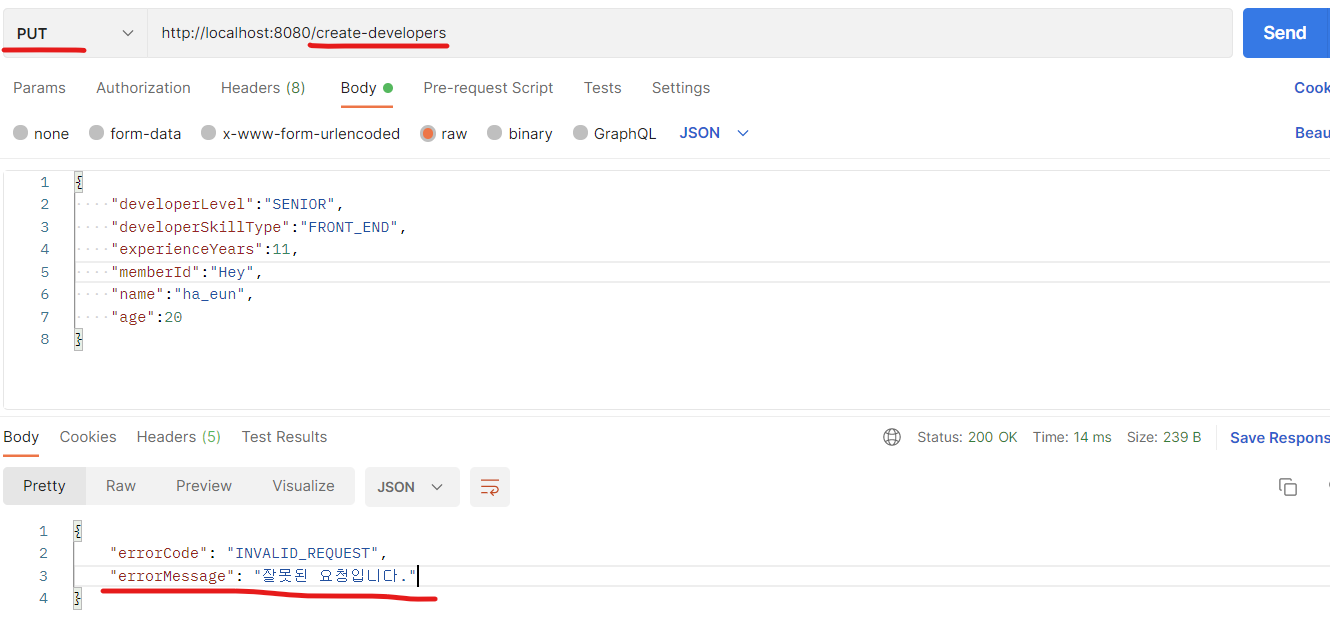

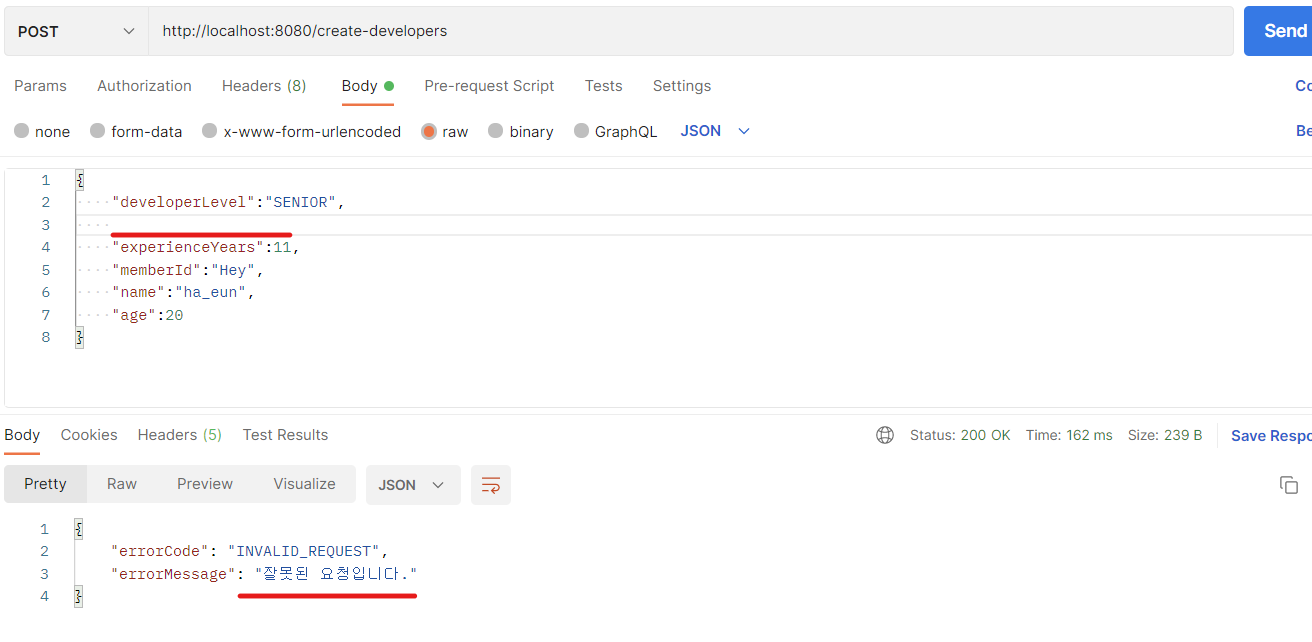

- url : http://localhost:8080/create-developers, message : Validation failed
[Field error in object 'request' on field 'developerSkillType': rejected value [null]
default message [널이어서는 안됩니다]
- validation이 실패했을 때 발생 (
MethodArgumentNotValidException
)
- 필수 요소인 developerSkillType이 없음