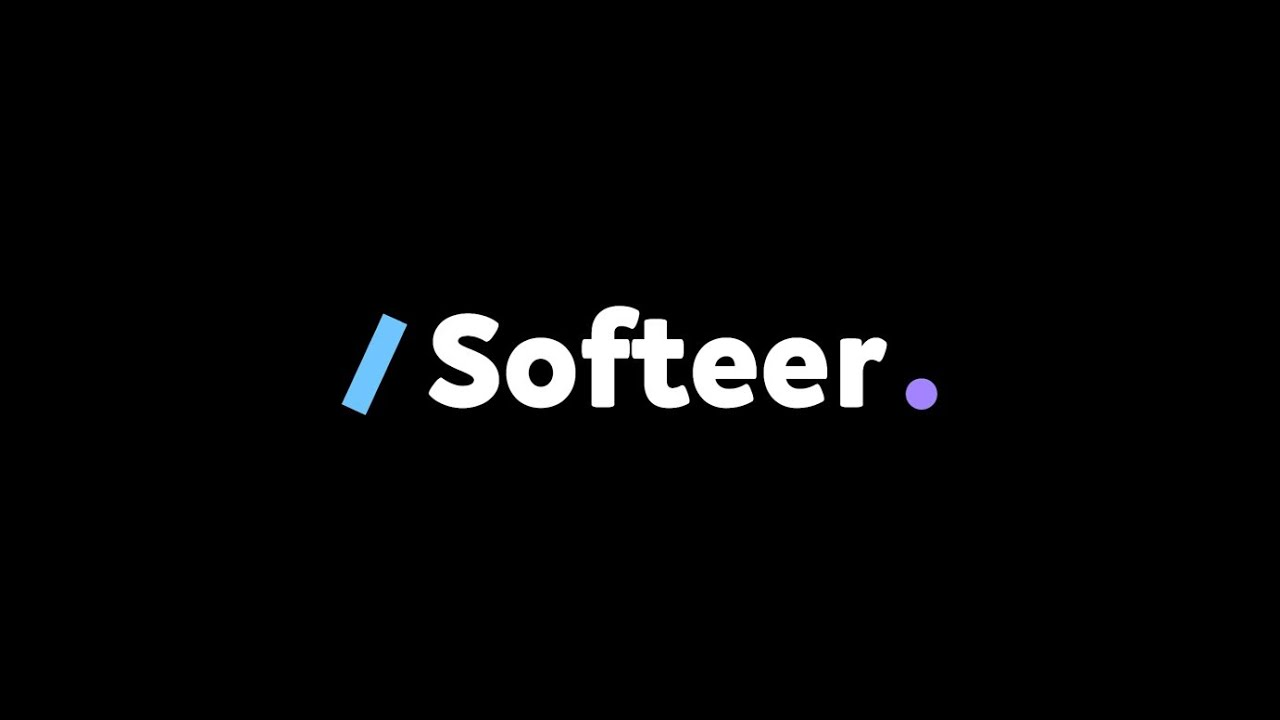
📃 문제
소프티어 - 좌석관리
📝 풀이
사용한 배열
- 좌석 정보 arr (N * M)
- 사원 정보 employee (Dictionary)
코드
import sys
input = sys.stdin.readline
def guard_check(x, y, person_num):
"""방역 좌석 체크
:param x, y: 사람이 앉은 자리
:param person_num:
방역 좌석 -= 1 을 해준다. (In일 경우)
사람이 식사 후 나가면 += 1을 하여 방역 좌석 체크
:return:
"""
for dx, dy in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
nx, ny = x + dx, y + dy
if 0 <= nx < N and 0 <= ny < M:
arr[nx][ny] += person_num
def search_seat(id):
"""좌석 탐색
:param id: 사원 id
:return: 상황에 맞는 문구 출력
"""
tx = ty = D = 0
for x in range(N):
for y in range(M):
min_d = 1000
if arr[x][y] == 0:
for xi, yi, ate in employee.values():
if ate: continue
d = ((xi - x) ** 2 + (yi - y) ** 2) ** 1 / 2
if min_d > d:
min_d = d
if D < min_d:
tx, ty = x, y
D = min_d
if arr[tx][ty] != 0:
return f'There are no more seats.'
employee[id] = (tx, ty, False)
arr[tx][ty] = id
guard_check(tx, ty, -1)
return f'{id} gets the seat ({tx + 1}, {ty + 1}).'
N, M, Q = map(int, input().split())
arr = [[0] * M for _ in range(N)]
employee = {}
'''
ate == False: 식사 중인 상태
ate == True: 식사 완료 상태
'''
for _ in range(Q):
q, id = input().split()
id = int(id)
if q == 'Out':
if id not in employee:
print(f"{id} didn't eat lunch.")
else:
x, y, ate = employee[id]
if ate:
print(f'{id} already left seat.')
else:
print(f'{id} leaves from the seat ({x + 1}, {y + 1}).')
employee[id] = (x, y, True)
arr[x][y] = 0
guard_check(x, y, 1)
else:
if id not in employee:
print(search_seat(id))
else:
x, y, ate = employee[id]
if ate:
print(f'{id} already ate lunch.')
else:
print(f'{id} already seated.')
❗ 느낀 점
- 분석을 확실히 하고 슈도 코드를 먼저 작성하니 테스트 케이스 확인 후 코드를 수정하는 시간이 확실히 짧았다.