DatabaseConfig 코드
import org.springframework.boot.jdbc.DataSourceBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
@Configuration
public class DetabaseConfig {
@Bean
public DataSource dataSource() {
DataSourceBuilder dataSourceBuilder = DataSourceBuilder.create();
dataSourceBuilder.driverClassName("com.mysql.cj.jdbc.Driver");
dataSourceBuilder.username("root");
dataSourceBuilder.password("");
dataSourceBuilder.url("jdbc:mysql://localhost:3306/DB이름?serverTimezone=Asia/Seoul&useUnicode=true&characterEncoding=UTF-8");
return dataSourceBuilder.build();
}
}
User Model 코드
import lombok.*;
import javax.persistence.*;
@Setter
@Getter
@NoArgsConstructor(access= AccessLevel.PROTECTED)
@Table(name="user")
@Entity
public class User {
@Id
@GeneratedValue(strategy= GenerationType.IDENTITY)
private int id;
@Column(nullable=false, length=30)
private String username;
@Column(nullable=false, length=100)
private String password;
@Builder
public User(int id, String username, String password) {
this.id=id;
this.username=username;
this.password=password;
}
}
application.yml
server:
port: 8080
spring:
application:
name: springbootstart
datasource:
url: jdbc:mysql://localhost:3306/DB이름?serverTimezone=Asia/Seoul&useUnicode=true&characterEncoding=UTF-8
username: root
password:
jpa:
show-sql: true
hibernate:
ddl-auto: update
properties:
dialect: org.hibernate.dialect.MySQL5InnoDBDialect
build.gradle 코드
dependencies {
implementation 'org.springframework.boot:spring-boot-starter'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
implementation 'org.springframework.boot:spring-boot-starter-web'
// Entity 사용 가능하게 하는 거
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
compileOnly 'org.projectlombok:lombok'
annotationProcessor 'org.projectlombok:lombok'
runtimeOnly 'mysql:mysql-connector-java'
}
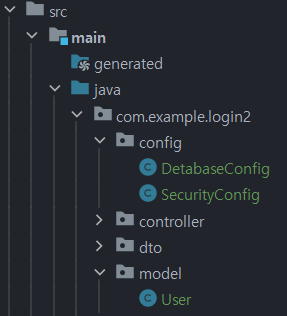