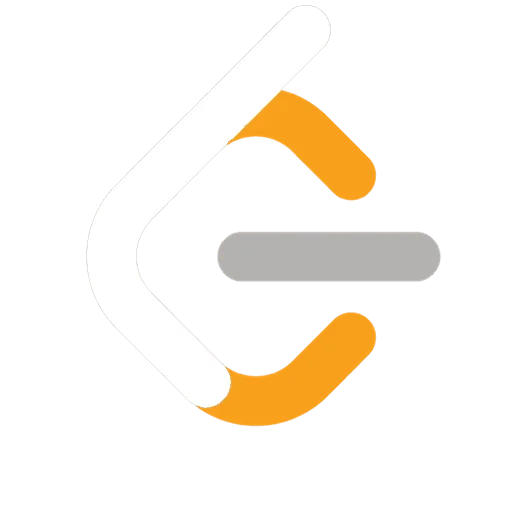
😎풀이
- 최소한의 체력 요구량을 미리 알아야 하므로 공주 위치에서 용사 위치로 이동
- 각 이동의 계산 시 최소 1의 체력은 유지되어야 함
- 용사 위치에 해당하는 값 반환
function calculateMinimumHP(dungeon: number[][]): number {
const maxRow = dungeon.length - 1
const maxCol = dungeon[0].length - 1
const dp = Array.from({length: maxRow + 1}, () => Array(maxCol + 1).fill(0))
for(let row = maxRow; row >= 0; row--) {
for(let col = maxCol; col >= 0; col--) {
const curDamage = dungeon[row][col]
if(row === maxRow && col === maxCol) {
dp[row][col] = Math.max(1, 1 - curDamage)
continue
}
if(row === maxRow) {
dp[row][col] = Math.max(1, dp[row][col + 1] - curDamage)
continue
}
if(col === maxCol) {
dp[row][col] = Math.max(1, dp[row + 1][col] - curDamage)
continue
}
const minHP = Math.min(dp[row + 1][col], dp[row][col + 1])
dp[row][col] = Math.max(1, minHP - curDamage)
}
}
return dp[0][0]
};