참조변수 super(=this)
- 객체 자신을 가르키는 참조변수
- 인스턴스 메서드(생성자)내에서만 존재
- 조상의 멤버를 자신의 멤버와 구별할 때 사용됨
- 조상에게는 super, 자식에게는 this를 붙임
코드
class Ex7_2 {
public static void main(String args[]) {
Child c = new Child();
c.method()[
}
}
class Parent { int x = 10; }
class Child extend Parent {
int x = 20;
void method() {
System.out.println("x=" + x);
System.out.println("x=" + this.x);
System.out.println("x=" + super.x);
- 3개의 멤버를 지니고 있음
- super.x != this.x(서로 다름)
코드2
class Ex7_3 {
public static void main(String args[]) {
Child2 c = new Child2();
c.method()[
}
}
class Parent2 { int x = 10; }
class Child2 extend Parent2 {
void method() {
System.out.println("x=" + x);
System.out.println("x=" + this.x);
System.out.println("x=" + super.x);
- 2개의 멤버를 가지고 있음
- super.x = this.x(서로 같음)
super() - 조상의 생성자
- 조상의 생성자를 호출할 때 사용됨
- 조상의 멤버는 조상의 생성자를 호출해서 초기화
- 생성자의 첫 줄에 반드시 생성자를 호출해야 됨 > 그렇지 않으면 컴파일러가 생성자의 첫 줄에 super();를 삽입함(중요)
class Point {
int x, y;
Point(int x, int y) {
this.x = x;
this.y = y;
}
}
class Point3D extends Point {
int z;
class Point3D(int x, int y, int z {
super(x, y);
this.z = z;
}
}
예제
package hello;
class Point {
int x;
int y;
Point(int x, int y) {
this.x = x;
this.y = y;
}
String getLocation() {
return "x:" + x + "y:" + y;
}
}
class Point3D extends Point {
int z;
Point3D(int x, int y, int z) {
this.x = x;
this.y = y;
this.z = z;
}
String getLocation() {
return "x:" + x + "y:" + y + "z:" + z;
}
}
public class Super {
public static void main(String[] args) {
}
}
결과
- 컴파일러 에러
- 자식 생성자에서 부모 생성자를 this로 정의했기 때문에
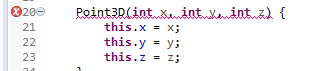
예제2
package hello;
class Point {
int x;
int y;
Point(int x, int y) {
super();
this.x = x;
this.y = y;
}
String getLocation() {
return "x:" + x + "y:" + y;
}
}
class Point3D extends Point {
int z;
Point3D(int x, int y, int z) {
super(x,y);
this.z = z;
}
String getLocation() {
return "x:" + x + ", y:" + y + ", z:" + z;
}
}
public class Super {
public static void main(String[] args) {
Point3D p3 = new Point3D(1,2,3);
System.out.println(p3);
System.out.println(p3.getLocation());
}
}
결과2
- 모든 생성자는 첫줄에 다른 생성자를 호출해야 됨 > super();
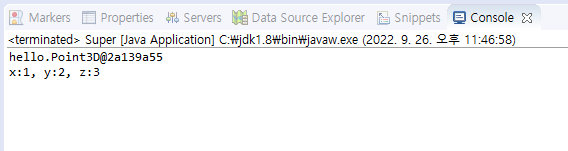