Make a converter with many units.
<!DOCTYPE html>
<html>
<body>
<div id="root"></div>
</body>
<script src="https://unpkg.com/react@17.0.2/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@17.0.2/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
<script type="text/babel">
const root = document.getElementById("root");
const MinutesToHours = () => {
const [amount, setAmount] = React.useState();
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => setAmount("");
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<div>
<label htmlFor="minutes">Minutes</label>
<input
value={inverted ? amount * 60 : amount}
id="minutes"
placeholder="Minutes"
type="number"
onChange={onChange}
disabled={inverted}
/>
</div>
<div>
<label htmlFor="hours">Hours</label>
<input
value={inverted ? amount : amount / 60}
id="hours"
placeholder="Hours"
type="number"
disabled={!inverted}
onChange={onChange}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>
{inverted ? "Turn back" : "Invert"}
</button>
</div>
);
};
const KmToMiles = () => {
const [amount, setAmount] = React.useState();
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => setAmount("");
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<div>
<label htmlFor="km">km</label>
<input
value={inverted ? amount * 1.609344 : amount}
id="km"
placeholder="km"
type="number"
onChange={onChange}
disabled={inverted}
/>
</div>
<div>
<label htmlFor="miles">miles</label>
<input
value={inverted ? amount : amount * 0.621371}
id="miles"
placeholder="miles"
type="number"
onChange={onChange}
disabled={!inverted}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>
{inverted ? "Turn back" : "Invert"}
</button>
</div>
);
};
const MmToInch = () => {
const [amount, setAmount] = React.useState();
const [inverted, setInverted] = React.useState(false);
const onChange = (event) => {
setAmount(event.target.value);
};
const reset = () => setAmount("");
const onInvert = () => {
reset();
setInverted((current) => !current);
};
return (
<div>
<div>
<label for="mm">mm</label>
<input
value={inverted ? amount * 25.4 : amount}
id="mm"
placeholder="mm"
type="number"
onChange={onChange}
disabled={inverted}
/>
</div>
<div>
<label for="inch">inch</label>
<input
value={inverted ? amount : amount * 0.03937}
id="inch"
placeholder="inch"
type="number"
onChange={onChange}
disabled={!inverted}
/>
</div>
<button onClick={reset}>Reset</button>
<button onClick={onInvert}>
{inverted ? "Turn back" : "Invert"}
</button>
</div>
);
};
const App = () => {
const [index, setIndex] = React.useState("x");
const onSelect = (event) => {
setIndex(event.target.value);
};
return (
<div>
<h1>Super Converter</h1>
<select value={index} onChange={onSelect}>
<option value="x">Select your units.</option>
<option value="0">Minutes & Hours</option>
<option value="1">Km & Miles</option>
<option value="2">mm & inch</option>
</select>
<hr />
{
index === "x" ? "Please Select your units." : null
}
{index === "0" ? <MinutesToHours /> : null}
{index === "1" ? <KmToMiles /> : null}
{index === "2" ? <MmToInch /> : null}
</div>
);
};
ReactDOM.render(<App />, root);
</script>
</html>
원리
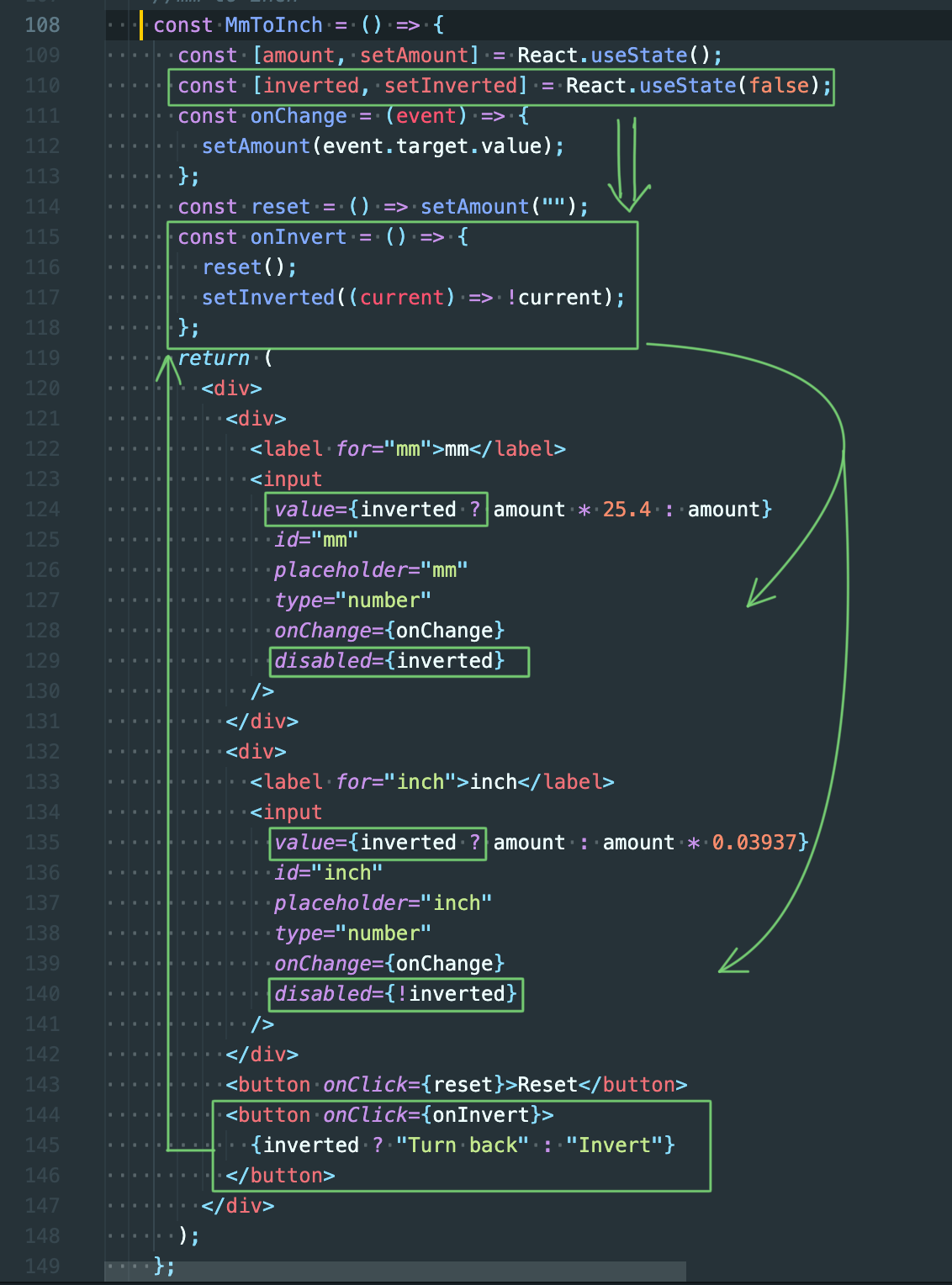