GraphQL
은 API
를 위한 쿼리 언어이며, 데이터에 대해 정의한 타입 시스템을 사용하여 쿼리를 실행하기 위한 서버 측 런타임이다.
- 예시는 여기를 참고한다.
GraphQL
에 쿼리를 보내면 결과를 JSON
형태로 응답한다.
- 불필요한 데이터를 제외하여 API 데이터를 요청할 수 있다.
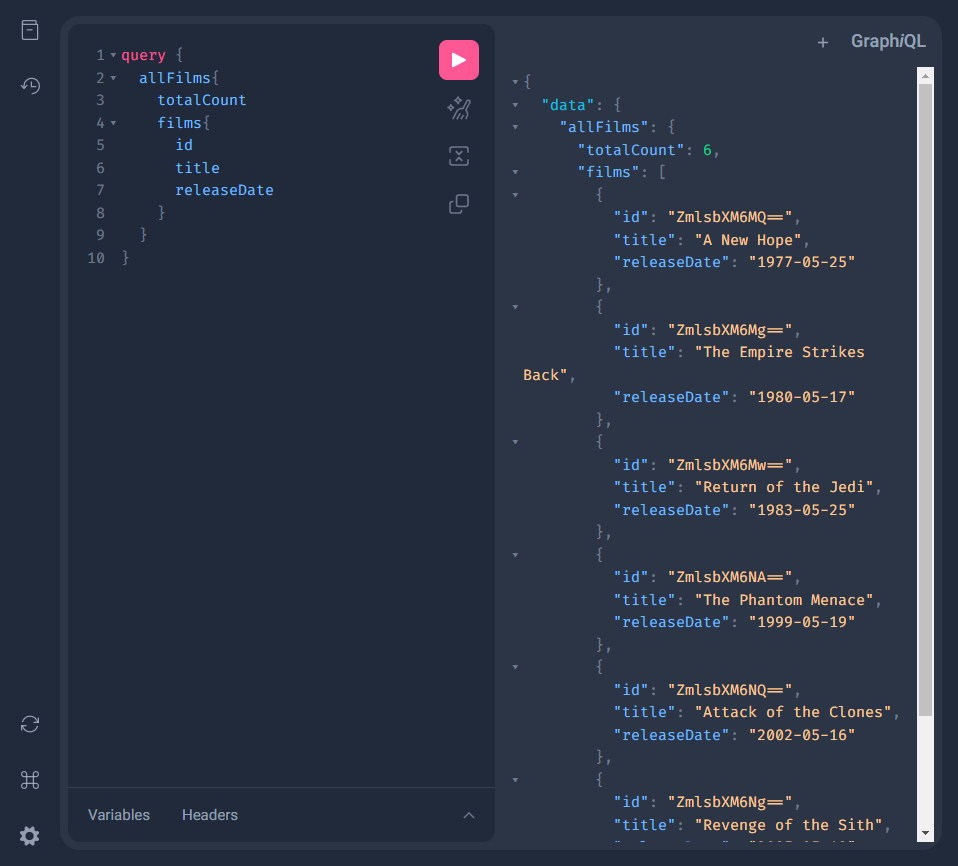
id
를 통한 요청 및 다른 데이터와의 관계 연결은 table(id:"~"){~Connection}
의 형태다.
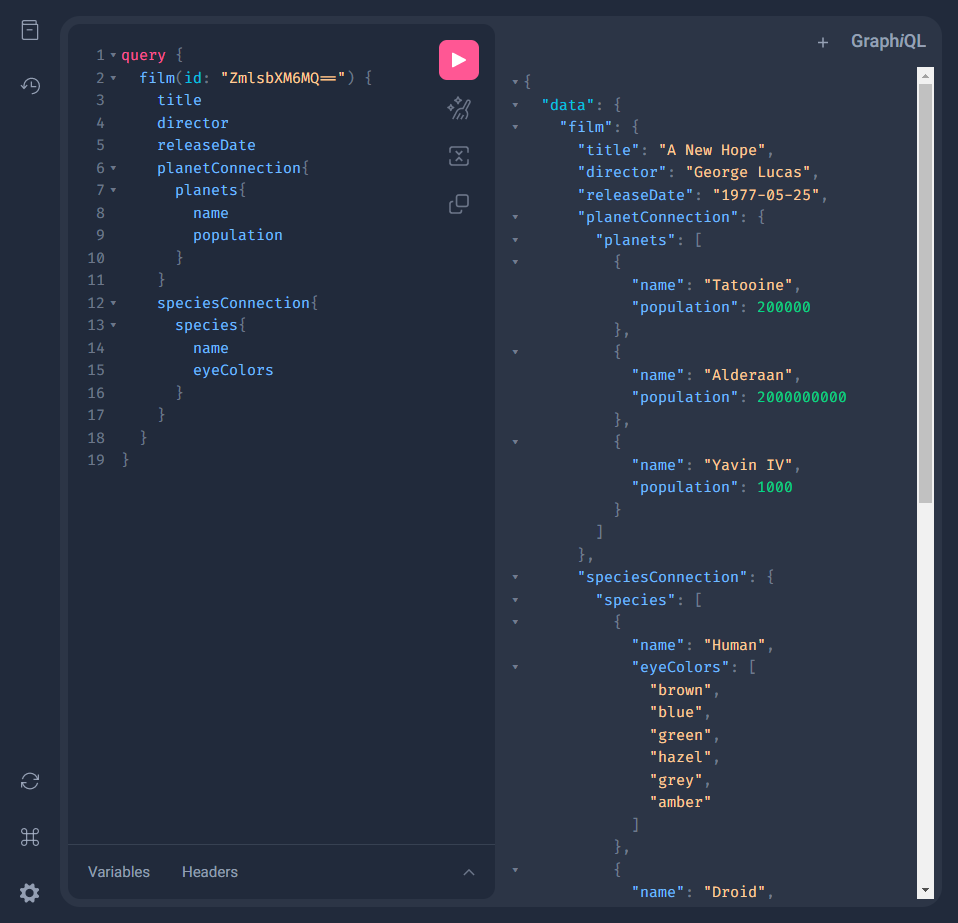
Explorer
Gatsby
프로젝트를 실행(dev
or start
)하면 ___graphql
링크를 받는다.
- 접속하면 개발자가 실행할 수 있는 쿼리 목록인
Explorer
가 있다.
- 쿼리 목록은 해당 프로젝트가 보유 중인 데이터이다.
- 클릭으로 손쉽게 쿼리를 작성된다.
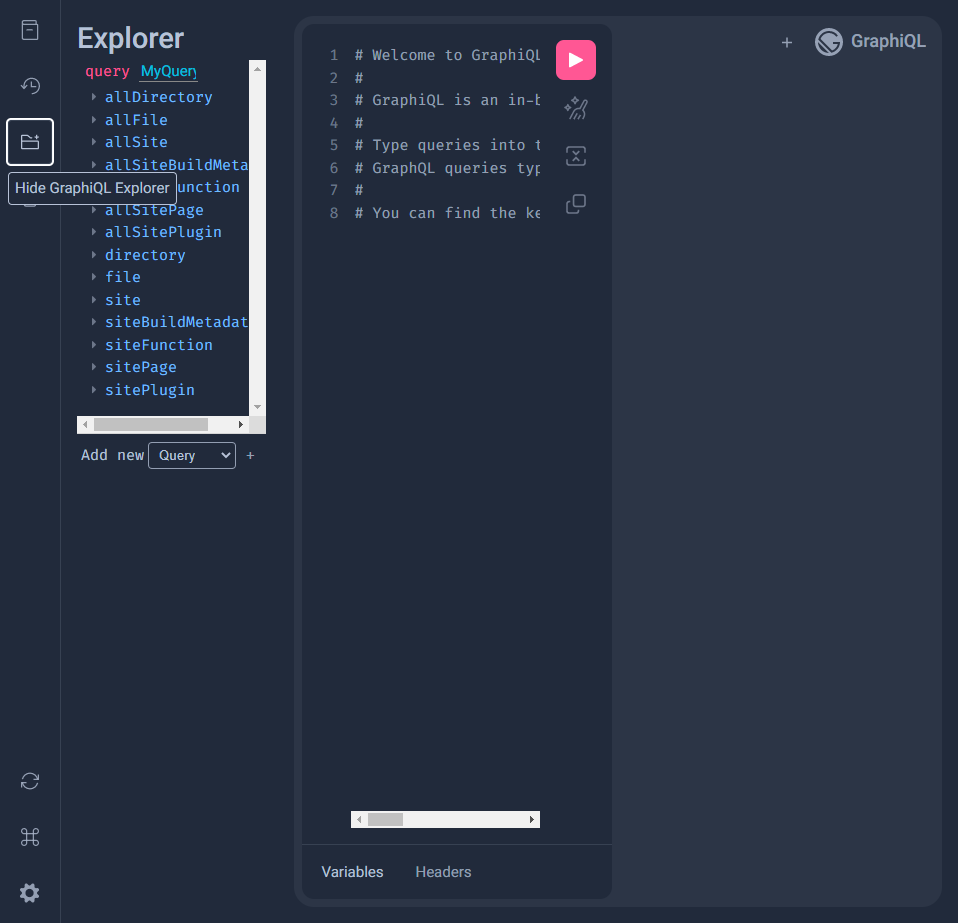
- 프로젝트 사이트에 대한 정보는 목록의
site
를 쿼리로 보내 확인할 수 있다.
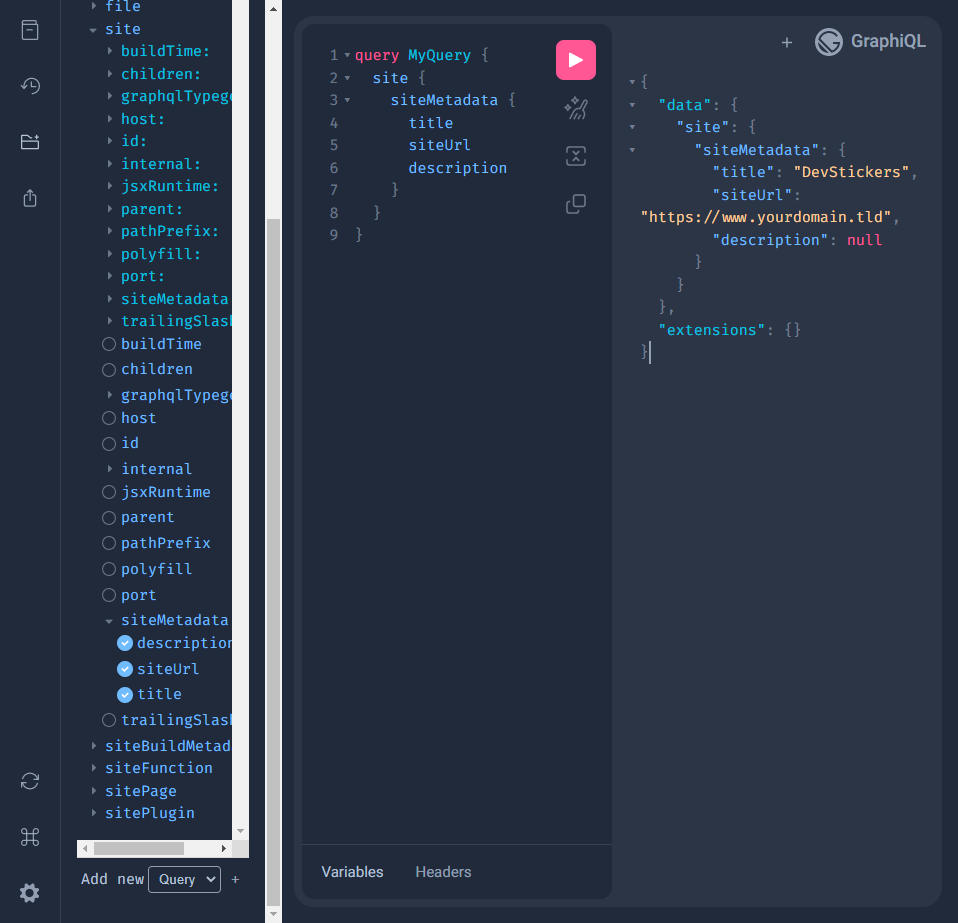
site
쿼리의 데이터는 Gatsby
프로젝트의 gatsby-config.ts
에서 가져온다.
import type { GatsbyConfig } from "gatsby";
const config: GatsbyConfig = {
siteMetadata: {
title: `DevStickers`,
siteUrl: `https://www.yourdomain.tld`,
},
graphqlTypegen: true,
plugins: [],
};
export default config;
config
내에 siteMetadata
가 있고, 그 안에 title
과 siteUrl
이 존재한다. description
은 없기 때문에 쿼리의 결과는 null
이다.
useStaticQuery
Gatsby
에서 쿼리 데이터를 가져올 때 useStaticQuery
를 사용한다.
import { graphql, useStaticQuery } from "gatsby";
import React from "react";
interface HeadProps {
title: string;
}
export default function Head({ title }: ISeoProps) {
const data = useStaticQuery<Queries.HeadDataQuery>(graphql`
query HeadData {
site {
siteMetadata {
title
}
}
}
`);
return <title>{title} | {data.site?.siteMetadata?.title}!</title>;
}
type HeadDataQueryVariables = Exact<{ [key: string]: never; }>;
type HeadDataQuery = { readonly site: { readonly siteMetadata: { readonly title: string | null } | null } | null };
- 쿼리의 타입은
config
파일의 graphqlTypegen
에 따른다.
- 값이
true
라면 Gatsby
가 쿼리를 읽고 자동으로 맞는 타입을 생성한다.
gatsby-types.d.ts
의 맨 아래에 해당 타입이 새로 생성된 것을 확인할 수 있다.
build
시 쿼리 데이터가 작성된 정적 페이지를 생성한다.
PageQuery
Gatsby
가 제공하는 plugin library
에서 source-filesytem
를 설치한다.
Gatsby
프로젝트가 개발자의 파일을 관찰할 수 있게 허용하는 라이브러리이다.
- 설치 :
npm install gatsby-source-filesystem
config
파일에 plugin
설정을 추가한다.
import type { GatsbyConfig } from "gatsby";
const config: GatsbyConfig = {
plugins: [
{
resolve: `gatsby-source-filesystem`,
options: {
path: `${__dirname}/blog-posts`,
},
}
],
};
export default config;
/blog-posts
폴더에 Hello.md
, ByeBye.md
두 파일을 만들었다면, 파일의 이름을 불러올 수 있다.
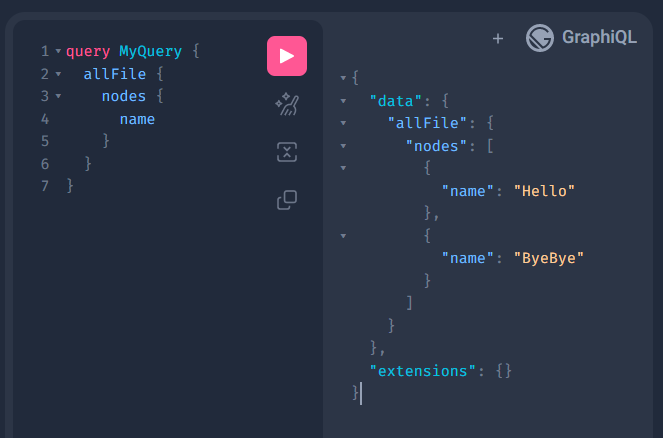
query
- 다른 파일을 불러올 페이지 컴포넌트에서 간단하게
query
를 export
하여 데이터를 받을 수 있다.
const Blog = ({ data }:PageProps<Queries.BlogTitlesQuery>) => {
console.log(data);
return (
{data.allFile.nodes.map((title) => (
<h3 key={title.name}>{title.name}</h3>
))}
);
};
export default Blog;
export const query = graphql`
query BlogTitles {
allFile {
nodes {
name
}
}
}
`;
참고
노마드코더 - 리액트JS 마스터 클래스
GraphQL Learn