pygame.init() # 2.pygame 초기화
WHITE = (255, 255, 255)
size = [400, 300]
screen = pygame.display.set_mode(size)
done = False
clock = pygame.time.Clock()
airplane = pygame.image.load('images/plane.png')
airplane = pygame.transform.scale(airplane, (60, 45))
def runGame():
global done, airplane
x = 20
y = 24
while not done:
clock.tick(10)
screen.fill(WHITE)
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 방향키 입력에 대한 이벤트 처리
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
y -= 10
elif event.key == pygame.K_DOWN:
y += 10
screen.blit(airplane, (x, y))
pygame.display.update()
runGame()
pygame.quit()
똑같은 코드를 붙여넣으면 실행이 되고, 내가 똑같이 타이핑하면 실행은 되는데 캐릭터가 움직이지 않는다.
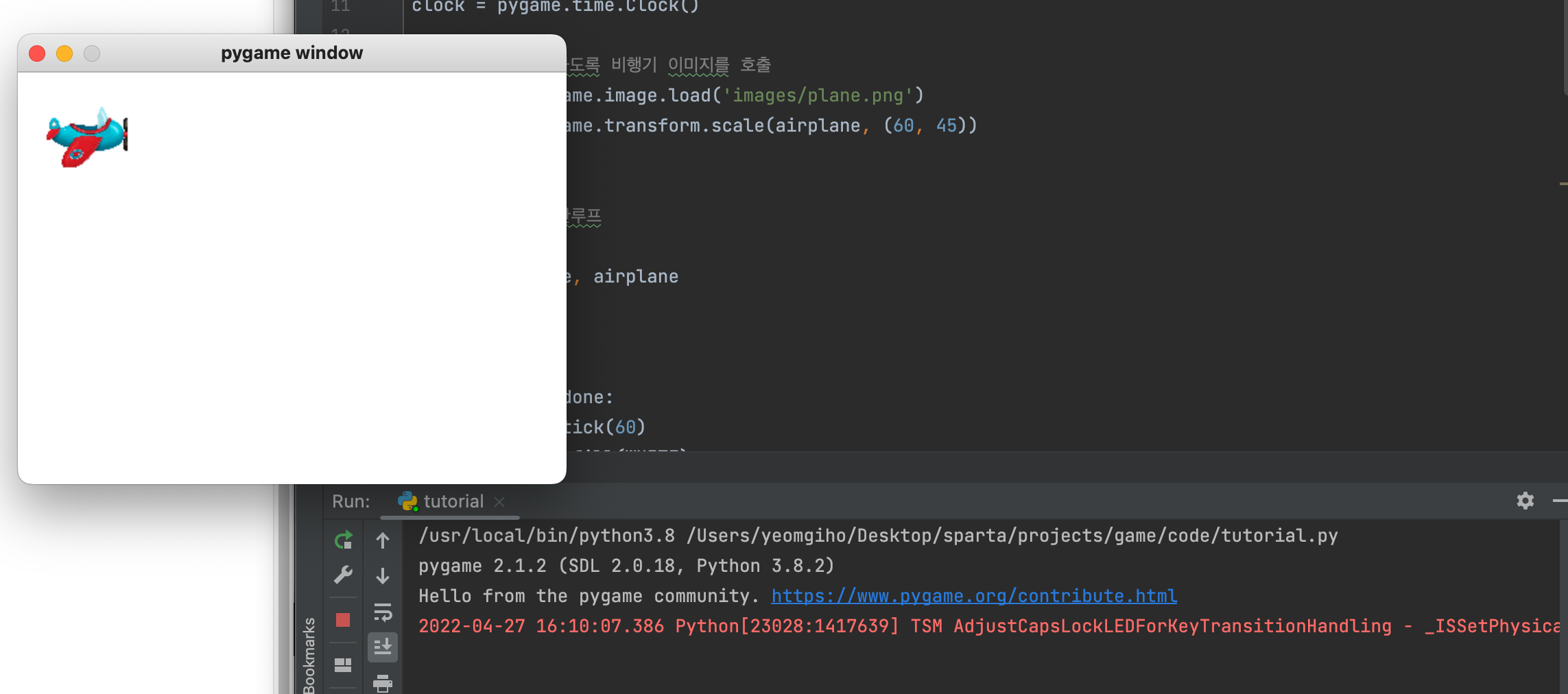
<h2> 개인 프로젝트 </h2>
이번 프로젝트는 실행되는 게임을 만드는 것이었다. 파이썬 문법 공부를 한다고 시간이 다소 부족한 감이 있지만 지속적으로 업데이트 할 계획이다.
<h4> 현재 구현 한 것 : </h4>
- 배경, 뱀, 사과 지정
- 뱀이 0.1초마다 머리가 향하는 방향으로 자동 이동 되도록 함
- 뱀이 사과를 먹으면 꼬리가 한 칸 길어지도록 함
- 뱀이 본인 몸에 부딪히면 게임 종료
<h4> 추가로 구현해 보고자 하는 것 </h4>
- 뱀이 벽에 부딪히면 게임 종료
- 난이도 설정
- 점수가 상단에 보이도록
- 컴퓨터와 상대로 게임을 할 수 있게 (실행 시 창이 2개 뜨도록)
- 멀티플레이
- 보다 부드러운 동작
<h3> 게임 코드 </h3>
이것이 snake 게임 결과 코드이다. 오류가 없는데 계속 실행이 되지 않아서 무엇이 문제인가 한참 고민했다.
import pygame
import random
from datetime import datetime
from datetime import timedelta
pygame.init()
GRASS = (114, 183, 106)
GREEN = (172, 206, 94)
RED = (233, 67, 37)
BLUE = (88, 125, 255)
size = [500, 500]
screen = pygame.display.set_mode(size)
done = False
clock = pygame.time.Clock()
last_moved_time = datetime.now()
KEY_DIRECTION = {
pygame.K_UP: 'N',
pygame.K_DOWN: 'S',
pygame.K_LEFT: 'W',
pygame.K_RIGHT: 'E'
}
def draw_block(screen, color, position):
block = pygame.Rect((position[1] 20, position[0] 20), (20, 20))
pygame.draw.rect(screen, color, block)
#뱀 클래스
class Snake:
def init(self):
self.positions = [(2, 0), (1, 0), (0, 0)] # 뱀의 위치, (2, 0)이 머리
self.direction = ''
def draw(self):
for position in self.positions:
draw_block(screen, BLUE, position)
def move(self):
head_position = self.positions[0]
y, x = head_position
if self.direction == 'N':
self.positions = [(y - 1, x)] + self.positions[:-1]
elif self.direction == 'S':
self.positions = [(y + 1, x)] + self.positions[:-1]
elif self.direction == 'W':
self.positions = [(y, x - 1)] + self.positions[:-1]
elif self.direction == 'E':
self.positions = [(y, x + 1)] + self.positions[:-1]
# 뱀의 길이를 늘려 주는 grow()함수 호출
def grow(self):
tail_position = self.positions[-1] #[(2,0),(1,0),(0,0)]일 경우 (0,0)을 의미함
x, y = tail_position
if self.direction == "N":
# positons.append()는 추가하는 값 뒤에 추가된다.
# positions가 [(2, 0), (1, 0), (0, 0)] 라면, positions.append((10, 20))의 결과는 [(2, 0), (1, 0), (0, 0), (10, 20)]이 된다.
self.positions.append((y - 1, x))
elif self.direction == 'S':
self.positions.append((y + 1, x))
elif self.direction == 'W':
self.positions.append((y, x - 1))
elif self.direction == 'E':
self.positions.append((y, x + 1))
#사과 클래스 : 사과가 랜덤 위치에 생성된다
class Apple:
def init(self, position=(5, 5)):
self.position = position
def draw(self):
draw_block(screen, RED, self.position)
def runGame():
global done, last_moved_time
# 게임 시작시 뱀과 사과를 초기화, 뱀과 사과 객체를 만듦
snake = Snake()
apple = Apple()
while not done:
clock.tick(10)
screen.fill(GREEN)
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
if event.type == pygame.KEYDOWN:
if event.key in KEY_DIRECTION: # 키보드 입력값을 이용해 뱀의 이동방향 지정
snake.direction = KEY_DIRECTION[event.key]
if timedelta(seconds=0.1) <= datetime.now() - last_moved_time: # 시간 검증을 통해 0.1초마다 뱀 이동
snake.move()
last_moved_time = datetime.now()
# 뱀이 사과에 닿은 것을 확인
if snake.positions[0] == apple.position:
snake.grow()
apple.position = (random.randint(0, 19), random.randint(0, 19))
# 뱀 충돌 처리
if snake.positions[0] in snake.positions[1:]:
done = True
# 뱀과 사과를 게임판에 그려줌
snake.draw()
apple.draw()
pygame.display.update()
runGame()
pygame.quit()
<h4> 동작 화면 </h4>
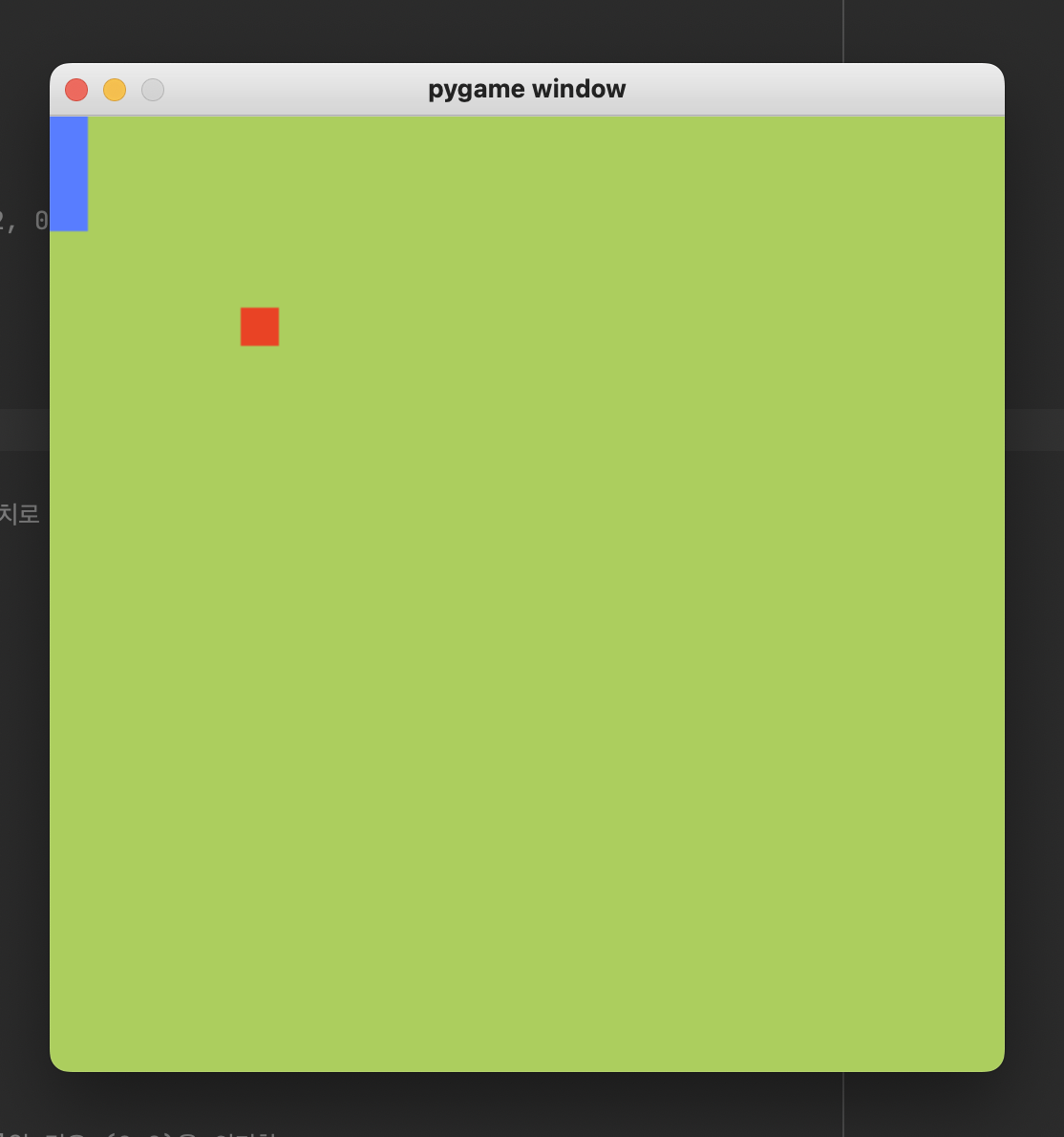
<h4> 확인 결과 </h4>
if self.direction == 'N':
self.positions = [(y - 1, x)] + self.positions[:-1] # 여기의 = 부분을 == 으로 작성함
결국 내 잘못이다. 오류가 없더라도 문법 오탈자가 있을 수 있으니 반드시 확인할 것!
<h3> 프로젝트 한 뒤의 회고 </h3>
- 주어진 시간에 최대한 결과를 도출하기위해 노력해보기
- 다른 사람들의 결과에 비해 다소 부족함을 인정하고 더욱 발전시켜보기
- 배경음악을 넣는 것은 생각도 못함
- 이동하는 물체를 누끼따서 진행해보기
- 할 수 있다!
reference
참고 url : https://ai-creator.tistory.com/522
https://nadocoding.tistory.com/8?category=902276
파이썬 문법 배우고 처음 만들어보는 게임이라 힘드셨을수도 있을텐데 스네이크 기존게임을 분석하면서 이해하려고 하면서 파이썬 문법에도 익숙해지셨을거에요!
미완성 기능은 git으로 관리하면서 예외처리, 리팩토링을 적용해보면서 틈틈히 유지보수해보시면 좋을것 같아요.
짧은기간 동안 파이썬 문법배우고 게임개발 하시느라 정말 수고많으셨습니다.!