Given an array nums of integers, return how many of them contain an even number of digits.
Constraints:
- 1 <= nums.length <= 500
- 1 <= nums[i] <= 10^5
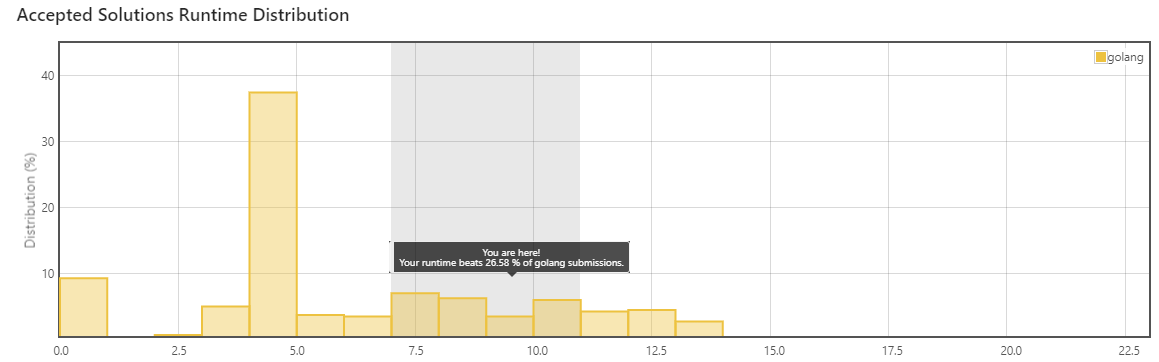
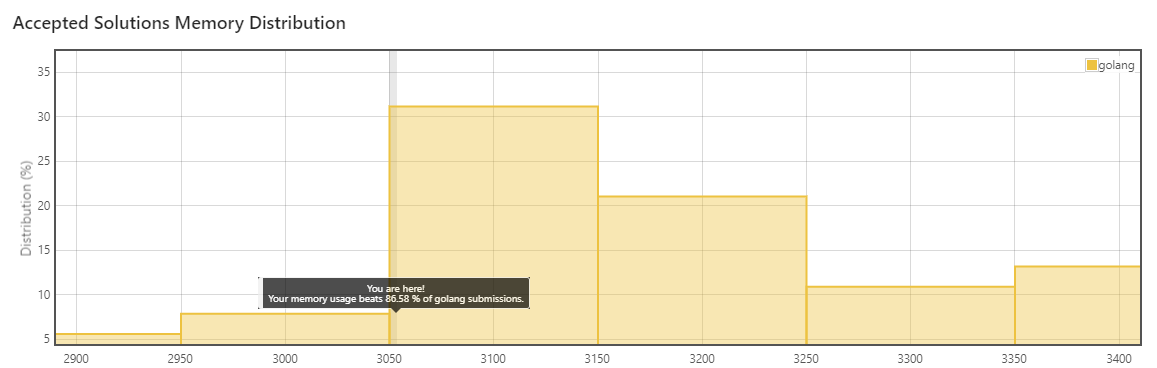
My code
func findNumbers(nums []int) int {
count := 0
for _, val := range nums {
for i := 10; 10^499/i > 1; {
if val/i < 1 {
break
} else if val/i >= 10 {
i = i * 100
} else {
count++
break
}
}
}
return count
}
- go.dev/play/p/2KlCdhBdEB4
- Due to nums length, I put power 499.
- length 10^1 = 2
- length 10^2 = 3
.
.
.
- length 10^499 = 500
- lenght even have patten about 10 * 100^n
- when starts, val / i(10 * 100^n) less than 1 will not be even
- example
- 1 / 10 = 0.1 (odd)
- this num will be passed because it is odd.
- 100 / 10 = 10 (odd?)
- 101 / 10 = 10.1 (odd?)
- 100 / 1000 = 0.1(odd)
sample 0 ms submission & Accepted Solutions Memory Distribution
Code
func getNumberOfDigits(n int) int {
var numDigit int
for n >= 1 {
numDigit += 1
n /= 10
}
return numDigit
}
func findNumbers(nums []int) int {
var numEven int
for _, n := range nums {
if getNumberOfDigits(n) % 2 == 0 {
numEven += 1
}
}
return numEven
}
- This code can check how many digits does num have.
- By checking number is more than 1, this code counts +1 digit and divide by 10 in getNumberofDigits.
- finally returned num digits were divided by 2 and remainder is 0 then it will be even.
- this code is perfect both sides(speed and size).