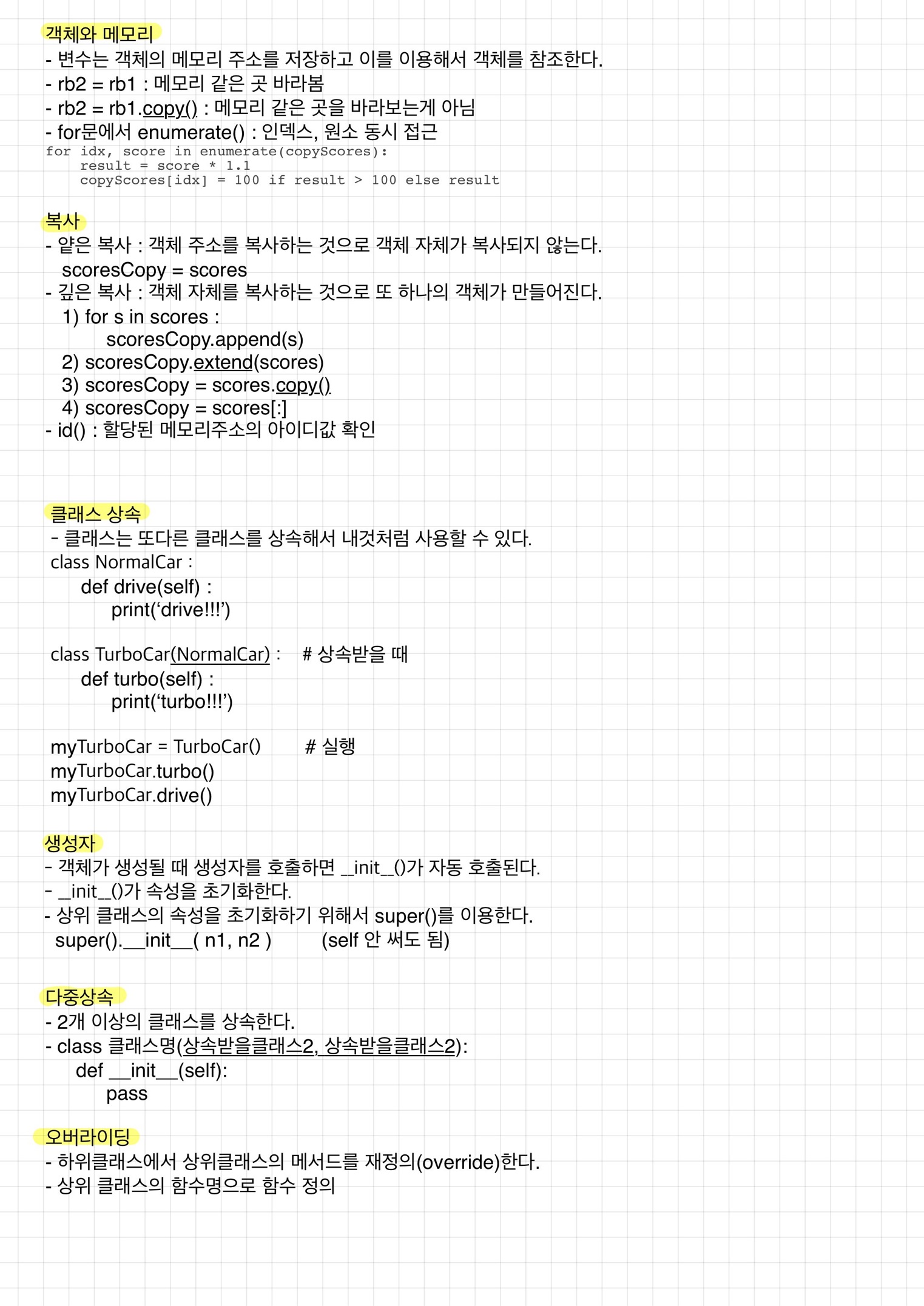
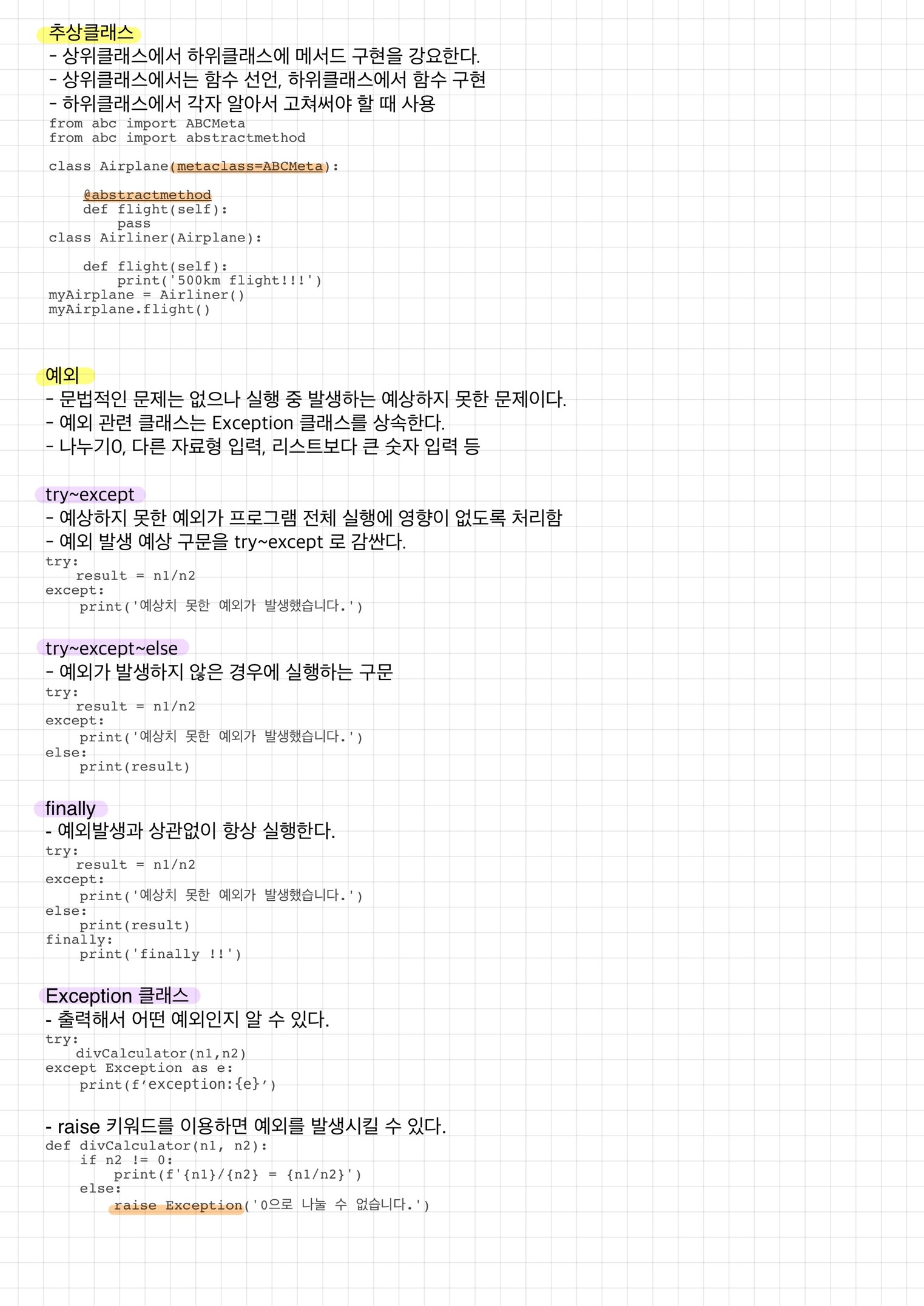
score = [8.7, 9.1, 8.9, 7.9, 9.0]
scoreCopy = []
score.sort()
scoreCopy = score.copy()
scoreCopy.pop(0)
scoreCopy.pop()
print('score : {}'.format(score))
print('scoreCopy : {}'.format(scoreCopy))
print('score 평균 : %.2f' %(sum(score) / len(score)))
print('최저, 최고값 뺀 평균 : %.2f' %(sum(scoreCopy) / len(scoreCopy)))
print('평균 차이 : %.2f' %(sum(score) / len(score) - sum(scoreCopy) / len(scoreCopy)))
class Calculator:
def add(self, n1, n2):
print(n1 + n2)
def sub(self, n1, n2):
print(n1 - n2)
class CalculatorMath(Calculator):
def mul(self, n1, n2):
print(n1 * n2)
def div(self, n1, n2):
print(n1 / n2)
myCalculator = CalculatorMath()
myCalculator.add(10,20)
myCalculator.sub(10,20)
myCalculator.mul(10,20)
myCalculator.div(10,20)
class MidScore:
def __init__(self, mNum1, mNum2, mNum3):
print('[MidScore] __init__')
self.mid_kor = mNum1
self.mid_eng = mNum2
self.mid_mat = mNum3
class FinalScore(MidScore):
def __init__(self, mNum1, mNum2, mNum3, fNum1, fNum2, fNum3):
print('[FinalScore] __init__')
super().__init__(mNum1, mNum2, mNum3)
self.fin_kor = fNum1
self.fin_eng = fNum2
self.fin_mat = fNum3
def printScores(self):
print('중간고사 국어 : {}'.format(self.mid_kor))
print('중간고사 영어 : {}'.format(self.mid_eng))
print('중간고사 수학 : {}'.format(self.mid_mat))
print('기말고사 국어 : {}'.format(self.fin_kor))
print('기말고사 영어 : {}'.format(self.fin_eng))
print('기말고사 수학 : {}'.format(self.fin_mat))
def printTotalSum(self):
totalSum = self.mid_kor + self.mid_eng + self.mid_mat +\
self.fin_kor + self.fin_eng + self.fin_mat
print('총점 : %d' %totalSum)
def printTotalAvg(self):
totalAvg = (self.mid_kor + self.mid_eng + self.mid_mat +
self.fin_kor + self.fin_eng + self.fin_mat) / 6
print('총 평균 : %.2f' %(totalAvg))
myScores = FinalScore(100,90,100,80,100,95)
myScores.printScores()
myScores.printTotalSum()
myScores.printTotalAvg()
class Robot:
def __init__(self, w, h):
self.width = w
self.height = h
def printRobotInfo(self):
print(f'가로 : {self.width}')
print(f'세로 : {self.height}')
def fire(self):
print('미사일 발사!')
class NewRobot(Robot):
def __init__(self, w, h):
super().__init__()
def fire(self):
print('레이저 발사!')
myRobot = NewRobot(200,300)
myRobot.printRobotInfo()
myRobot.fire()
eveList=[]; oddList=[]; floatList=[]; dataList=[]
n=1
while n<6:
try:
data = input('number : ')
num = float(data)
except:
print('숫자를 입력하세요')
continue
else:
if num-int(num) != 0 :
floatList.append(num)
else:
num = int(num)
if num%2==0:
eveList.append(num)
else:
oddList.append(num)
n+=1
finally:
dataList.append(data)
print(f'실수 : {floatList}')
print(f'짝수 : {eveList}')
print(f'홀수 : {oddList}')
print(f'입력값 : {dataList}')