예외 처리
- 런티임중에 자바 프로그램이 에러를 맞이했을 때, 이를 처리해줌으로써 프로그램의 비정상적인 종료를 방지할 수 있음
- 예외 처리시에는 try-catch-finally문을 사용
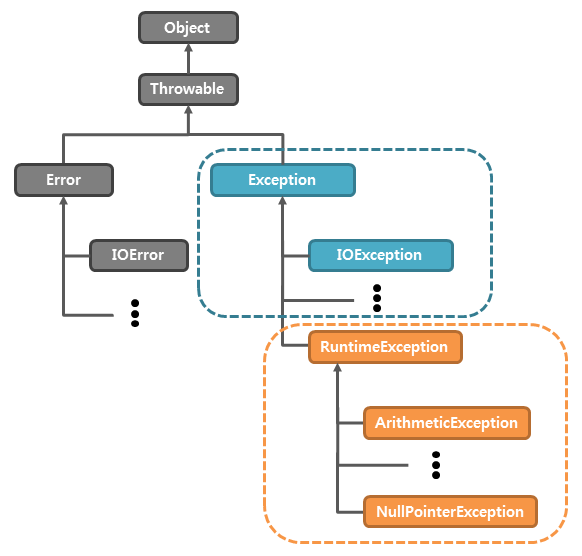
- 실행중 큰 문제가 발생하지 않는 RuntimeException 이외의 예외가 발생할 수 있는 구문에는 반드시 예외 처리를 해주어야 함
byte[] list = {'a', 'b', 'c'};
try {
System.out.write(list);
} catch (IOException e) {
e.printStackTrace();
}
- catch문은 여러개 중첩이 가능하나, 먼저 등장하는 catch문부터 검사하기 때문에 catch블록의 순서에 주의해야 함
try {
System.out.write(list);
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
- 여러개의 예외를 동시에 처리하고자 하는 경우 | 기호를 사용 가능
try {
this.db.commit();
} catch (IOException | SQLException e) {
e.getMessage();
}
예외 발생시키기
- 예외를 직접 발생시키고자 하는 경우 throw문 사용
public class Exception03 {
static void handlingException() {
try {
throw new Exception();
} catch (Exception e) {
System.out.println("error in method");
}
}
public static void main(String[] args) {
System.out.println("error in main");
}
}
public class Exception04 {
static void handlingException() throws Exception { throw new Exception(); }
public static void main(String[] args) {
try {
handlingException();
} catch (Exception e) {
System.out.println("error in main");
}
}
}
- 만약 예외 클래스가 필요하다면 Exception, 또는 RuntimeException 클래스를 상속해 새로운 예외 클래스를 만들 수 있음
예외처리를 강제하지 않는 RuntimeException를 더 많이 사용함
class MyException extends RuntimeException {
MyException(String errMsg) {
super(errMsg);
}
}
try-with-resources문
- Java 7부터는 사용한 자원을 자동으로 해제해 주는 try-with-resources문을 사용할 수 있음
- try문에 자원을 할당하는 명령문 또는 파일을 읽는 명령문을 명시하면, try블럭 종료 즉시 파일을 닫거나 자원 할당을 해제해줌
static String readFile(String filePath) throws IOException {
BufferedReader br = new BufferedReader(new FileReader(filePath));
try {
return br.readLine();
} finally {
if (br != null)
br.close();
}
}
static String readFile(String filePath) throws IOException {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
return br.readLine();
}
}
출처:
http://www.tcpschool.com/java/java_exception_intro