chip
Chip(
avatar: CircleAvatar(
backgroundColor: Colors.grey.shade800,
child: const Text('AB'),
),
label: const Text('Aaron Burr'),
)
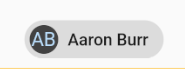
ConstrainedBox 크기 고정 + 제한
ConstrainedBox(
constraints: const BoxConstraints.expand(),
child: const Card(child: Text('Hello World!')),
)
CupertinoPicker
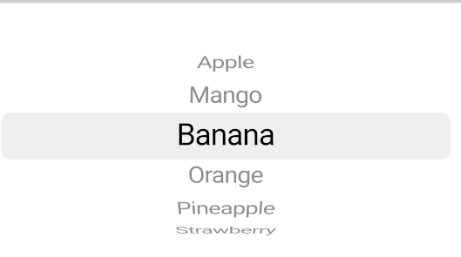
import 'package:flutter/cupertino.dart';
const double _kItemExtent = 32.0;
const List<String> _fruitNames = <String>[
'Apple',
'Mango',
'Banana',
'Orange',
'Pineapple',
'Strawberry',
];
void main() => runApp(const CupertinoPickerApp());
class CupertinoPickerApp extends StatelessWidget {
const CupertinoPickerApp({super.key});
@override
Widget build(BuildContext context) {
return const CupertinoApp(
theme: CupertinoThemeData(brightness: Brightness.light),
home: CupertinoPickerExample(),
);
}
}
class CupertinoPickerExample extends StatefulWidget {
const CupertinoPickerExample({super.key});
@override
State<CupertinoPickerExample> createState() => _CupertinoPickerExampleState();
}
class _CupertinoPickerExampleState extends State<CupertinoPickerExample> {
int _selectedFruit = 0;
void _showDialog(Widget child) {
showCupertinoModalPopup<void>(
context: context,
builder: (BuildContext context) => Container(
height: 216,
padding: const EdgeInsets.only(top: 6.0),
margin: EdgeInsets.only(
bottom: MediaQuery.of(context).viewInsets.bottom,
),
color: CupertinoColors.systemBackground.resolveFrom(context),
child: SafeArea(
top: false,
child: child,
),
));
}
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text('CupertinoPicker Sample'),
),
child: DefaultTextStyle(
style: TextStyle(
color: CupertinoColors.label.resolveFrom(context),
fontSize: 22.0,
),
child: Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
const Text('Selected fruit: '),
CupertinoButton(
padding: EdgeInsets.zero,
onPressed: () => _showDialog(
CupertinoPicker(
magnification: 1.22,
squeeze: 1.2,
useMagnifier: true,
itemExtent: _kItemExtent,
onSelectedItemChanged: (int selectedItem) {
setState(() {
_selectedFruit = selectedItem;
});
},
children:
List<Widget>.generate(_fruitNames.length, (int index) {
return Center(
child: Text(
_fruitNames[index],
),
);
}),
),
),
child: Text(
_fruitNames[_selectedFruit],
style: const TextStyle(
fontSize: 22.0,
),
),
),
],
),
),
),
);
}
}
CupertinoTimerPicker
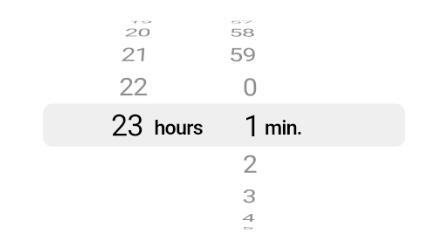
import 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'package:village/view/widgets/test/cupertino_time_picker.dart';
void main() {
runApp(
const ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
),
debugShowCheckedModeBanner: false,
home: const TimerPickerApp(),
);
}
}
showDatePicker function
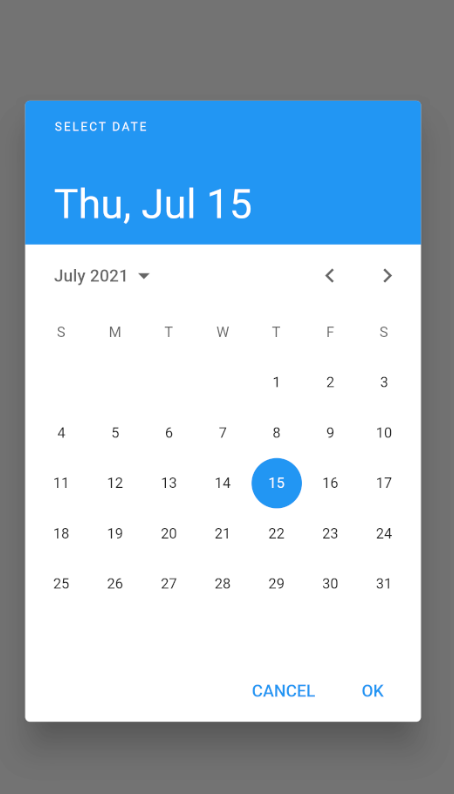
import 'package:flutter/material.dart';
class DatePicker extends StatefulWidget {
const DatePicker({super.key, this.restorationId});
final String? restorationId;
@override
State<DatePicker> createState() => _DatePickerState();
}
class _DatePickerState extends State<DatePicker> with RestorationMixin {
@override
String? get restorationId => widget.restorationId;
final RestorableDateTime _selectedDate =
RestorableDateTime(DateTime(2021, 7, 25));
late final RestorableRouteFuture<DateTime?> _restorableDatePickerRouteFuture =
RestorableRouteFuture<DateTime?>(
onComplete: _selectDate,
onPresent: (NavigatorState navigator, Object? arguments) {
return navigator.restorablePush(
_datePickerRoute,
arguments: _selectedDate.value.millisecondsSinceEpoch,
);
},
);
static Route<DateTime> _datePickerRoute(
BuildContext context,
Object? arguments,
) {
return DialogRoute<DateTime>(
context: context,
builder: (BuildContext context) {
return DatePickerDialog(
restorationId: 'date_picker_dialog',
initialEntryMode: DatePickerEntryMode.calendarOnly,
initialDate: DateTime.fromMillisecondsSinceEpoch(arguments! as int),
firstDate: DateTime(2021),
lastDate: DateTime(2022),
);
},
);
}
@override
void restoreState(RestorationBucket? oldBucket, bool initialRestore) {
registerForRestoration(_selectedDate, 'selected_date');
registerForRestoration(
_restorableDatePickerRouteFuture, 'date_picker_route_future');
}
void _selectDate(DateTime? newSelectedDate) {
if (newSelectedDate != null) {
setState(() {
_selectedDate.value = newSelectedDate;
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text(
'Selected: ${_selectedDate.value.day}/${_selectedDate.value.month}/${_selectedDate.value.year}'),
));
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: OutlinedButton(
onPressed: () {
_restorableDatePickerRouteFuture.present();
},
child: const Text('Open Date Picker'),
),
),
);
}
}