Why typescript?
- Add types
- Non javascript features (interface, generic)
- Next-gen javascript features (like babel)
- Rich configuration options
- Less bugs
Types
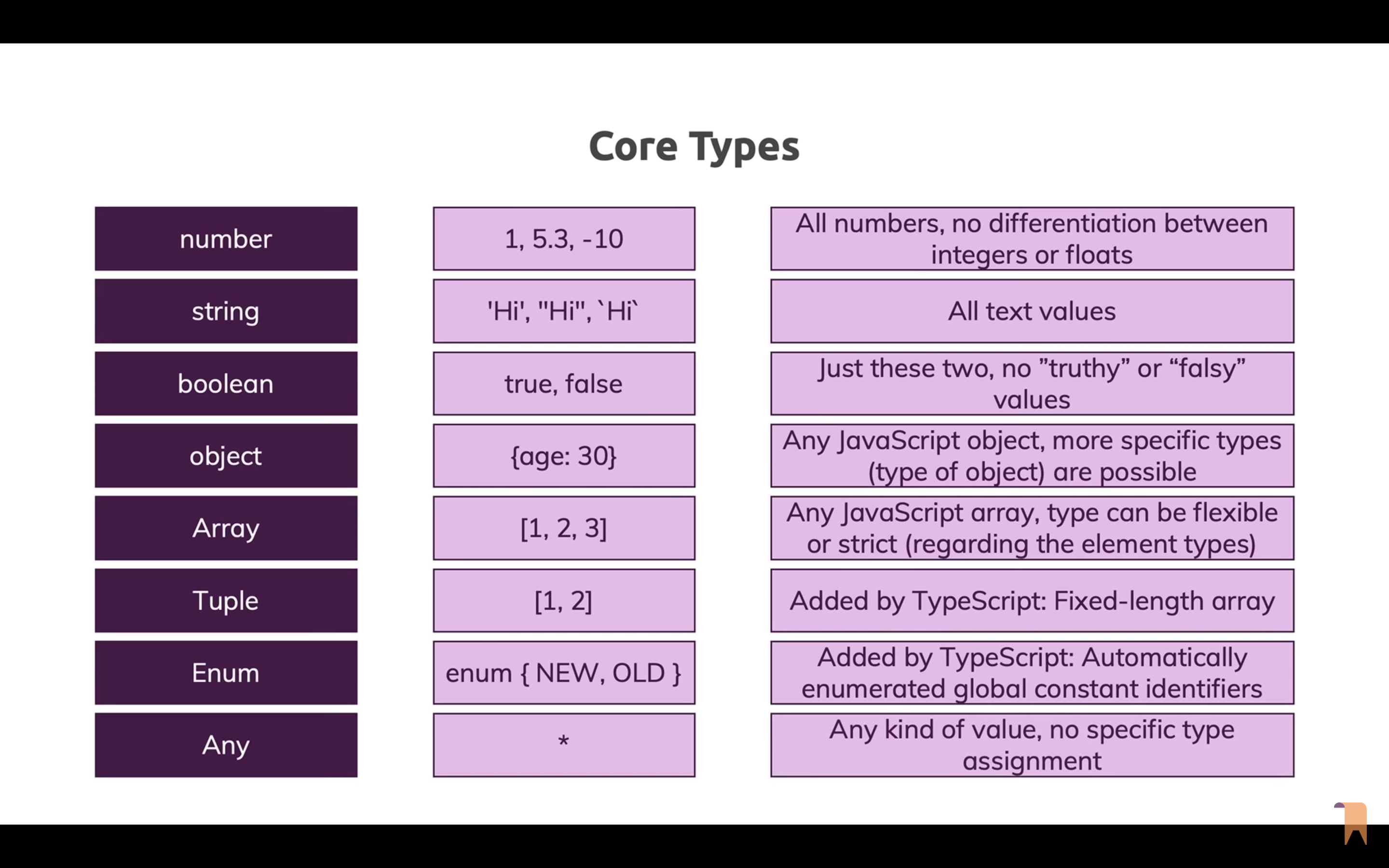
- boolean
- number
- string
- array
- object
- tuple: An array with a fixed number of elements whose types are known, but need not be the same.
- enum: Names to sets of numeric values.
- unknown: Values may come from dynamic content or API.
- any: Values from code that has been written without TypeScript or a 3rd party library.
- void: The absence of having any type at all as the return type of functions that do not return a value. (console.log)
- Null and Undefined
- never: The type of values that never occur like returning an error.
let isDone: boolean = false;
let num: number = 6;
let color: string = "blue";
let sentence: string = `Hello, my name is ${fullName}.`
let list: number[] = [1, 2, 3];
let list: Array<number> = [1, 2, 3];
function create(o: object | null): void;
create({ prop: 0 });
create(null);
let x: [string, number];
x = ["hello", 10];
x.push('something');
enum Color {
Red = 1,
Green,
Blue,
}
let c: Color = Color.Green;
let userInput: unknown;
let userName: string;
userInput = 5;
userInput = 'Suyeon'
if(typeof userInput === 'string') {
userName = userInput;
}
function getValue(key: string): any;
const str: string = getValue("myString");
function warnUser(): void {
console.log("This is my warning message");
}
let unusable: void = undefined;
unusable = null;
let u: undefined = undefined;
let n: null = null;
function error(message: string): never {
throw new Error(message);
}
enum
- Set of variables for abstraction
- Readability
- String / number
- Key-value pair (reverse mapping)