드림코딩 by 엘리 유투버님의 영상인 ('자바스크립트 프로처럼 쓰는 팁')을 보고 스스로 정리한 포스팅입니다! 자세한 내용은 https://www.youtube.com/watch?v=BUAhpB3FmS4&t=758s 요 영상을 참고하세요
// ❌ Bad Code 💩
function getResult(score) {
let result;
if (score > 5) result = 'good!';
else if (score <= 5) result = 'bad!';
return result;
}
// ✅ Good Code ✨
function getResult(score) {
return score > 5 ? 'good!' : 'bad!';
}
// ❌ Bad Code 💩
function printMessage(text) {
let message = text;
if (text == null || text == undefined) {
message = 'Nothing to display 😜';
}
console.log(message);
}
// ✅ Good Code ✨ Ver1. Nullish coalescing operator
function printMessage(text) {
const message = text ?? 'Nothing to display 😜';
console.log(message);
}
// ✅ Good Code ✨ Ver2. default parameter
function printMessage2(text = 'Nothing to display 😜') {
console.log(message);
}
/* default parameter는 undefined에만 적용 */
printMessage('Hello'); // Hello
printMessage(undefined); // Nothing to display 😜
printMessage(null); // Nothing to display 😜
const result = getInitialState() ?? fetchFromServer();
// 코드를 실행한 값을 할당할 때에도 사용됨
console.log(result);
function getInitialState() {
retunr null;
}
function fetchFromServer() {
retunr 'Hi';
}
const person = {
name : 'Sol',
age: 26,
phone: '01012345678',
}
// ❌ Bad Code 💩
function displayPerson(person) {
// case 1.
displayName(person.name);
...
// case 2.
const name = person.name
}
// ✅ Good Code ✨
function displayPerson(person) {
const { name, age } = person;
...
}
const item = { type : '👔', size : 'M' };
const detail = { price: 20, made : 'Korea', gender: 'M' }
// ❌ Bad Code 💩
// case1. 원본 값에 영향을 줌
item['price'] = detail.price;
// case2.
const newObject = new Object;
newObject['price'] = detail.price;
...
// case3.
const newObject2 = {
type: item.type;
}
// ✅ Good Code ✨ Ver1. 옛날 문법
const newObject = Object.assign(item, detail);
/*
=> 이렇게 하면 item에 detail 값이 들어가고, newObject === item이므로
item 객체가 오염되는 것을 원치 않으려면 아래와 같이 작성!
*/
const newObject = Object.assign({}, item, detail);
// ✅ Better! Code ✨
const shirt = {
...item,
...detail,
price : 40 // 기존 값을 유지하면서 특정값 변경 ㅇ
}
let fruits = ['🍈','🍑','🍒'];
// fruits.push('🍓');
fruits = [...fruits, '🍓'];
// fruits.unshift('🍓');
fruits = ['🍓', ...fruits];
let fruits2 = ['🍈','🍑','🍒'];
let combined = fruits.concat(fruits2);
combined = [...fruits, '🍒', ...fruits2];
const bob = {
name : 'julia',
age: 20,
};
const anna = {
name : 'julia',
age: 20,
job : {
title : 'Software Engineer',
},
};
// ❌ Bad Code 💩
function displayJobTitle(person) {
if (person.job && person.job.title) {
console.log(person.job.title);
}
}
// ✅ Good Code ✨
function displayJobTitle(person) {
if (person.job?.title) {
// 이 때, job이 없을때 정확히는 undefined 따라서, nullish polishing이 가능함
console.log(person.job.title);
}
}
// ✅ Good Code ✨ + nullish coalescing
function displayJobTitle(person) {
const title = person.job?.title ?? 'No Job Yet 🔥';
console.log(title);
}
}
const person = {
name : 'julia',
score: 4,
};
// ❌ Bad Code 💩
console.log(
'Hello ' + person.name;
);
// ✅ Good Code ✨
console.log(`Hello ${person.name}`);
// ✅ Good Code ✨
const { name, score } = person;
console.log(`Hello ${name}, ${score}`);
// ✅ Good Code ✨ + 확장성을 고려한 함수
function greetings(person) {
const { name, score } = person;
console.log(`Hello ${name}, ${score}`);
}
// Looping
const items = [1,2,3,4,5,6];
const result = 0;
// ✅ Good Code ✨
const evens = items.filter((num) => num % 2 === 0);
const multiple = evens.map((num) => num * 4);
const sum = multiple.reduce((a, b) => a + b, 0);
console.log(sum);
const result = items
.filter((num) => num % 2 === 0)
.map((num) => num * 4)
.reduce((a, b) => a + b, 0);
console.log(result);
const result = items
.reduce((sum, cur) => cur % 2 == 0? sum + cur * 4 : sum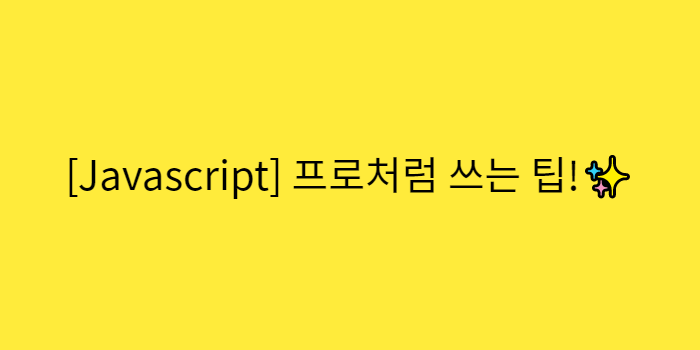, 0);
// Promise -> Async / await
// ❌ Bad Code 💩
function displayUser() {
fetchUser()
.then((user) => {
fetchProfile(user)
.then((profile) => {
updateUI(user, profile);
});
});
}
// ✅ Good Code ✨
async function displayUser() {
const user = await fetchUser();
const profile = await fetchProfile(user);
updateUI(user, profile);
}
const array = [1,2,3,1,2];
console.log([...new Set(array)]); // 3
/*
1. set을 통해 중복제거
2. set을 spread 연산자를 사용해서 새로운 배열로 만듦
*/