You are given two non-empty linked lists representing two non-negative integers. The digits are stored in reverse order and each of their nodes contain a single digit. Add the two numbers and return it as a linked list.
You may assume the two numbers do not contain any leading zero, except the number 0 itself.
두개의 비지 않은 링크드 리스트. 각각은 음수가 아닌 정수로 됨. 수는 거꾸로 저장되있다. 각 노드는 1개의 수. 각 노드의 두개의 수를 더한 링크드 리스트를 리턴.
첫 번째 노드 부터 더해 올림이 발생하는지 체크. 올림이 발생하면 그 다음 노드에 1을 더함.
코드를 입력하세# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def addTwoNumbers(self, l1: ListNode, l2: ListNode) -> ListNode:
isUp = False
ret = ListNode()
cur = ret
while True:
v = 0
if l1 != None and l2 != None:
v = l1.val+l2.val
elif l1 != None:
v = l1.val
else :
v = l2.val
if isUp:
v = v + 1
#condition
if v > 9:
isUp = True
else:
isUp = False
cur.val = v % 10
if (l1 != None and l1.next != None) or (l2 != None and l2.next != None):
cur.next = ListNode()
cur = cur.next
if l1 != None and l1.next != None:
l1 = l1.next
else:
l1 = None
if l2 != None and l2.next != None:
l2 = l2.next
else:
l2 = None
else:
break
#last
if isUp:
cur.next = ListNode(1)
return ret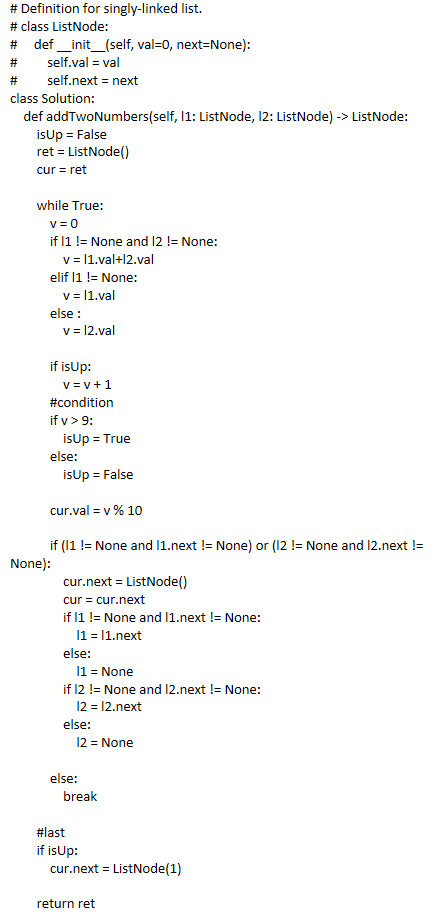요