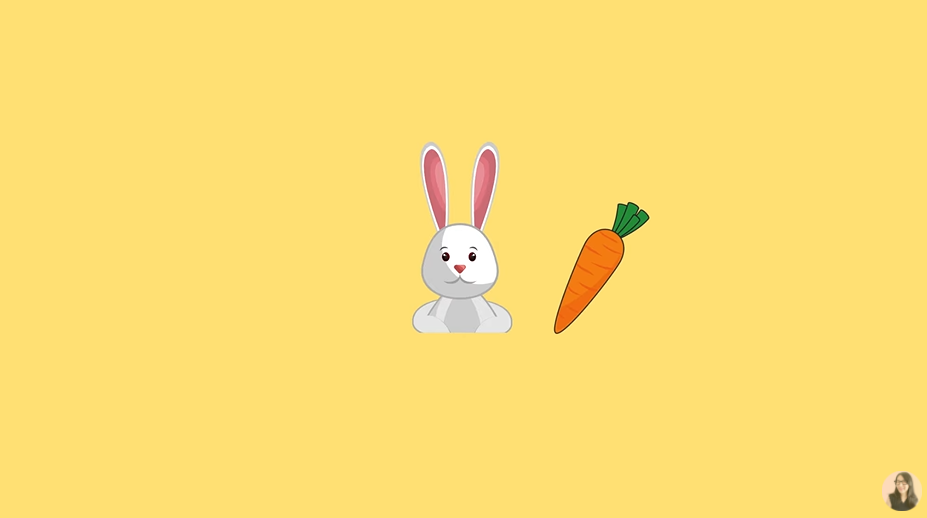
자료구조.
- 어떤 방식으로 어떤 형식으로 담아 놓을 것인지?
Object와 자료구조의 차이
Object
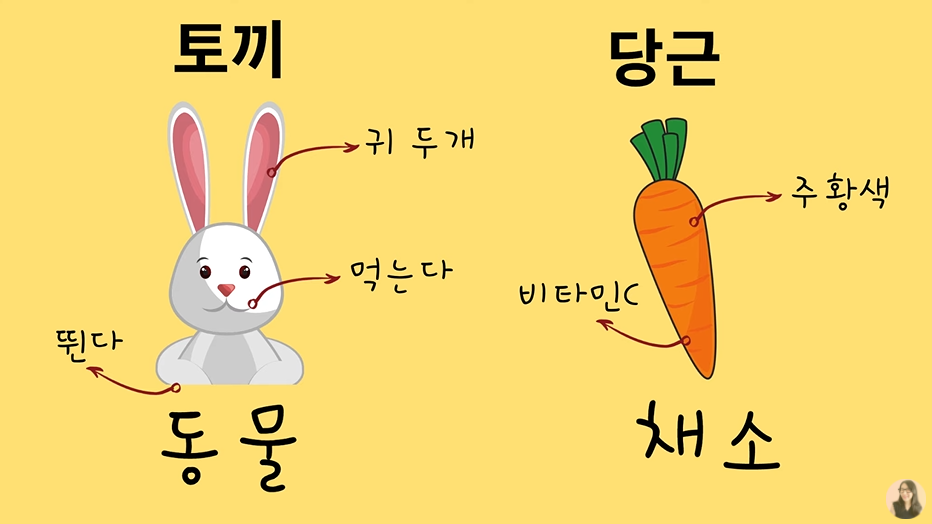
자료구조
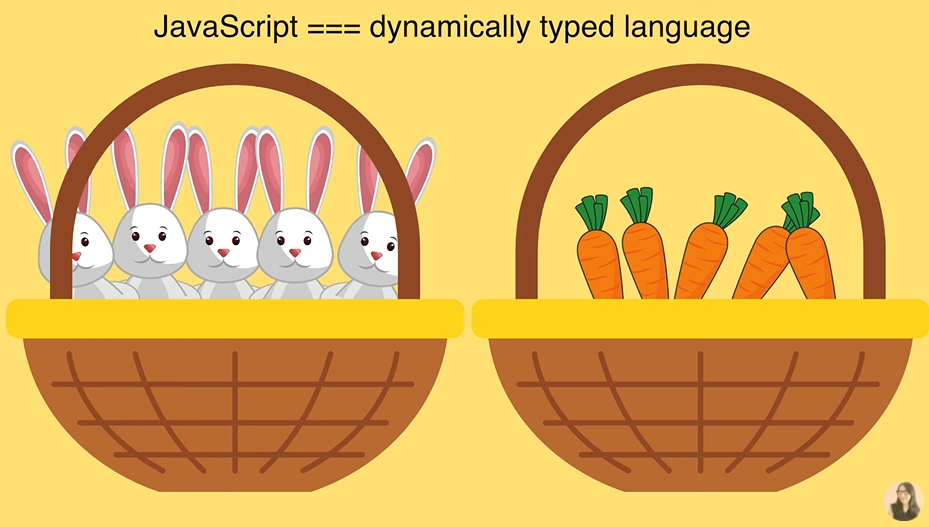
Array
1. Declaration
const arr1 = new Array();
const arr2 = [1, 2];
2. Index position
const fruits = ['apple', 'banana'];
console.log(fruits);
console.log(fruits.length);
console.log(fruits[0]);
console.log(fruits[1]);
console.log(fruits[2]); // undefined
console.log(fruits[fruits.length-1]);
- index를 통해 배열의 값에 접근할 수 있다.
- index는 0부터 시작
3. looping over an array
// a. for
console.clear()
for(let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
// b. for of
for(let fruit of fruits){
console.log(fruits);
}
// c. forEach
fruits.forEach((fruit) => console.log(fruit))
- a는 기본적인 for문 이요
- b는 for of를 이용
- c는 forEach를 이용
- 개인적으로 forEach가 가장 이쁘다.
4. addition, deletion, copy
// push: add an item to the end
fruits.push('strawberry', 'peach');
console.log(fruits);
// pop: remove an item from the end
fruits.pop();
fruits.pop();
console.log(fruits);
- 기본적인 뒤에 넣고 빼기
push, pop
// unshift: add an item to the beginning
fruits.unshift('strawberry', 'peach');
console.log(fruits);
// remove an item from the beginnig
fruits.shift();
fruits.shift();
console.log(fruits);
// note!! shift, unshift are slower than pop, push(정말 정말 어마머아하게)
- 앞에 넣고 빼기
unshift, shift
- 배열의 길이가 길면 길수록 당겨오는 과정이 많아진다.
- 중간에 데이터가 움직여야 한다면 느리다.
// splice: remove an item by index position
fruits.push('strawberry', 'peach', 'lemon');
console.log(fruits)
fruits.splice(1, 1)
// fruits.splice(1);// 몇개 지울지 지정하지 않으면 모두 지워버림
console.log(fruits)
fruits.splice(1, 1, 'apple', 'watermelon')
console.log(fruits)
splice
를 통해 중간에서 삭제 가능
- 그외 여러가지 활용 방안이 있음으로 찾아볼 것
// combine two arrays
const fruits2 = ['stone', 'dog'];
const newfruits = fruits.concat(fruits2);
console.log(newfruits);
concat
두 배열을 합체
- 역시 그외 활용방안에 대해 찾아볼 것
5. Searching
// indexOf: find the index
console.clear()
console.log(fruits);
console.log(fruits.indexOf('apple'));
// includes
console.log(fruits.includes('apple'));
console.log(fruits.includes('coconut'));
// lastIndexOf
console.clear();
console.log(fruits);
console.log(fruits.indexOf('apple'))
console.log(fruits.lastIndexOf('apple'))
indexOf, lastIndexOf, includes
index
는 앞에서부터 있으면 해당 인덱스를 없으면 -1
를 리턴
lastIndexOf
는 뒤에서부터 있으면 해당 인덱스를 없으면 -1
를 리턴
includes
는 있으면 true, 없으면 false를 리턴