이 시리즈는 fragment shader의 내용을 소개합니다.
fragment shader는 화면에 보이는 모든 pixel들을 control하게해줍니다.
shader는 화면의 모든 pixel들에게 공통적으로 내려지는 지시이다.
그렇기 때문에 실행될 때 매우 빠르다.
Shader 코드의 특징
void main() { gl_FragColor = vec4(1,0,0,1); // ERROR }
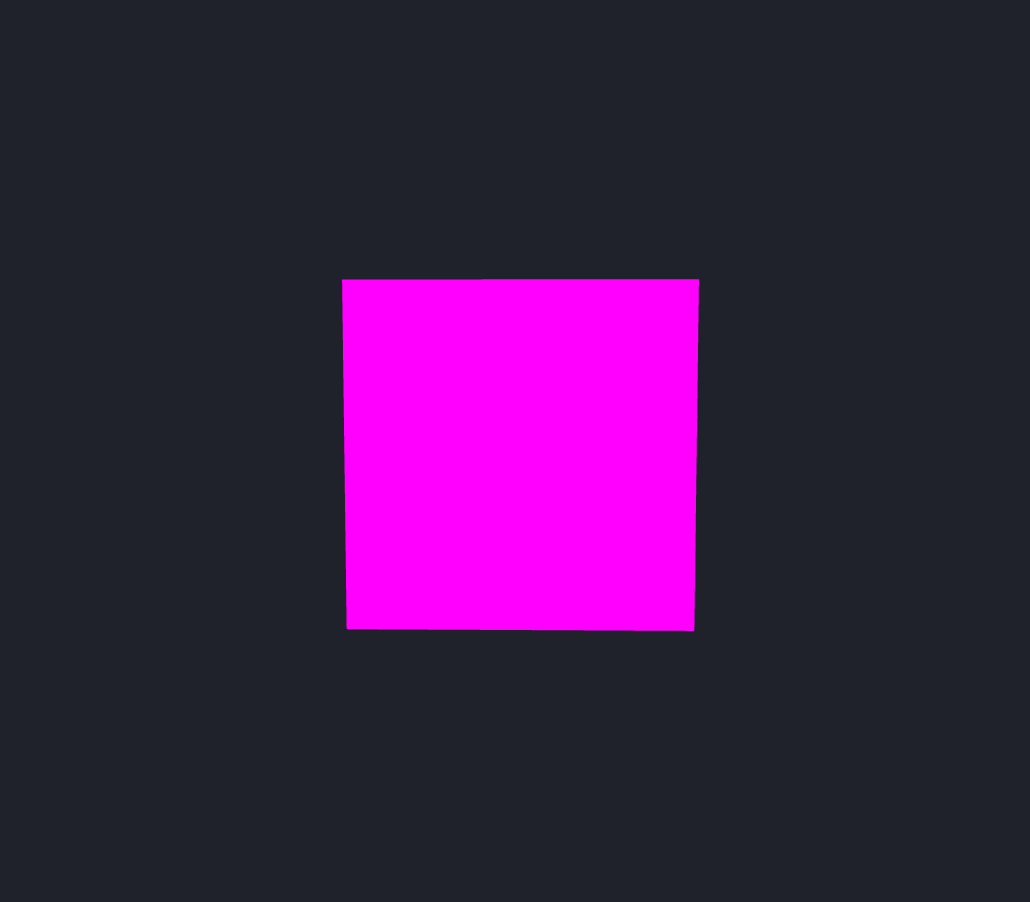
vertex shader
```c
void main(){
vec4 modelPosition = modelMatrix * vec4(position, 1.0);
vec4 viewPosition = viewMatrix * modelPosition;
vec4 projectedPosition = projectionMatrix * viewPosition;
gl_Position = projectedPosition;
}
fragment shader
precision mediump float;
void main() {
gl_FragColor = vec4(1.0,0.0,1.0,1.0);
}
이미지의 각각의 부분들의 색상을 GPU가 할당함에 있어서 CPU가 모든 부분들에게 공통적으로 input을 보낼 때가 있다.
그리고 readonly data로 관리될 필요도 있다.
이러한 문제를 해결해주는 것이 Uniform이다.
#ifdef GL_ES
precision mediump float;
#endif
uniform vec2 u_resolution; // Canvas size (width,height)
uniform vec2 u_mouse; // mouse position in screen pixels
uniform float u_time; // Time in seconds since load
위와 같은 예시로 uniform이 사용된다.
mouse 위치, 시간, canvas 사이즈 등의 데이터를 모든 image의 부분들이 공통적이고 readonly로 input 받기 위해서 사용된다.
CPU와 GPU 사이의 유일한 통로라고 생각하면 쉽다.
uniform의 타입의 종류
GLSL 제공하는 함수
GLSL의 기본적인 output은 gl_FragColor이다.
그리고 기본적인 input은 gl_FragCoord이다.
gl_FragCoord는 이미지에서의 pixel의 coordination을 의미한다.
이는 각각의 부분들 마다 다른 varying이라고 부른다.