구상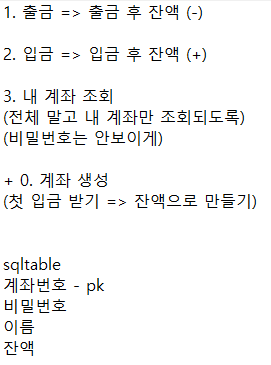
메인 클래스
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
System.out.println("원하시는 서비스를 선택해주세요.");
while (true) {
Scanner scanner = new Scanner(System.in);
System.out.println("1. 계좌 생성 \t 2. 출금 \t 3. 입금 \t 4. 내 계좌 조회하기");
System.out.print("번호 선택 : ");
int code = scanner.nextInt();
if (code == 1) {
System.out.print("생성할 계좌 번호를 입력해주세요. (8자리) : ");
int account = scanner.nextInt();
System.out.print("\n계좌 비밀번호를 입력해주세요. (4자리 숫자) : ");
int pw = scanner.nextInt();
System.out.print("\n사용자의 이름을 입력해주세요. : ");
String name = scanner.next();
System.out.print("\n계좌를 이용하시려면 10,000원 이상 입금하셔아햡니다."
+ "\n입금하실 금액을 입력해주세요. : ");
int balance = scanner.nextInt();
CreateAccount createAccount
= new CreateAccount(account, pw, name, balance);
createAccount.mtdCreate();
break;
} else if (code == 2) {
System.out.print("계좌번호를 입력해주세요. : ");
int account = scanner.nextInt();
System.out.print("비밀번호를 입력해주세요. : ");
int pw = scanner.nextInt();
System.out.print("출금하실 금액을 입력해주세요. : ");
int balance = scanner.nextInt();
Withdrawal withdrawal = new Withdrawal(account, pw, balance);
withdrawal.mtdWithdrawal();
break;
} else if (code == 3) {
System.out.print("계좌번호를 입력해주세요. : ");
int account = scanner.nextInt();
System.out.print("비밀번호를 입력해주세요. : ");
int pw = scanner.nextInt();
System.out.print("입금하실 금액을 입력해주세요. : ");
int balance = scanner.nextInt();
Deposit deposit = new Deposit(account, pw, balance);
deposit.mtdDeposit();
break;
} else if (code == 4) {
System.out.print("조회하실 계좌번호를 입력해주세요. : ");
int account = scanner.nextInt();
System.out.print("비밀번호를 입력해주세요. : ");
int pw = scanner.nextInt();
ShowAccount showAccount = new ShowAccount(account, pw);
showAccount.mtdShow();
break;
} else {
System.out.println("번호를 확인하시고 다시 입력해주세요.");
}
}
}
}
DB 접속 커스텀 클래스
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class Conn {
Connection conn = null;
public void mtdConn() {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
String url = "jdbc:mysql://localhost:3308/atm?";
url += "useSSL=false&";
url += "serverTimezone=Asia/Seoul&";
url += "useUnicode=true&";
url += "characterEncoding=UTF-8&";
url += "allowPublicKeyRetrieval=true";
String uid = "root";
String upw = "1234";
conn = DriverManager.getConnection(url, uid, upw);
} catch(ClassNotFoundException e){
System.out.println(e.getMessage());
} catch(SQLException e) {
System.out.println(e.getMessage());
}
}
}
계좌 생성 커스텀 클래스
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class CreateAccount extends Conn{
int account;
int pw;
String name;
int balance;
public CreateAccount(int account, int pw, String name, int balance) {
this.account = account;
this.pw = pw;
this.name = name;
this.balance = balance;
}
public void mtdCreate(){
mtdConn();
PreparedStatement pstmt = null;
try {
String sql = "insert into atm values (?, ?, ?, ?)";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, this.account);
pstmt.setInt(2, this.pw);
pstmt.setString(3, this.name);
pstmt.setInt(4, this.balance);
int result = pstmt.executeUpdate();
if (result == 1) {
System.out.println("계좌가 생성되었습니다."
+ "재실행하여 서비스를 이용해주세요.");
} else {
System.out.println("죄송합니다. "
+ "오류가 발생하여 계좌가 생성되지 않았습니다.");
}
pstmt.close();
conn.close();
} catch(SQLException e) {
System.out.println(e.getMessage());
}
}
}
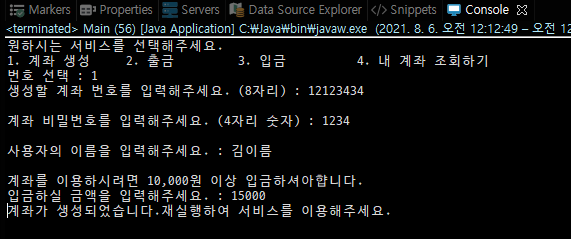
출금 기능 구현 커스텀 클래스
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.text.DecimalFormat;
public class Withdrawal extends Conn{
int account;
int pw;
int balance;
public Withdrawal(int account, int pw, int balance) {
this.account = account;
this.pw = pw;
this.balance = balance;
}
public void mtdWithdrawal() {
mtdConn();
Statement stmt = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement
(ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_UPDATABLE);
String sql = "select pw from atm where account = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, account);
rs = pstmt.executeQuery();
if (rs.next()) {
if (rs.getInt(1) == this.pw) {
String sqlwith = "update atm set balance = balance -? where account = ?";
pstmt = conn.prepareStatement(sqlwith);
pstmt.setInt(1, balance);
pstmt.setInt(2, this.account);
pstmt.executeUpdate();
System.out.println("입력하신 금액이 출금되었습니다.");
System.out.println("\n===== 잔액 =====");
String sqlbal = "select balance from atm where account = " + this.account;
rs = stmt.executeQuery(sqlbal);
int balance = 0;
DecimalFormat decimalFormat = new DecimalFormat("###,###");
rs.beforeFirst();
while(rs.next()) {
balance = rs.getInt("balance");
System.out.println("잔액은 " + decimalFormat.format(balance) + "원 입니다.");
}
}
}
rs.close();
pstmt.close();
stmt.close();
conn.close();
} catch(SQLException e) {
System.out.println(e.getMessage());
}
}
}
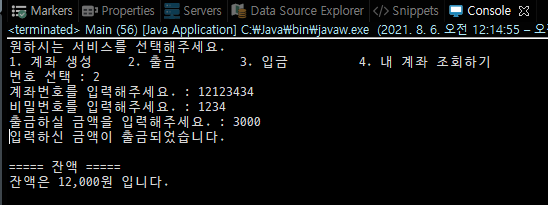
입금 기능 구현 커스텀 클래스
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.text.DecimalFormat;
public class Deposit extends Conn{
int account;
int pw;
int balance;
public Deposit(int account, int pw, int balance) {
this.account = account;
this.pw = pw;
this.balance = balance;
}
public void mtdDeposit() {
mtdConn();
Statement stmt = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement
(ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_UPDATABLE);
String sql = "select pw from atm where account = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, account);
rs = pstmt.executeQuery();
if (rs.next()) {
if (rs.getInt(1) == this.pw) {
String sqlwith = "update atm set balance = balance +? where account = ?";
pstmt = conn.prepareStatement(sqlwith);
pstmt.setInt(1, balance);
pstmt.setInt(2, this.account);
pstmt.executeUpdate();
System.out.println("입력하신 금액이 입금되었습니다.");
System.out.println("\n===== 잔액 =====");
String sqlbal = "select balance from atm where account = " + this.account;
rs = stmt.executeQuery(sqlbal);
int balance = 0;
DecimalFormat decimalFormat = new DecimalFormat("###,###");
rs.beforeFirst();
while(rs.next()) {
balance = rs.getInt("balance");
System.out.println("잔액은 " + decimalFormat.format(balance) + "원 입니다.");
}
}
}
rs.close();
pstmt.close();
stmt.close();
conn.close();
} catch(SQLException e) {
System.out.println(e.getMessage());
}
}
}
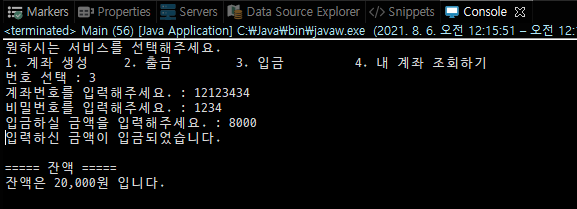
내 계좌 조회 커스텀 클래스
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class ShowAccount extends Conn{
int account;
int pw;
public ShowAccount(int account, int pw) {
this.account = account;
this.pw = pw;
}
public void mtdShow() {
mtdConn();
Statement stmt = null;
PreparedStatement pstmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement
(ResultSet.TYPE_SCROLL_INSENSITIVE, ResultSet.CONCUR_UPDATABLE);
String sql = "select * from atm where account = ?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, account);
rs = pstmt.executeQuery();
System.out.println("계좌번호 \t 이름 \t\t 잔액");
System.out.println("====================================");
while (rs.next()) {
int account = rs.getInt("account");
String name = rs.getString("name");
int balance = rs.getInt("balance");
System.out.println(account + " " + name +
" " + balance);
}
rs.close();
pstmt.close();
stmt.close();
conn.close();
} catch(SQLException e) {
System.out.println(e.getMessage());
}
}
}
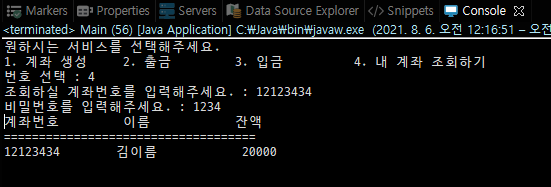