score = (9.5, 8.9, 9.2, 0.8, 8.8, 9.0)
score = list(score)
print(score)
score.sort()
score.pop(0)
score.pop(len(score)-1)
score = tuple(score)
sumScore = 0
for scores in score :
sumScore += scores
resultAver =int(sumScore/len(score))
print(f'{score}')
print(f'{sumScore}')
print(f'{resultAver}')
school = ((1,18),(2,19),(3,23),(4,21),(5,20),(6,22),(7,17))
sumStudent = 0
averageStudent = 0
count = 0
for classNo, student in school :
print(f'{classNo}학급 학생 수 : {student}')
sumStudent += student
count += 1
averageStudent = sumStudent / count
print(f'전체 학생 수 : {sumStudent}')
print(f'평균 학생 수 : {averageStudent}')
minScore = 60
kor = int(input('국어점수'))
math = int(input('수학점수'))
eng = int(input('영어점수'))
science = int(input('과학점수'))
history = int(input('국사점수'))
scoreList = (
('국어', kor),
('수핟', math),
('영어', eng),
('과핟', science),
('국사', history),
)
for subject, score in scoreList :
if score > minScore : continue
print(f'{subject} 점수 {score}로, 과락.')
schoolList = (
(1, 18),
(2, 19),
(3, 23),
(4, 21),
(5, 20),
(6, 22),
(7, 12)
)
maxStudent = 0
maxClass = 0
minStudent = 0
minClass = 0
for classNo, students in schoolList:
if students == 0 or maxStudent < students :
maxStudent = students
maxClass = classNo
if minStudent == 0 or minStudent > students :
minStudent = students
minClass = classNo
print(f'학생이 가장 많은 {maxClass}학급 {maxStudent}명')
print(f'학생이 가장 적은 {minClass}학급 {minStudent}명')
schoolList = (
(1, 18),
(2, 19),
(3, 23),
(4, 21),
(5, 20),
(6, 22),
(7, 17),
)
sum = 0
avg = 0
n = 0
while n < len(schoolList) :
print(f'{schoolList[n][0]}학급 학생수는 {schoolList[n][1]}')
sum += schoolList[n][1]
n += 1
avg = sum / n
print(f'전체학급 학생수 : {sum}')
print(f'학급 평균 학생수 : {avg}')
minscore = 60
kor = int(input('국어점수입력'))
eng = int(input('영어점수입력'))
math = int(input('수학점수입력'))
science = int(input('과학점수입력'))
history = int(input('국사점수입력'))
scoreList = (
('국어', kor),
('영어', eng),
('수학', math),
('과학', science),
('국사', history)
)
n = 0
while n <len(scoreList):
if scoreList[n][1] <minscore:
print(f'{scoreList[n][0]}과목 과락. 점수 {scoreList[n][1]}')
n += 1
myinfo = {'이름' : '오연수',
'전공' : '동물생명자원학과',
'이메일' : 'softwater960@gmail.com',
'연락처' : '010-9659-8242'
}
print(f'{myinfo}')
print(myinfo['이름'])
result = myinfo.get('이름')
print(result)
studentInfo = {}
studentInfo['name'] = input('이름입력')
studentInfo['grade'] = input('학년입력')
studentInfo['mail'] = input('메일입력')
studentInfo['addr'] = input('주소입력')
print(studentInfo)
studentInfo['etc'] = input('메모입력')
print(studentInfo)
facDic = {}
for i in range(11) :
if i == 0 :
facDic[i] = 1
else:
for j in range(1, i+1) :
facDic[i] = facDic[i-1] * j
print(f'facdic : {facDic}')
scores = {
'kor' : 88 ,
'eng' : 55 ,
'mat' : 85 ,
'sci' : 57 ,
'his' : 82
}
minscore = 60
result = 'F, 재시험'
if scores['kor'] <minscore : scores['kor'] = result
if scores['eng'] <minscore : scores['eng'] = result
if scores['mat'] <minscore : scores['mat'] = result
if scores['sci'] <minscore : scores['sci'] = result
if scores['his'] <minscore : scores['his'] = result
print(scores)
scores = {
'kor' : 88 ,
'eng' : 55 ,
'mat' : 85 ,
'sci' : 57 ,
'his' : 82
}
minscore = 60
result = 'F, 재시험'
fList = {}
for key in scores.keys():
if scores[key] < minscore :
scores[key] = result
fList[key] = result
print(scores)
print(fList)
scores = {
'score1' : 8.9 ,
'score2' : 8.1 ,
'score3' : 8.5 ,
'score4' : 9.8 ,
'score5' : 8.8
}
minScore = 10
minKey =''
maxScore = 0
maxKey = ''
for key in scores.keys():
if scores[key] <minScore :
minScore = scores[key]
minKey = key
if scores[key] >maxScore :
maxScore = scores[key]
maxKey = key
del scores[minKey]
del scores[maxKey]
print(scores)
in, not in only for check key is in dic
in 있으면 True, 없으면 False
not in 있으면 False, 없으면 True
len counting items(ㅁㅁ:ㅁㅁ)
all delete items in dic
myinfo = {
'이름' : '오연수',
'나이' : '27',
'연락처' : '010-1234-5678',
'주민등록번호' : '960314-2029518',
'주소' : '960314-2029518'
}
deleteIf = ['연락처', '주민등록번호']
for key in deleteIf :
if (key in myinfo) :
del myinfo[key]
print(myinfo)
문제: 주어진 리스트에서 중복된 요소를 제거하여 새로운 리스트를 생성하세요.
예시 입력: [1, 2, 3, 2, 4, 3, 5]
예시 출력: [1, 2, 3, 4, 5]
exList = list(map(int, input('예시 입력').split(', ')))
result = list(set(exList))
print(f'예시출력 :{result}')
print(type(result))
result 에서 set만 해두니까 타입이 set으로 나오더라. 그래서 list로 다시 묶어뒀어야함!
문제: 주어진 리스트에서 홀수만 남기고 짝수는 제거하여 리스트를 수정하세요.
예시 입력: [1, 2, 3, 4, 5, 6, 7, 8, 9]
예시 출력: [1, 3, 5, 7, 9]
exList = list(map(int, input('예시 입력').split(', ')))
result = []
for num in exList :
if num % 2 != 0 :
result.append(num)
print(result)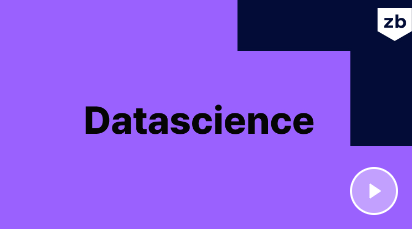