[Programmers/프로그래머스] 2020 KAKAO BLIND RECRUITMENT [코딩테스트]
- [Lv. 2] 문자열 압축
- [Lv. 2] 괄호 변환
- [Lv. 3] 자물쇠와 열쇠
- [Lv. 4] 가사 검색
- [Lv. 3] 기둥과 보 설치
- [Lv. 3] 외벽 점검
- [Lv. 3] 블록 이동하기
📌 문제
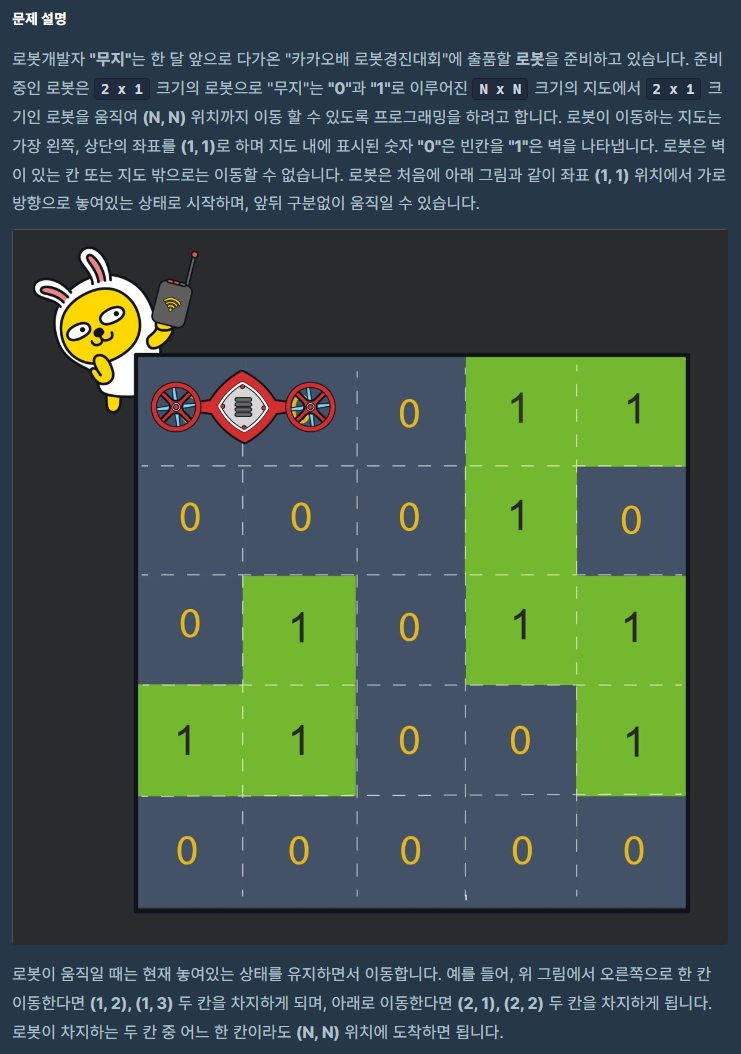
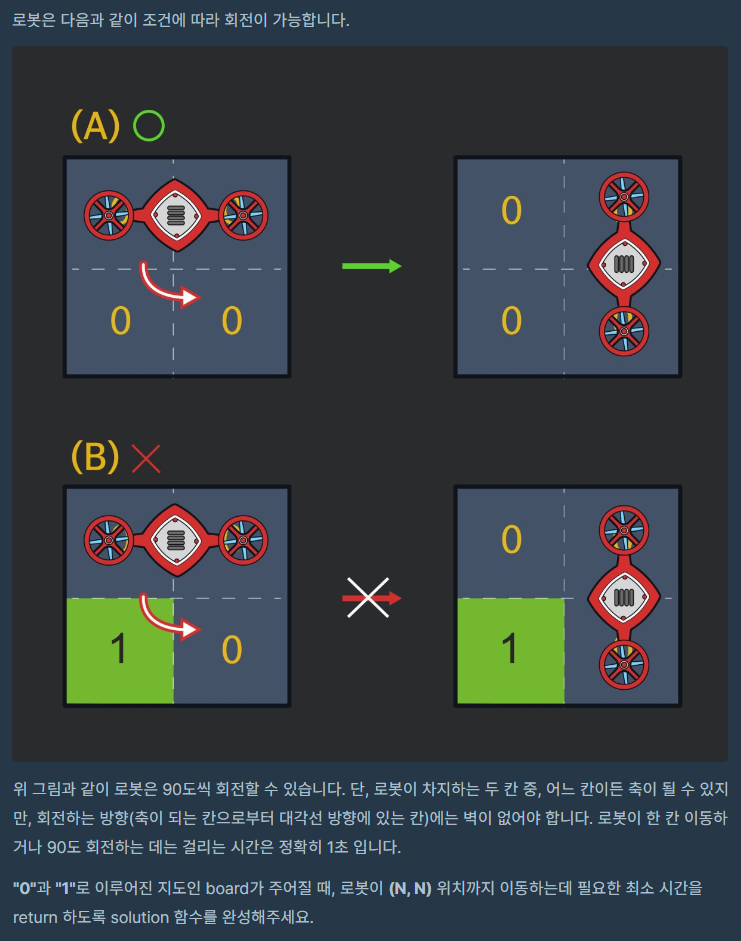
📝 제한사항
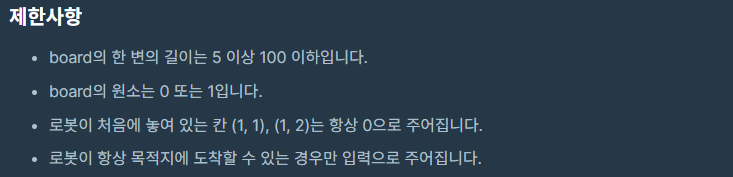
💻 입출력 예

📖 입출력 예에 대한 설명
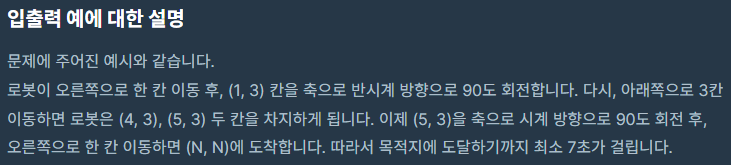
📌 풀이
from collections import deque
def get_next_position(position, board):
next_position = []
x1, y1 = list(position)[0]
x2, y2 = list(position)[1]
dx = [-1, 1, 0, 0]
dy = [0, 0, -1, 1]
for i in range(4):
nx1, ny1 = x1 + dx[i], y1 + dy[i]
nx2, ny2 = x2 + dx[i], y2 + dy[i]
if board[nx1][ny1] == 0 and board[nx2][ny2] == 0:
next_position.append({(nx1, ny1), (nx2, ny2)})
if x1 == x2:
for i in [-1, 1]:
if board[x1 + i][y1] == 0 and board[x2 + i][y2] == 0:
next_position.append({(x1, y1), (x1 + i, y1)})
next_position.append({(x2, y2), (x2 + i, y2)})
elif y1 == y2:
for i in [-1, 1]:
if board[x1][y1 + i] == 0 and board[x2][y2 + i] == 0:
next_position.append({(x1, y1), (x1, y1 + i)})
next_position.append({(x2, y2), (x2, y2 + i)})
return next_position
def solution(board):
n = len(board)
new_board = [[1] * (n + 2) for _ in range(n + 2)]
for row in range(n):
for col in range(n):
new_board[row + 1][col + 1] = board[row][col]
visited = []
start = {(1, 1), (1, 2)}
visited.append(start)
queue = deque([(start, 0)])
while queue:
position, cost = queue.popleft()
if (n, n) in position:
return cost
for next_position in get_next_position(position, new_board):
if next_position not in visited:
visited.append(next_position)
queue.append((next_position, cost + 1))
return 0