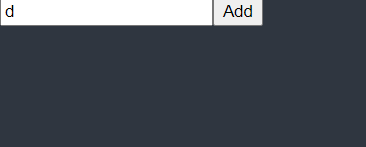
function ToDoList() {
const [toDo, setToDo] = useState("");
const onChange = (event: React.FormEvent<HTMLInputElement>) => {
const {
currentTarget: { value },
} = event;
setToDo(value);
};
const onSubmit = (event: React.FormEvent<HTMLFormElement>) => {
event.preventDefault();
console.log(toDo);
};
return (
<div>
<form onSubmit={onSubmit}>
<input onChange={onChange} value={toDo} placeholder="Write a to do" />
<button>Add</button>
</form>
</div>
);
}
const { register, handleSubmit, setValue } = useForm<IForm>();
const handleValid = (data: IForm) => {
console.log("add to do", data.toDo);
setValue("toDo", "");
};
<form onSubmit={handleSubmit(handleValid)}>
<input
{...register("toDo", {
required: "Please write a To Do",
})}
placeholder="Write a to do"
/>
<button>Add</button>
</form>
- handleSubmit
-첫번째 인자(필수): 데이터가 유효할 때 호출되는 함수
-두번째 인자(필수X): 데이터가 유효하지 않을 때 호출되는 함수
- input 유효성 검사
-<input {...register("toDo",{required: true, minLength: 10})} />
- formState으로 에러메세지 출력
const {
register,
handleSubmit,
setError,
formState: { errors },
} = useForm<IForm>({
defaultValues: {
email: "@naver.com",
},
});
<input
{...register("email", {
required: "Email is required",
pattern: {
value: /^[A-Za-z0-9._%+-]+@naver.com$/,
message: "Only naver.com emails allowed",
},
})}
placeholder="Email"
/>
<span>{errors?.email?.message}</span>
- setError 발생하는 문제에 따라 추가적으로 에러를 설정할 수 있게 도와줌
const onValid = (data: IForm) => {
if (data.password !== data.password1) {
setError(
"password1",
{ message: "Password are not the same" },
{ shouldFocus: true }
);
}
setError("extraError", { message: "Server offline." });
};
<input
{...register("password", { required: "write here", minLength: 5 })}
placeholder="Password"
/>
<span>{errors?.password?.message}</span>
<input
{...register("password1", {
required: "Password is required",
minLength: {
value: 5,
message: "Your password is too short.",
},
})}
placeholder="Password1"
/>
<span>{errors?.password1?.message}</span>
<span>{errors?.extraError?.message}</span>
- validate 에러메세지 사용
<input
{...register("firstName", { required: "write here" })}
{...register("firstName", {
required: "write here",
validate: {
noNico: (value) =>
value.includes("nico") ? "no nicos allowed" : true,
noNick: (value) =>
value.includes("nick") ? "no nick allowed" : true,
},
})}
placeholder="First Name"
/>